You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment. In this problem, you must implement a tokenizer for the Assembly instruction format: an operation type (e.g. SUBI) followed by a comma-separated list of parameters (e.g. R0, R1, 6). If a line has an invalid syntax, it should be skipped in the output. You do not need to check whether the operations and arguments are valid Assembly instructions: you just need to separate them into tokens. Input Format The input to the program will consist of some number of lines. Each line is of the following form: "op arg1, arg2, ..., argn". Your program should terminate upon receiving a blank line or EOF. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem. Output Format The tokenized output from each input line should be given as a series of space-separated tokens, without commas or other symbols. Invalid lines should be skipped. Input 0 ADD R0, R6, R7 SUB R3, R4, R5 Output 0 ADD R0 R6 R7 SUB R3 R4 R5 Input 1 ADDI R8, R9, 6 JMP R3 Output 1 ADDI R8 R9 6 JMP R3 Input 2 TEST a TEST a, b, c, d, e, f, g Output 2 TEST a TEST a b c d e f g Input 3 this file, tests TEST a, b c erroneous cases TEST, a, b there should, only, be TEST, a b six lines, in, the, output TEST a b and it, should TEST a , b, c include this, one TEST a, b,, c Output 3 this file tests erroneous cases there should only be six lines in the output and it should include this one All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows: TEST a, b c: the comma between b and c is missing TEST, a, b: there is an extra comma after TEST TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST TEST a b: the comma between a and b is missing TEST a , b, c: there is a space before the comma between a and b TEST a, b,, c: there is an extra comma between b and c
You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment.
In this problem, you must implement a tokenizer for the Assembly instruction format: an operation type (e.g. SUBI) followed by a comma-separated list of parameters (e.g. R0, R1, 6). If a line has an invalid syntax, it should be skipped in the output. You do not need to check whether the operations and arguments are valid Assembly instructions: you just need to separate them into tokens. Input Format The input to the program will consist of some number of lines. Each line is of the following form: "op arg1, arg2, ..., argn". Your program should terminate upon receiving a blank line or EOF. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem. Output Format The tokenized output from each input line should be given as a series of space-separated tokens, without commas or other symbols. Invalid lines should be skipped. Input 0 ADD R0, R6, R7 SUB R3, R4, R5 Output 0 ADD R0 R6 R7 SUB R3 R4 R5 Input 1 ADDI R8, R9, 6 JMP R3 Output 1 ADDI R8 R9 6 JMP R3 Input 2 TEST a TEST a, b, c, d, e, f, g Output 2 TEST a TEST a b c d e f g Input 3 this file, tests TEST a, b c erroneous cases TEST, a, b there should, only, be TEST, a b six lines, in, the, output TEST a b and it, should TEST a , b, c include this, one TEST a, b,, c Output 3 this file tests erroneous cases there should only be six lines in the output and it should include this one All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows: TEST a, b c: the comma between b and c is missing TEST, a, b: there is an extra comma after TEST TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST TEST a b: the comma between a and b is missing TEST a , b, c: there is a space before the comma between a and b TEST a, b,, c: there is an extra comma between b and c

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

I have something similar but I having difficulty for it passing the final test case
Sample Input 3
Sample Output 3
Explanation 3
All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows:
- TEST a, b c: the comma between b and c is missing
- TEST, a, b: there is an extra comma after TEST
- TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST
- TEST a b: the comma between a and b is missing
- TEST a , b, c: there is a space before the comma between a and b
- TEST a, b,, c: there is an extra comma between b and c
My current output as of right now
this file tests
TEST a b c
erroneous cases
TEST a b
there should only be
TEST a b
six lines in the output
TEST a b
and it should
TEST a b c
include this one
TEST a b c
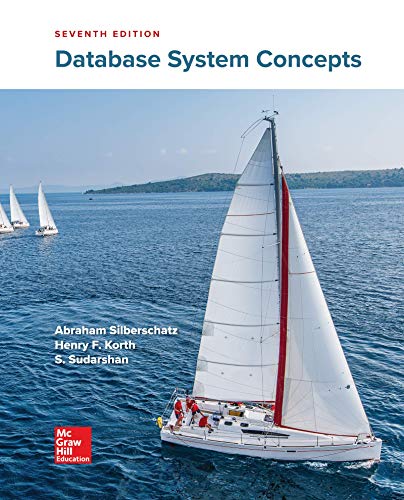
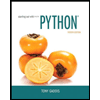
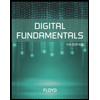
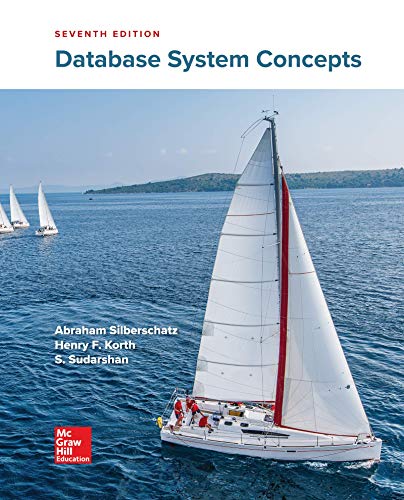
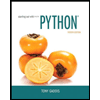
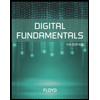
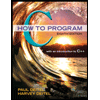
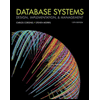
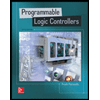