I have a problem with the program below. Line 31 give a warning of 3 dots (…) under the suf word suffix. I have attached a screenshot showing where the problem is and a message box stating what the problem is. If possible, can you fix this program problem please. Thank you Write a program in C++ that reads a sentence as input and converts each word to "Pig Latin." In one version, to convert a word to Pig Latin, your remove the first letter and place the letter at the end of the word. Then you append the string "ay" to the word. Here is an example: English: I SLEPT MOST OF THE NIGHT Pig Latin: IAY LEPTSAY OSTMAY FOAY HETAY IGHTNAY Criteria program compiles 2) program solves problem according to specification 3) program declares, creates, or initializes static or dynamic array correctly 4) if required program defines function or functions with array parameters or array returns 5) program uses arrays to solve problem 6) program destroys any dynamic arrays #include #include #include using namespace std; string piglatin(string); string substr(string, int&); int main() { string input; cout << "Enter a sentence: "; getline(cin, input); cout << "Piglatin: " << piglatin(input) << endl << endl; return 0; } string piglatin(string input) { int len = 0, counter = 0, start = 0, stop = 0; string word = "", newstring = ""; do { word = substr(input, start); //translate the next word start++; newstring = newstring + word + " "; //add the word and a blank to the new sentence } while (start < input.length()); return newstring; } string substr(string s, int& n) { char word[50] = "", suffix[2]; // has warning, three ... under the suf of the word suffix[2]; int i = 0; suffix[0] = s[n]; //get the first letter suffix[1] = '\0'; n++; while (s[n] != ' ' && s[n] != '\0') //copy letters from the input to the new word until end of the word { word[i] = s[n]; n++; i++; } strcat(word, suffix); //add the suffix created to the new word strcat(word, "ay"); //add "ay" to it return word; }
I have a problem with the program below. Line 31 give a warning of 3 dots (…) under the suf word suffix. I have attached a screenshot showing where the problem is and a message box stating what the problem is. If possible, can you fix this program problem please. Thank you
Write a program in C++ that reads a sentence as input and converts each word to "Pig Latin." In one version, to convert a word to Pig Latin, your remove the first letter and place the letter at the end of the word. Then you append the string "ay" to the word. Here is an example:
English: I SLEPT MOST OF THE NIGHT
Pig Latin: IAY LEPTSAY OSTMAY FOAY HETAY IGHTNAY
Criteria
- program compiles
2) program solves problem according to specification
3) program declares, creates, or initializes static or dynamic array correctly
4) if required program defines function or functions with array parameters or array returns
5) program uses arrays to solve problem
6) program destroys any dynamic arrays
#include<iostream>
#include <cstring>
#include <string>
using namespace std;
string piglatin(string);
string substr(string, int&);
int main()
{
string input;
cout << "Enter a sentence: ";
getline(cin, input);
cout << "Piglatin: " << piglatin(input) << endl << endl;
return 0;
}
string piglatin(string input)
{
int len = 0, counter = 0, start = 0, stop = 0;
string word = "", newstring = "";
do
{
word = substr(input, start); //translate the next word
start++;
newstring = newstring + word + " "; //add the word and a blank to the new sentence
} while (start < input.length());
return newstring;
}
string substr(string s, int& n)
{
char word[50] = "", suffix[2]; // has warning, three ... under the suf of the word suffix[2];
int i = 0;
suffix[0] = s[n]; //get the first letter
suffix[1] = '\0';
n++;
while (s[n] != ' ' && s[n] != '\0') //copy letters from the input to the new word until end of the word
{
word[i] = s[n];
n++;
i++;
}
strcat(word, suffix); //add the suffix created to the new word
strcat(word, "ay"); //add "ay" to it
return word;
}
![Homework13Problem2
| (Global Scope)
O substr(string s, int & n)
22
23
word- substr(input, start); //translate the next word
24
start++;
newstring - newstring + word + "; //add the word and a blank to the new sentence
} while (start < input.length());
return newstring;
25
26
27
28
Estring substr(string s, intå n)
30
29
char word[58] - "", suffix[2];
int i- e;
suffix[0] = s[n
suffix[1] - "le Add initializer
31
32
33
w fiect latter
34
Int1006: Local variable is not initialiced.
35
nt;
while (s[n] !-
{
word[i]
36
88 s[n] !-
end of the word
37
char word[5e] -
char word[50] -
int i- 0;
suffix[2];
suffix[2):
38
- s[n];
39
n++;
40
i+t;
41
strcat(word, suffix); //add the suffix created to the new word
strcat(word, "ay"); //add "ay" to it
return word;
42
100%
O No issues found
Ln: 31 Ch: 34 SPC
CRLF](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F802555ea-2663-4669-897b-7df9c2cf7457%2F23fdc5bf-ae41-487d-9388-cbcea1114eb6%2Frx7l758_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

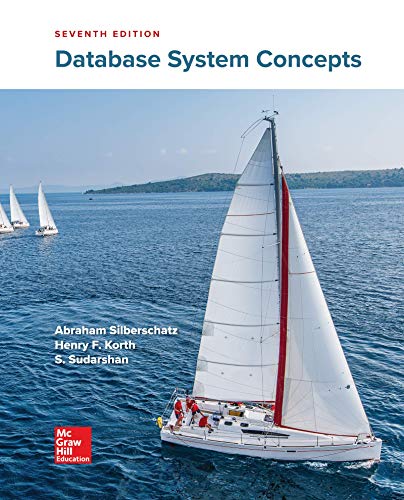
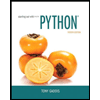
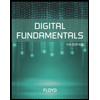
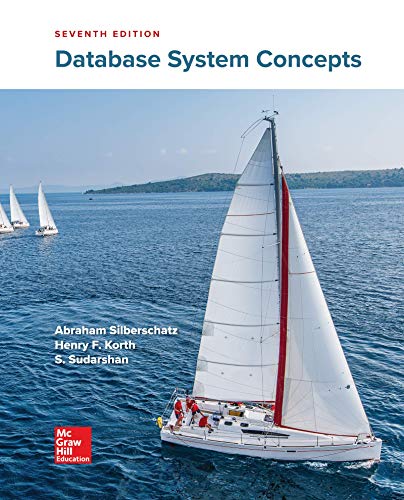
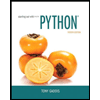
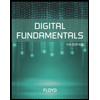
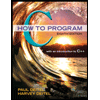
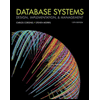
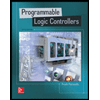