I need line by line explanation (using comments in the code itself) of the below Java program. I want to know what each line of code is doing / its function / purpose. Please and thank you Java program source code: class LowBalanceException extends Exception { public LowBalanceException(String message) { super(message); } } class BankAccount { private double balance; private static final double MIN_BALANCE = 50.0; public BankAccount(double initialBalance) { balance = initialBalance; } public void withdraw(double amount) throws LowBalanceException { if (balance - amount < MIN_BALANCE) { throw new LowBalanceException("Insufficient funds! Your balance cannot go below $" + MIN_BALANCE); } balance -= amount; System.out.println("Withdrawal successful. Current balance: $" + balance); } } public class Main { public static void main(String[] args) { BankAccount account = new BankAccount(100.0); try { account.withdraw(70.0); // Withdraw an amount that will result in a LowBalanceException } catch (LowBalanceException e) { System.out.println("Error: " + e.getMessage()); } } }
I need line by line explanation (using comments in the code itself) of the below Java
Java program source code:
class LowBalanceException extends Exception {
public LowBalanceException(String message) {
super(message);
}
}
class BankAccount {
private double balance;
private static final double MIN_BALANCE = 50.0;
public BankAccount(double initialBalance) {
balance = initialBalance;
}
public void withdraw(double amount) throws LowBalanceException {
if (balance - amount < MIN_BALANCE) {
throw new LowBalanceException("Insufficient funds! Your balance cannot go below $" + MIN_BALANCE);
}
balance -= amount;
System.out.println("Withdrawal successful. Current balance: $" + balance);
}
}
public class Main {
public static void main(String[] args) {
BankAccount account = new BankAccount(100.0);
try {
account.withdraw(70.0); // Withdraw an amount that will result in a LowBalanceException
} catch (LowBalanceException e) {
System.out.println("Error: " + e.getMessage());
}
}
}

Step by step
Solved in 3 steps

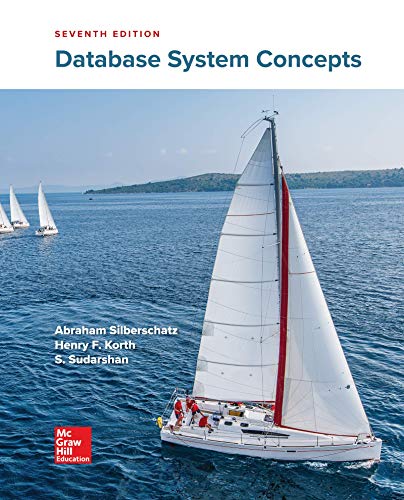
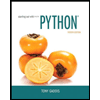
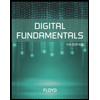
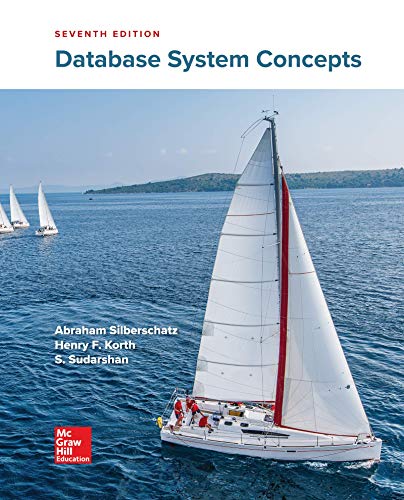
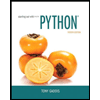
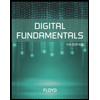
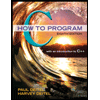
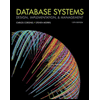
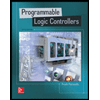