I have to write a java program that will allow me to insert Ravens 13 3 and output "Congratulations, Team Ravens has a winning average!". Also if my input is "Angels 80 82" then my ouput should be "Team Angels has a losing average." I also have 2 java files. (Team.java and WinningTeam.java) Team java looks like public class Team { // TODO: Declare private fields - teamName, teamWins, teamLosses // TODO: Define mutator methods - // setTeamName(), setTeamWins(), setTeamLosses() // TODO: Define accessor methods - // getTeamName(), getTeamWins(), getTeamLosses() // TODO: Define getWinPercentage() } AND WinningTeam.Java looks like import java.util.Scanner; public class WinningTeam { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Team team = new Team(); String name = scnr.next(); int wins = scnr.nextInt(); int losses = scnr.nextInt(); team.setTeamName(name); team.setTeamWins(wins); team.setTeamLosses(losses); if (team.getWinPercentage() >= 0.5) { System.out.println("Congratulations, Team " + team.getTeamName() + " has a winning average!"); } else { System.out.println("Team " + team.getTeamName() + " has a losing average."); } } } WinningTeam.java is correct, that much I do know
I have to write a java program that will allow me to insert Ravens 13 3 and output "Congratulations, Team Ravens has a winning average!". Also if my input is "Angels 80 82" then my ouput should be
// TODO: Declare private fields - teamName, teamWins, teamLosses
// TODO: Define mutator methods -
// setTeamName(), setTeamWins(), setTeamLosses()
// TODO: Define accessor methods -
// getTeamName(), getTeamWins(), getTeamLosses()
// TODO: Define getWinPercentage()
}
import java.util.Scanner;
public class WinningTeam {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Team team = new Team();
String name = scnr.next();
int wins = scnr.nextInt();
int losses = scnr.nextInt();
team.setTeamName(name);
team.setTeamWins(wins);
team.setTeamLosses(losses);
if (team.getWinPercentage() >= 0.5) {
System.out.println("Congratulations, Team " + team.getTeamName() +
" has a winning average!");
}
else {
System.out.println("Team " + team.getTeamName() +
" has a losing average.");
}
}
}
WinningTeam.java is correct, that much I do know

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

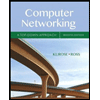
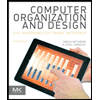
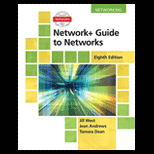
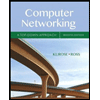
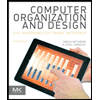
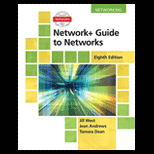
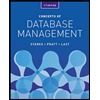
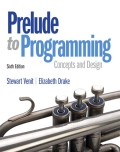
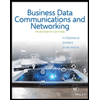