I have a block of Python. How do I modify it to solve this classical mechanics problem (questions are in the picture)? import matplotlib.pyplot as plt import numpy as np import os # This solves for x such that 1+tanh(x)=alpha *x # The program first prompts for alpha print("This find zeros of function 1+tanh(x)-alpha*x") alpha=float(input("Enter alpha: ")) # This defines a function F(x) and its derivative Fprime(x) def GetFFprime(x): F=1.0+np.tanh(x)-alpha*x Fprime=1.0/(np.cosh(x)*np.cosh(x)) -alpha return F,Fprime xguess=float(input("Guess a value of x: ")) yarray=[] # Make arrays with no elements xarray=[] xarray.append(xguess) # Add element with guess for first value yarray.append(0.0) biggestx=xarray[0] offby=1.0E20 # used to check convergence narray=0 while offby>1.0E-7 and narray<10: yarray[narray],yprime=GetFFprime(xarray[narray]) print("x=",xarray[narray]," y=",yarray[narray]) offby=np.fabs(yarray[narray]) xarray.append(0.0) yarray.append(0.0) xarray[narray+1]=xarray[narray]-yarray[narray]/yprime if xarray[narray+1] > biggestx: #This is usedfor plot biggestx=xarray[narray+1] narray+=1 yarray[narray],yprime=GetFFprime(xarray[narray]) print("----------------\n Final: x=",xarray[narray]," y=",yarray[narray]) # Plot the points plt.plot(xarray,yarray,linestyle='None',markersize='5',marker='s',markerfacecolor='none',markeredgecolor='red') # Plot 1+tanh(x), -alpha*x and 1+tanh(x)-alpha*x nplot=50 delx=biggestx/nplot x=np.zeros(nplot+1,dtype='float') y1=np.zeros(nplot+1,dtype='float') y=np.zeros(nplot+1,dtype='float') for ix in range(0,nplot+1): x[ix]=ix*delx y1[ix]=1.0+np.tanh(x[ix]) y[ix]=y1[ix]-alpha*x[ix] plt.plot(x,y1,linestyle='-',lw=1,color='red',marker=None,label="$1+\\tanh(x)$") plt.plot(x,alpha*x,linestyle='-',lw=1,color='green',marker=None,label="$\\alpha x$") plt.plot(x,y,linestyle='-',lw=2,color='blue',marker=None,label="$1+\\tanh(x)-\\alpha x$") # Add some dashed lines for visual hline_y=[0.0,0.0] hline_x=[0.0,nplot*delx] plt.plot(hline_x,hline_y,linestyle='--',lw=1,color='k',marker=None) vline_y=[0.0,alpha*xarray[narray]] vline_x=[xarray[narray],xarray[narray]] plt.plot(vline_x,vline_y,linestyle='--',lw=1,color='k',marker=None) plt.legend() sometext="$\\alpha=$"+str(alpha)+"\n$x=$"+str(xarray[narray]) plt.text(0.0,y[nplot],sometext) plt.xlabel('x') plt.ylabel('$1.0+\\tanh(x)-\\alpha x$') #plt.show() # Or make pdf file plt.savefig('newton_template.pdf',format='pdf') # if you want to look at pdf file os.system("open newton_template.pdf") # This can replace "plt.show()" --works on Mac or Linux quit()
I have a block of Python. How do I modify it to solve this classical
import matplotlib.pyplot as plt
import numpy as np
import os
# This solves for x such that 1+tanh(x)=alpha *x
# The program first prompts for alpha
print("This find zeros of function 1+tanh(x)-alpha*x")
alpha=float(input("Enter alpha: "))
# This defines a function F(x) and its derivative Fprime(x)
def GetFFprime(x):
F=1.0+np.tanh(x)-alpha*x
Fprime=1.0/(np.cosh(x)*np.cosh(x)) -alpha
return F,Fprime
xguess=float(input("Guess a value of x: "))
yarray=[] # Make arrays with no elements
xarray=[]
xarray.append(xguess) # Add element with guess for first value
yarray.append(0.0)
biggestx=xarray[0]
offby=1.0E20 # used to check convergence
narray=0
while offby>1.0E-7 and narray<10:
yarray[narray],yprime=GetFFprime(xarray[narray])
print("x=",xarray[narray]," y=",yarray[narray])
offby=np.fabs(yarray[narray])
xarray.append(0.0)
yarray.append(0.0)
xarray[narray+1]=xarray[narray]-yarray[narray]/yprime
if xarray[narray+1] > biggestx: #This is usedfor plot
biggestx=xarray[narray+1]
narray+=1
