I need help with the part 3 of debugging and correction of program part so it can run properly. My debugger is not giving me the exact result as asked in the manual, so please help with that and correct the program so it run and display result as below. (Following algoritham 1 in manual) Program I wrote following the manual and ran debugger on:- package homework; import java.util.Scanner; public class Lab4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); //Display result to input integer System.out.print("Enter value for a: "); int a = input.nextInt(); System.out.print("Enter value for b: "); int b = input.nextInt(); System.out.print("Enter value for c: "); int c = input.nextInt(); System.out.print("Enter value for d: "); int d = input.nextInt(); //Display result of answers System.out.println(“The larger of a and b is ” + larger); int larger = max(a,b); System.out.println(“The largest of a, b, and c is ” + largestOfThree); int largestOfThree = max(a,b,c); System.out.println(“The largest of a, b, c, and d is ” + largestOfFour); int largestOfFour = max(a,b,c,d); } } } Display Result :- Enter value for a: 4 Enter value for b: -9 Enter value for c: 7 Enter value for d: 10 The larger of a and b is 4 The largest of a, b, and c is 7 The largest of a, b, c, and d is 10
I need help with the part 3 of debugging and correction of program part so it can run properly. My debugger is not giving me the exact result as asked in the manual, so please help with that and correct the program so it run and display result as below. (Following algoritham 1 in manual)
Program I wrote following the manual and ran debugger on:-
package homework;
import java.util.Scanner;
public class Lab4 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
//Display result to input integer
System.out.print("Enter value for a: ");
int a = input.nextInt();
System.out.print("Enter value for b: ");
int b = input.nextInt();
System.out.print("Enter value for c: ");
int c = input.nextInt();
System.out.print("Enter value for d: ");
int d = input.nextInt();
//Display result of answers
System.out.println(“The larger of a and b is ” + larger);
int larger = max(a,b);
System.out.println(“The largest of a, b, and c is ” + largestOfThree);
int largestOfThree = max(a,b,c);
System.out.println(“The largest of a, b, c, and d is ” + largestOfFour);
int largestOfFour = max(a,b,c,d);
}
}
}
Display Result :-
Enter value for a: 4
Enter value for b: -9
Enter value for c: 7
Enter value for d: 10
The larger of a and b is 4
The largest of a, b, and c is 7
The largest of a, b, c, and d is 10

![1. Add a third overloading max method
In your class, add a third overloading max method that returns the maximum among four integer numbers
public static int max (int numl, int num2, int num3, int num4)
There exist multiple algorithms to implement this max. Algorithm 1 is to
1) use the first max to find the larger number of num1 and num2,
2) use the first max again to find the larger number of num3 and num4,
3) use the first max again to find the large number of the previous two results.
Algorithm 2 is to
1) use the second max to find the largest number of num1, num2 and num3, 2)
use the first max to find the larger number of the previous result and num4.
Please implement the third max method using one of the above two algorithms.
The "step over" button appears on the "Debug" window pane in the upper left of your screen. It looks like this:
2 The "step into" button appears next to the "step over" button and looks like this:
2. Modify the main method.
Modify your main method as follows:
public static void main (String[] args) {
System.out.print("Enter value for a: ");
// Add your own statement to get user input for
a
System.out.print("Enter value for b: ");
// Add your own statement to get user input
for b
System.out.print("Enter value for c: ");
// Add your own statement to get user input for
C
System.out.print("Enter value for d: ");
// Add your own statement to get user input for
d
int larger = max (a,b);
System.out.println("The larger of a and b is " + larger);
int largestOfThree = max (a, b, c);
System.out.println("The largest of a, b, and c is " + largestOfThree);
int largestofFour = max (a,b,c,d);
System.out.println("The largest of a, b, c, and d is " + largestOfFour);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2F67f3b3fd-d689-4d80-8ecd-44bd53425f79%2F1e75iw_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

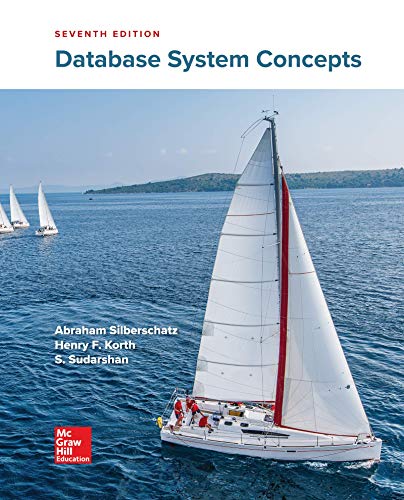
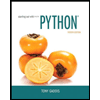
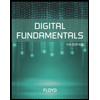
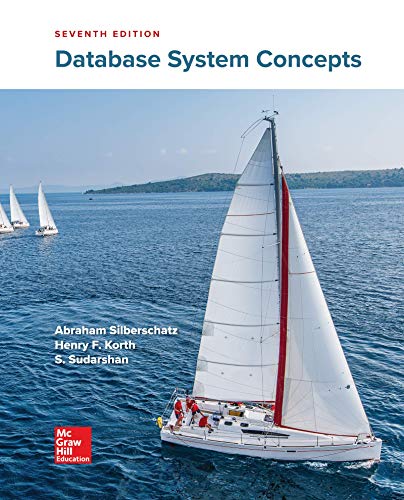
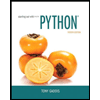
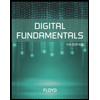
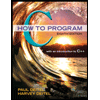
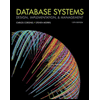
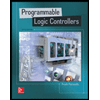