I need help in constructing the function. Following are the given struct that can't be changed // name structure data type struct nameTag { String last; String first; }; typedef struct nameTag nameType; // vaccinee structure data type struct vaccineeTag { int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input) nameType name; // name of vacinee int age; // age of vacinee int bool_frontliner; // 0 means not frontliner, 1 means frontliner int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity int priority; // 0 means priority not yet set, 1 to 4 means priority is set // where 1 is the highest priority and 4 is the lowest priority }; typedef struct vaccineeTag vaccineeType; TO DO #1: Implement Search_by_Name() ( Implement a linear search algorithm to search the VACCINEE[] list if there is a matching element corresponding to the name_keyparameter. If there is a match, return the corresponding array index where it was found in the list. Otherwise, return a value of -1 toindicate that it was not found.) @param: name_key is the search key (containing the last name and first name of the person to be searched) @param: VACCINEE[] is the array of structures (containing info about people who will be scheduled for vaccination) @param: n is the actual number of elements in VACCINEE[] where n <= MAXSIZE. returns: if name_key is found, return the index where name_key was found in VACCINEE[]; otherwise, return a value of -1. */ int Search_by_Name(nameType name_key, vaccineeType VACCINEE[], int n) { /* for Declare your own local variables. Do NOT call printf() or scanf() in this function. */ }
I need help in constructing the function.
Following are the given struct that can't be changed
// name structure data type
struct nameTag {
String last;
String first;
};
typedef struct nameTag nameType;
// vaccinee structure data type
struct vaccineeTag {
int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input)
nameType name; // name of vacinee
int age; // age of vacinee
int bool_frontliner; // 0 means not frontliner, 1 means frontliner
int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity
int priority; // 0 means priority not yet set, 1 to 4 means priority is set
// where 1 is the highest priority and 4 is the lowest priority
};
typedef struct vaccineeTag vaccineeType;
TO DO #1: Implement Search_by_Name() ( Implement a linear search
@param: name_key is the search key (containing the last name and first name of the person to be searched)
@param: VACCINEE[] is the array of structures (containing info about people who will be scheduled for vaccination)
@param: n is the actual number of elements in VACCINEE[] where n <= MAXSIZE.
returns: if name_key is found, return the index where name_key was found in VACCINEE[];
otherwise, return a value of -1.
*/
int Search_by_Name(nameType name_key, vaccineeType VACCINEE[], int n)
{
/* for
Declare your own local variables.
Do NOT call printf() or scanf() in this function.
*/
}

Step by step
Solved in 2 steps with 1 images

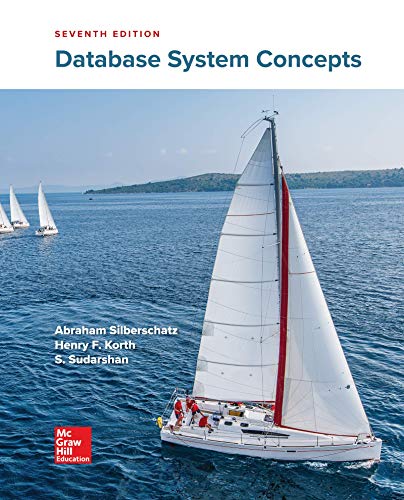
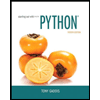
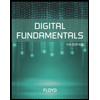
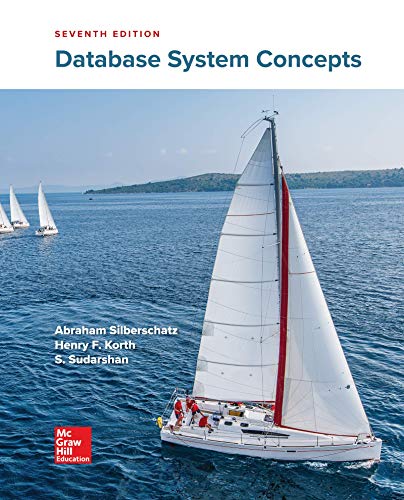
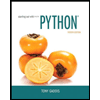
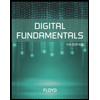
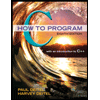
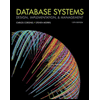
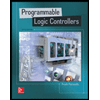