I need help creating the printAnimal() method that prints from the menu, I keep getting errors and I cannot figure out what I am doing wrong. I have included pictures of the first part of the menu loop and the print menu implement a printAnimals() method that provides easy-to-read output displaying the details of objects in an ArrayList. To demonstrate this criterion in a “proficient” way, your implemented method must successfully print the ArrayList of dogs or the ArrayList of monkeys. To demonstrate this criterion in an “exemplary” way, your implemented method must successfully print a list of all animals that are “in service” and “available”. This is what I have so far for the method: // Complete printAnimals // Include the animal name, status, acquisition country and if the animal is reserved. // Remember that this method connects to three different menu items. // The printAnimals() method has three different outputs // based on the listType parameter // dog - prints the list of dogs // monkey - prints the list of monkeys // available - prints a combined list of all animals that are // fully trained ("in service") but not reserved // Remember that you only have to fully implement ONE of these lists. // The other lists can have a print statement saying "This option needs to be implemented". // To score "exemplary" you must correctly implement the "available" list. public static void printAnimals(Scanner scnr) { if (scnr == "4") { System.out.println(dogList); } else if(scnr == '5') { System.out.println(monkeyList); } else if (scnr == '6') { //iterates through dog list for (int i = 0; i < dogList.size(); i++){ if (dogList.get(i).getTrainingStatus().equals("in service") && dogList.get(i).getReserved() == false) { System.out.println(dogList.get(i)); } } for(int i = 0; i < monkeyList.size(); i++) { if (monkeyList.get(i).getTrainingStatus().equals("in service") && monkeyList.get(i).getReserved() == false) { System.out.println(monkeyList.get(i)); } } } } }
I need help creating the printAnimal() method that prints from the menu, I keep getting errors and I cannot figure out what I am doing wrong. I have included pictures of the first part of the menu loop and the print menu
implement a printAnimals() method that provides easy-to-read output displaying the details of objects in an ArrayList.
- To demonstrate this criterion in a “proficient” way, your implemented method must successfully print the ArrayList of dogs or the ArrayList of monkeys.
- To demonstrate this criterion in an “exemplary” way, your implemented method must successfully print a list of all animals that are “in service” and “available”.
This is what I have so far for the method:
// Complete printAnimals
// Include the animal name, status, acquisition country and if the animal is reserved.
// Remember that this method connects to three different menu items.
// The printAnimals() method has three different outputs
// based on the listType parameter
// dog - prints the list of dogs
// monkey - prints the list of monkeys
// available - prints a combined list of all animals that are
// fully trained ("in service") but not reserved
// Remember that you only have to fully implement ONE of these lists.
// The other lists can have a print statement saying "This option needs to be implemented".
// To score "exemplary" you must correctly implement the "available" list.
public static void printAnimals(Scanner scnr) {
if (scnr == "4") {
System.out.println(dogList);
}
else if(scnr == '5') {
System.out.println(monkeyList);
}
else if (scnr == '6') {
//iterates through dog list
for (int i = 0; i < dogList.size(); i++){
if (dogList.get(i).getTrainingStatus().equals("in service") && dogList.get(i).getReserved() == false) {
System.out.println(dogList.get(i));
}
}
for(int i = 0; i < monkeyList.size(); i++) {