yarray[narray],yprime=GetFFprime(xarray[narray])
print("----------------\n Final: x=",xarray[narray]," y=",yarray[narray])
# Plot the points
plt.plot(xarray,yarray,linestyle='None',markersize='5',marker='s',markerfacecolor='none',markeredgecolor='red')
# Plot 1+tanh(x), -alpha*x and 1+tanh(x)-alpha*x
nplot=50
delx=biggestx/nplot
x=np.zeros(nplot+1,dtype='float')
y1=np.zeros(nplot+1,dtype='float')
y=np.zeros(nplot+1,dtype='float')
for ix in range(0,nplot+1):
x[ix]=ix*delx
y1[ix]=1.0+np.tanh(x[ix])
y[ix]=y1[ix]-alpha*x[ix]
plt.plot(x,y1,linestyle='-',lw=1,color='red',marker=None,label="$1+\\tanh(x)$")
plt.plot(x,alpha*x,linestyle='-',lw=1,color='green',marker=None,label="$\\alpha x$")
plt.plot(x,y,linestyle='-',lw=2,color='blue',marker=None,label="$1+\\tanh(x)-\\alpha x$")
# Add some dashed lines for visual
hline_y=[0.0,0.0]
hline_x=[0.0,nplot*delx]
plt.plot(hline_x,hline_y,linestyle='--',lw=1,color='k',marker=None)
vline_y=[0.0,alpha*xarray[narray]]
vline_x=[xarray[narray],xarray[narray]]
plt.plot(vline_x,vline_y,linestyle='--',lw=1,color='k',marker=None)
plt.legend()
sometext="$\\alpha=$"+str(alpha)+"\n$x=$"+str(xarray[narray])
plt.text(0.0,y[nplot],sometext)
plt.xlabel('x')
plt.ylabel('$1.0+\\tanh(x)-\\alpha x$')
#plt.show()
# Or make pdf file
plt.savefig('newton_template.pdf',format='pdf')
# if you want to look at pdf file
os.system("open newton_template.pdf") # This can replace "plt.show()" --works on Mac or Linux
quit()


- Start.
- Import the necessary libraries, including matplotlib and numpy.
- Define a function `x_position` to calculate the horizontal position at time `t` based on the initial velocity `vo`, launch angle `theta`, drag term `drag_term`, and time `t`.
- Define a function `x_position_derivative` to calculate the derivative of the horizontal position with respect to time `t` at a given time `t`.
- Define a function `horizontal_position_error` to calculate the horizontal position error at time `t` by subtracting the desired horizontal position `h` from the calculated horizontal position.
- Prompt the user to input values for initial velocity `vo`, launch angle in degrees `theta_deg`, drag term `drag_term`, and the height of the cannon above the plain `h`.
- Convert the input angle `theta_deg` to radians for calculations.
- Initialize a guess for the time `t_guess` as 0.0.
- Create empty lists `t_values` and `error_values` to store time and error values for plotting.
- Use Newton's method to find the time when the horizontal position is equal to `h` (y = 0). Set a maximum number of iterations (`max_iterations`) and a tolerance value (`tolerance`) for convergence.
- Inside a loop, calculate the current horizontal position error and its derivative at the current `t_guess`.
- Update the `t_guess` using Newton's method: `t_guess -= error / derivative`.
- Check if the absolute value of the error is less than the tolerance. If it is, break out of the loop.
- Calculate the horizontal range of the cannon by calling the `x_position` function with the final `t_guess`.
- Print the time to return to horizontal and the horizontal range of the cannon.
- Plot the error vs. iteration to visualize the convergence of the algorithm.
- Display the plot.
- End.
Step by step
Solved in 4 steps with 2 images

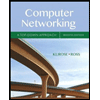
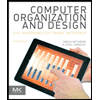
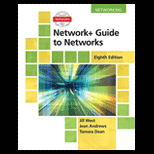
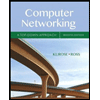
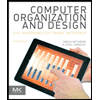
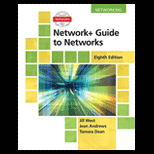
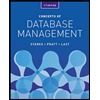
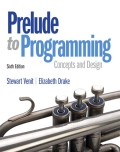
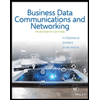