I need help write this java code, described in the image below public class Person { private int ageYears; private String lastName; public void setName(String userName) { lastName = userName; } public void setAge(int numYears) { ageYears = numYears; } // Other parts omitted public void printAll()
I need help write this java code, described in the image below
public class Person {
private int ageYears;
private String lastName;
public void setName(String userName) {
lastName = userName;
}
public void setAge(int numYears) {
ageYears = numYears;
}
// Other parts omitted
public void printAll() {
System.out.print("Name: " + lastName);
System.out.print(", Age: " + ageYears);
}
}
// ===== end =====
// ===== Code from file Student.java =====
public class Student extends Person {
private int idNum;
public void setID(int studentId) {
idNum = studentId;
}
public int getID() {
return idNum;
}
}
// ===== end =====
// ===== Code from file StudentDerivationFromPerson.java =====
public class StudentDerivationFromPerson {
public static void main(String[] args) {
Student courseStudent = new Student();
/* Your solution goes here */
}
}
// ===== end =====
![Assign courseStudent's name with Smith, age with 20, and ID with 9999. Use the printAll() member method and a separate
println() statement to output courseStudents's data. Sample output from the given program:
Name: Smith, Age: 20, ID: 9999
Learn how our autograder works
463796.2927918.gazy7
s
£$£££ wwwwwwwwwww
29
30
31 public int getID() {
return idNum;
32
33 }
34 }
35 // ===== end =====
36
37 // ===== Code from file student Derivation FromPerson.java
38 public class StudentDerivationFromPerson {
39 public static void main(String[] args) {
student coursestudent = new Student ();
/* Your solution goes here */
40
41
42
43
}
44
45 }
46 // ===== end =====
=====
▶](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F7fca094e-0996-4319-a931-2c4dfdbd252f%2Fgc6m2yc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

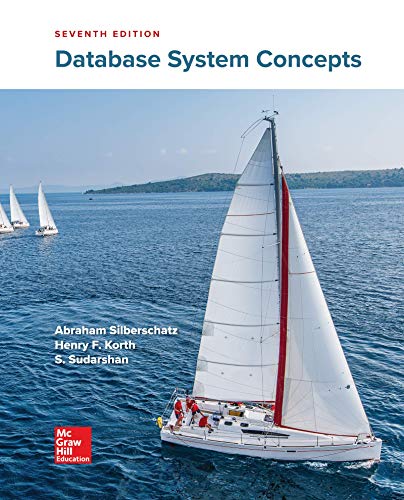
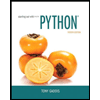
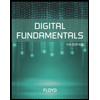
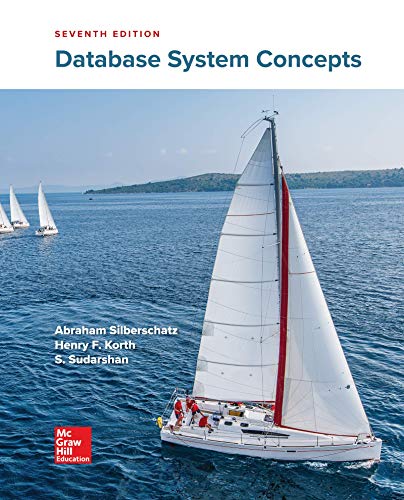
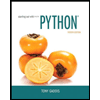
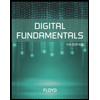
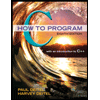
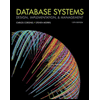
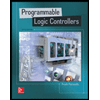