if (monkeyList.get(i).getTrainingStatus().equals("in service") && monkeyList.get(i).getReserved() == false) {
System.out.println(monkeyList.get(i));
}
}
}
}
}
![11 public class Driver {
12
private static ArrayList<Dog> dogList = new ArrayList<Dog> ();
13
14
//Monkey Array
15
private static ArrayList<Monkey> monkeyList = new ArrayList<Monkey> ();
170
public static void main (String [] args) {
18
initializeDoglist ();
initializeMonkeyList ();
19
20
21
//loop that displays menu and takes to correct menu option
Scanner scnr = new Scanner (System.in);
displayMenu ();
22
23
24
char input = scnr.nextLine ().charAt (0) ;
25
if(input == 'q') (
26
System.exit(0);
27
28
int inputl = Character.getNumericValue (input);
29
while (inputl < 1 || inputl > €) {
System.out.println ("Invalid selection, please select from the menu"); //input validation
displayMenu ();
input = senr.nextLine ().charAt (0);
inputl = Character.getNumericValue (input);
30
31
32
33
34
35
switch (inputl) {//Takes menu selection to appropriate area
36
case l:
37
intakeNevDog (scnr);
38
break;
39
40
case 2:
41
intakeNewMonkey(senr);
42
break;
43
44
case 3:
45
reserveAnimal (senr);
break;
47
48
саве 4:
49
printAnimals (scnr);
50
break;
51
52
case 5:
53
printAnimals(senr);
54
break;
55
56
case 6:
57
printAnimals(scnr);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9fa295a-90dd-4ec6-950d-22c80fce920e%2Facf2fdf0-45e2-4822-b764-29fee84a4e6c%2Fp7uy9e7_processed.png&w=3840&q=75)
![printAnimals(scnr);
}
// This method prints the menu options
public static void displayMenu () {
System.out.println ("\n\n");
System.out.println ("\t\t\t\tRescue Animal System Menu");
System.out.println ("[1] Intake a new dog");
System.out.println ("[2] Intake a new monkey") ;
System.out.println ("[3] Reserve an animal");
System.out.println (" [4] Print a list of all dogs");
System.out.println (" [5] Print a list of all monkeys");
System.out.println (" [6] Print a list of all animals that are not reserved");
System. out.println ("[q] Quit application");
System.out.println();
System.out.println ("Enter a menu selection");
// Adds dogs to a list for testing
public static void initializeDogList () {
Dog dogl = new Dog ("Spot", "German Shepherd", "male", "1", "25.€", "05-12-2019", "United States", "intake", false, "United States");
Dog dog2 = new Dog ("Rex", "Great Dane", "male", "3", "35.2", "02-03-2020", "United States", "Phase I", false, "United States");
Dog dog3 = new Dog ("Bella", "Chihuahua", "female", "4", "25.6", "12-12-2019", "Canada", "in service", true, "Canada");
doglist.add (dogl);
doglist.add (dog2);
doglist.add (dog3);
}
// Adds monkeys to a list for testing
public static void initializeMonkeyList () {
Monkey monkeyl = new Monkey ("Marcel", "Capuchin", "male", "2", "15.9", "36.5", "24.75", "42.5", "04-05-2020", "United States", "intake", false,
Monkey monkey2 = new Monkey ("Hoffman", "Tamarin", "male", "3", "20.25", "24.25", "30.25", " 46.75", "05-27-2019", "United States", "in service", true, "United States");
Monkey monkey3 = new Monkey ("Elsa", "Marmoset", "female", "E", "30.75", "36.25", "41.€", "49.75", "l1-19-2018", "United States", "in service", true, "United States");
"United States");
monkeyList.add (monkeyl);
monkeyList.add (monkey2);
monkeylist.add (monkey3);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9fa295a-90dd-4ec6-950d-22c80fce920e%2Facf2fdf0-45e2-4822-b764-29fee84a4e6c%2Fchlww5d_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

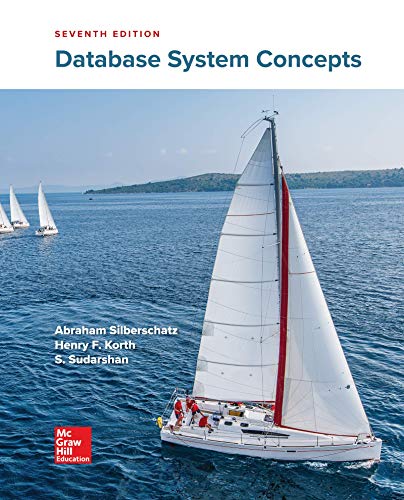
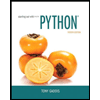
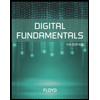
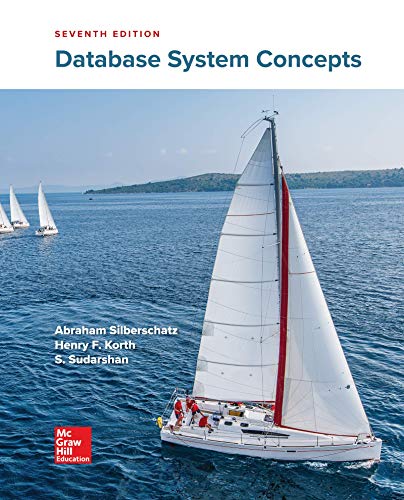
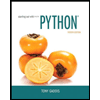
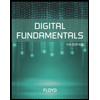
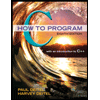
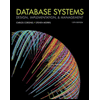
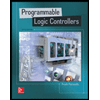