*3.17 (Game: scissor, rock, paper) Write a program that plays the popular scissor-rock-paper game. (A scissor can cut a paper, a rock can knock a scissor, and a paper can wrap a rock.) The program randomly generates a number 0, 1, or 2 representing scissor, rock, and paper. The program prompts the user to enter a number 0, 1, or 2 and displays a message indicating whether the user or the computer wins, loses, or draws.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:


The source code of the program
import java.util.Random;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Random rand = new Random();
Scanner sc = new Scanner(System.in);
int computerChoice = rand.nextInt(3); // 0 = scissors, 1 = rock, 2 = paper
System.out.print("Enter your choice (0 = scissors, 1 = rock, 2 = paper): ");
int userChoice = sc.nextInt();
String computerChoiceStr = "";
switch (computerChoice) {
case 0:
computerChoiceStr = "scissors";
break;
case 1:
computerChoiceStr = "rock";
break;
case 2:
computerChoiceStr = "paper";
break;
}
String userChoiceStr = "";
switch (userChoice) {
case 0:
userChoiceStr = "scissors";
break;
case 1:
userChoiceStr = "rock";
break;
case 2:
userChoiceStr = "paper";
break;
}
if (computerChoice == userChoice) {
System.out.println("Computer chose " + computerChoiceStr
+ ", User chose " + userChoiceStr + ", it's a draw!");
} else if (computerChoice == 0 && userChoice == 2 ||
computerChoice == 1 && userChoice == 0 ||
computerChoice == 2 && userChoice == 1) {
System.out.println("Computer chose " + computerChoiceStr
+ ", User chose " + userChoiceStr + ", computer wins!");
} else {
System.out.println("Computer chose " + computerChoiceStr
+ ", User chose " + userChoiceStr + ", user wins!");
}
}
}
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

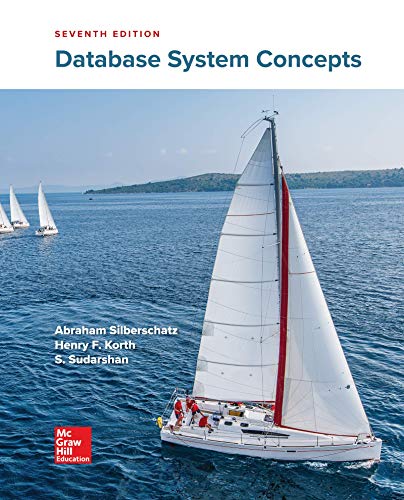
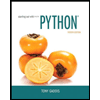
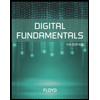
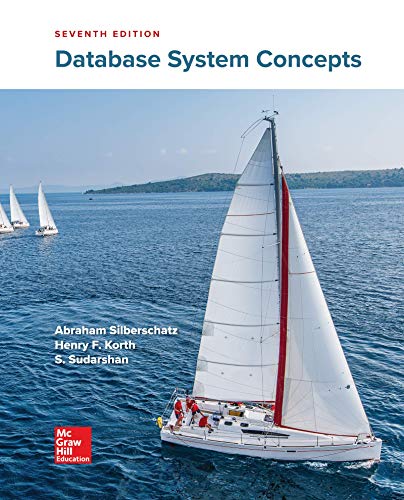
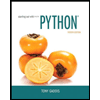
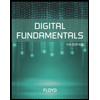
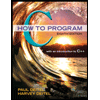
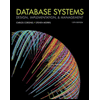
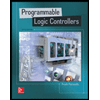