I just need to add a move counter to the following code and make the outout as shown in the attached image. I need to display the number of moves made by the knight and wether or not a full tour of 64moves was completed or not. import java.util.*; public class Main { private final static int base_value = 12; private final static int[][] board_moves = {{1,-2},{2,-1},{2,1},{1,2},{-1,2},{-2,1},{-2,-1},{-1,-2}}; private static int[][] board_grid; private static int total_moves; public static void main(String[] args) { board_grid = new int[base_value][base_value]; total_moves = (base_value - 4) * (base_value - 4); for (int row = 0; row < base_value; row++) for (int col = 0; col < base_value; col++) if (row < 2 || row > base_value - 3 || col < 2 || col > base_value - 3) board_grid[row][col] = -1; int row = 2 + (int) (Math.random() * (base_value - 4)); int col = 2 + (int) (Math.random() * (base_value - 4)); board_grid[row][col] = 1; if (sol(row, col, 2)) display_Result(); else System.out.println("no result"); } private static boolean sol(int row, int col, int move_count) { if (move_count > total_moves) return true; List nrst = nearest(row, col); if (nrst.isEmpty() && move_count != total_moves) return false; Collections.sort(nrst, new Comparator() { public int compare(int[] a_row, int[] b_col) { return a_row[2] - b_col[2]; } }); for (int[] nt : nrst) { row = nt[0]; col = nt[1]; board_grid[row][col] = move_count; if (!orphan_Detected(move_count, row, col) && sol(row, col, move_count + 1)) return true; board_grid[row][col] = 0; } return false; } private static List nearest(int row, int col) { List nrst = new ArrayList<>(); for (int[] move : board_moves) { int row_x = move[0]; int col_y = move[1]; if (board_grid[row + col_y][col + row_x] == 0) { int num = count_nearest(row + col_y, col + row_x); nrst.add(new int[]{row + col_y, col + row_x, num}); } } return nrst; } private static int count_nearest(int row, int col) { int num = 0; for (int[] move : board_moves) if (board_grid[row + move[1]][col + move[0]] == 0) num++; return num; } private static boolean orphan_Detected(int count, int row, int col) { if (count < total_moves - 1) { List nrst = nearest(row, col); for (int[] nt : nrst) if (count_nearest(nt[0], nt[1]) == 0) return true; } return false; } private static void display_Result() { for (int[] row : board_grid) { for (int i : row) { if (i == -1) continue; System.out.printf("%2d ", i); } System.out.println(); } } }
I just need to add a move counter to the following code and make the outout as shown in the attached image. I need to display the number of moves made by the knight and wether or not a full tour of 64moves was completed or not.
import java.util.*;
public class Main {
private final static int base_value = 12;
private final static int[][] board_moves = {{1,-2},{2,-1},{2,1},{1,2},{-1,2},{-2,1},{-2,-1},{-1,-2}};
private static int[][] board_grid;
private static int total_moves;
public static void main(String[] args) {
board_grid = new int[base_value][base_value];
total_moves = (base_value - 4) * (base_value - 4);
for (int row = 0; row < base_value; row++)
for (int col = 0; col < base_value; col++)
if (row < 2 || row > base_value - 3 || col < 2 || col > base_value - 3)
board_grid[row][col] = -1;
int row = 2 + (int) (Math.random() * (base_value - 4));
int col = 2 + (int) (Math.random() * (base_value - 4));
board_grid[row][col] = 1;
if (sol(row, col, 2))
display_Result();
else System.out.println("no result");
}
private static boolean sol(int row, int col, int move_count) {
if (move_count > total_moves)
return true;
List<int[]> nrst = nearest(row, col);
if (nrst.isEmpty() && move_count != total_moves)
return false;
Collections.sort(nrst, new Comparator<int[]>() {
public int compare(int[] a_row, int[] b_col) {
return a_row[2] - b_col[2];
}
});
for (int[] nt : nrst) {
row = nt[0];
col = nt[1];
board_grid[row][col] = move_count;
if (!orphan_Detected(move_count, row, col) && sol(row, col, move_count + 1))
return true;
board_grid[row][col] = 0;
}
return false;
}
private static List<int[]> nearest(int row, int col) {
List<int[]> nrst = new ArrayList<>();
for (int[] move : board_moves) {
int row_x = move[0];
int col_y = move[1];
if (board_grid[row + col_y][col + row_x] == 0) {
int num = count_nearest(row + col_y, col + row_x);
nrst.add(new int[]{row + col_y, col + row_x, num});
}
}
return nrst;
}
private static int count_nearest(int row, int col) {
int num = 0;
for (int[] move : board_moves)
if (board_grid[row + move[1]][col + move[0]] == 0)
num++;
return num;
}
private static boolean orphan_Detected(int count, int row, int col) {
if (count < total_moves - 1) {
List<int[]> nrst = nearest(row, col);
for (int[] nt : nrst)
if (count_nearest(nt[0], nt[1]) == 0)
return true;
}
return false;
}
private static void display_Result() {
for (int[] row : board_grid) {
for (int i : row) {
if (i == -1)
continue;
System.out.printf("%2d ", i);
}
System.out.println();
}
}
}


Step by step
Solved in 2 steps with 1 images

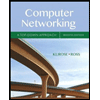
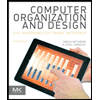
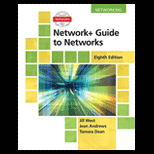
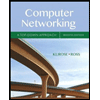
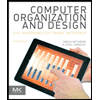
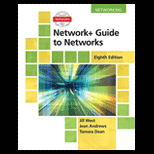
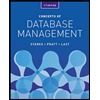
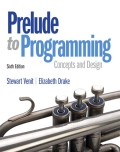
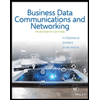