I need help with this problem, and an explanation for the solution is described below (Fundamentals of Computer Engineering: ModelSim - standard edition). I need help modifying the below codes of "VHDL so that the counter counts up from 1 to 7" of both files into "VHDL to design a counter to count up from 1 to 6". (Fundamentals of Computer Engineering: ModelSim - standard edition). Counter_1_to_7.vhdl: library IEEE; use IEEE.STD_LOGIC_1164.ALL; use IEEE.STD_LOGIC_ARITH.ALL; use IEEE.STD_LOGIC_UNSIGNED.ALL; entity Counter_1_to_7 is Port ( clk : in STD_LOGIC; -- Clock input reset : in STD_LOGIC; -- Asynchronous reset count : out STD_LOGIC_VECTOR (2 downto 0) -- 3-bit output (1 to 7) ); end Counter_1_to_7; architecture Behavioral of Counter_1_to_7 is signal counter_reg : STD_LOGIC_VECTOR (2 downto 0) := "001"; -- Start at 1 begin process(clk, reset) begin if reset = '1' then counter_reg <= "001"; -- Reset to 1 elsif rising_edge(clk) then if counter_reg = "111" then counter_reg <= "001"; -- Wrap around to 1 after 7 else counter_reg <= counter_reg + 1; -- Increment counter end if; end if; end process; count <= counter_reg; -- Assign internal counter to output end Behavioral; Counter_1_to_7_tb.vhdl: library IEEE; use IEEE.STD_LOGIC_1164.ALL; entity Counter_1_to_7_tb is end Counter_1_to_7_tb; architecture Behavioral of Counter_1_to_7_tb is -- Component declaration for the Unit Under Test (UUT) component Counter_1_to_7 is Port ( clk : in STD_LOGIC; reset : in STD_LOGIC; count : out STD_LOGIC_VECTOR (2 downto 0) ); end component; -- Testbench signals signal clk : STD_LOGIC := '0'; signal reset : STD_LOGIC := '0'; signal count : STD_LOGIC_VECTOR (2 downto 0); -- Clock period constant clk_period : time := 10 ns; begin -- Instantiate the Unit Under Test (UUT) uut: Counter_1_to_7 Port map ( clk => clk, reset => reset, count => count ); -- Clock process clk_process: process begin while True loop clk <= '0'; wait for clk_period / 2; clk <= '1'; wait for clk_period / 2; end loop; end process; -- Test process stim_proc: process begin -- Test case 1: Reset the counter reset <= '1'; wait for clk_period * 2; reset <= '0'; wait for clk_period * 8; -- Test case 2: Let the counter run wait for clk_period * 10; -- Test case 3: Assert reset mid-operation reset <= '1'; wait for clk_period * 2; reset <= '0'; wait for clk_period * 10; -- End simulation wait for clk_period * 10; assert false report "End of simulation" severity note; end process; end Behavioral;
I need help with this problem, and an explanation for the solution is described below (Fundamentals of Computer Engineering: ModelSim - standard edition). I need help modifying the below codes of "VHDL so that the counter counts up from 1 to 7" of both files into "VHDL to design a counter to count up from 1 to 6". (Fundamentals of Computer Engineering: ModelSim - standard edition).
Counter_1_to_7.vhdl:
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity Counter_1_to_7 is
Port (
clk : in STD_LOGIC; -- Clock input
reset : in STD_LOGIC; -- Asynchronous reset
count : out STD_LOGIC_VECTOR (2 downto 0) -- 3-bit output (1 to 7)
);
end Counter_1_to_7;
architecture Behavioral of Counter_1_to_7 is
signal counter_reg : STD_LOGIC_VECTOR (2 downto 0) := "001"; -- Start at 1
begin
process(clk, reset)
begin
if reset = '1' then
counter_reg <= "001"; -- Reset to 1
elsif rising_edge(clk) then
if counter_reg = "111" then
counter_reg <= "001"; -- Wrap around to 1 after 7
else
counter_reg <= counter_reg + 1; -- Increment counter
end if;
end if;
end process;
count <= counter_reg; -- Assign internal counter to output
end Behavioral;
Counter_1_to_7_tb.vhdl:
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity Counter_1_to_7_tb is
end Counter_1_to_7_tb;
architecture Behavioral of Counter_1_to_7_tb is
-- Component declaration for the Unit Under Test (UUT)
component Counter_1_to_7 is
Port (
clk : in STD_LOGIC;
reset : in STD_LOGIC;
count : out STD_LOGIC_VECTOR (2 downto 0)
);
end component;
-- Testbench signals
signal clk : STD_LOGIC := '0';
signal reset : STD_LOGIC := '0';
signal count : STD_LOGIC_VECTOR (2 downto 0);
-- Clock period
constant clk_period : time := 10 ns;
begin
-- Instantiate the Unit Under Test (UUT)
uut: Counter_1_to_7
Port map (
clk => clk,
reset => reset,
count => count
);
-- Clock process
clk_process: process
begin
while True loop
clk <= '0';
wait for clk_period / 2;
clk <= '1';
wait for clk_period / 2;
end loop;
end process;
-- Test process
stim_proc: process
begin
-- Test case 1: Reset the counter
reset <= '1';
wait for clk_period * 2;
reset <= '0';
wait for clk_period * 8;
-- Test case 2: Let the counter run
wait for clk_period * 10;
-- Test case 3: Assert reset mid-operation
reset <= '1';
wait for clk_period * 2;
reset <= '0';
wait for clk_period * 10;
-- End simulation
wait for clk_period * 10;
assert false report "End of simulation" severity note;
end process;
end Behavioral;

Step by step
Solved in 2 steps

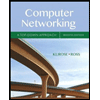
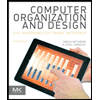
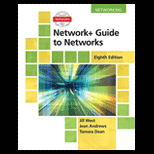
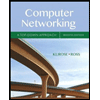
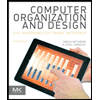
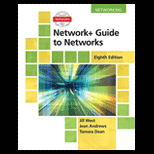
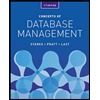
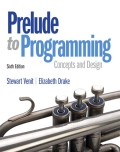
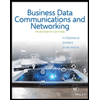