Write the C++ program so that it processes both uppercase and lowercase letters and outputs the corresponding telephone digit. If the input is something other than an uppercase or lowercase letter, the program must output an appropriate error message. Hint: If-else statements and type casting are involved. No switch structure should be involved. Please use the below program to only add not delete the program. //User enters a letter (capital or lowercase) //Program should write out whether that letter is //a telephone digit or invalid input #include using namespace std; int main() { //Declare variables char letter; int digit; int numc, num1; //Prompt the user and reads in values. cout << "To stop the program press #." << endl; cout << "Enter a letter: "; cin >> letter; while (letter != '#') { cout << "Letter: " << letter << ", Corresponding telephone" << " digit: "; numc = static_cast(letter) - static_cast('A'); num1 = static_cast(letter) - static_cast('a'); if (0 <= numc && numc < 26) { digit = numc / 3 + 2; if ((numc / 3 == 6 || numc / 3 == 7) && (numc % 3 == 0)) digit = digit - 1; if (digit > 9) digit = 9; cout << digit << endl;
//User enters a letter (capital or lowercase)
//Program should write out whether that letter is
//a telephone digit or invalid input
#include <iostream>
using namespace std;
int main()
{
//Declare variables
char letter;
int digit;
int numc, num1;
//Prompt the user and reads in values.
cout << "To stop the program press #." << endl;
cout << "Enter a letter: ";
cin >> letter;
while (letter != '#')
{
cout << "Letter: " << letter << ", Corresponding telephone"
<< " digit: ";
numc = static_cast<int>(letter) - static_cast<int>('A');
num1 = static_cast<int>(letter) - static_cast<int>('a');
if (0 <= numc && numc < 26)
{
digit = numc / 3 + 2;
if ((numc / 3 == 6 || numc / 3 == 7) && (numc % 3 == 0))
digit = digit - 1;
if (digit > 9)
digit = 9;
cout << digit << endl;

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

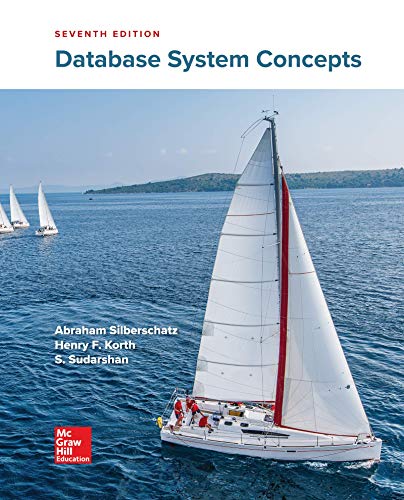
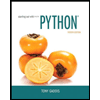
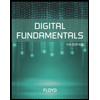
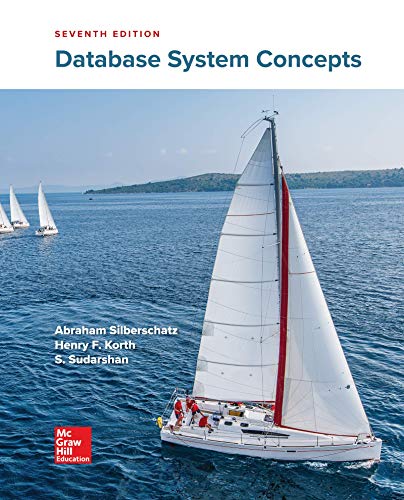
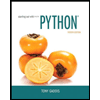
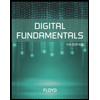
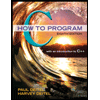
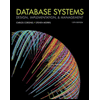
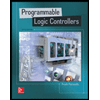