Write a function to calculate the hypotenuse using Pythagorean theorem: = Vla? + b?) To do this you will need to use a function to calculate the square root of a number. We need to import this function from a package called numpy (numerical python), and this packages is imported and given the shortname np by calling import numpy as np. You only need to do this once and the standard convention is to import all the packages we need at the top of file. To calculate the square root of a number, x , you would type, np.sqrt(x) , e.g. np. sqrt(4) would give you 2. Use the cell below to try out numpy's square root function. In [ ]: import numpy as np In [ ]: # use this cell to play around with np.sqrt() Finish the function below so that it calculates the hypotenuse In []: # This function calculates the hypotenuse def calc_hypotenuse(a,b): "uses pythagorus a: first argument b: second argument # YOUR CODE HERE raise NotImplementedError(). return c In [ ]: calc_hypotenuse(3,4) In [ ]: assert calc_hypotenuse(3,4) == 5
Write a function to calculate the hypotenuse using Pythagorean theorem: = Vla? + b?) To do this you will need to use a function to calculate the square root of a number. We need to import this function from a package called numpy (numerical python), and this packages is imported and given the shortname np by calling import numpy as np. You only need to do this once and the standard convention is to import all the packages we need at the top of file. To calculate the square root of a number, x , you would type, np.sqrt(x) , e.g. np. sqrt(4) would give you 2. Use the cell below to try out numpy's square root function. In [ ]: import numpy as np In [ ]: # use this cell to play around with np.sqrt() Finish the function below so that it calculates the hypotenuse In []: # This function calculates the hypotenuse def calc_hypotenuse(a,b): "uses pythagorus a: first argument b: second argument # YOUR CODE HERE raise NotImplementedError(). return c In [ ]: calc_hypotenuse(3,4) In [ ]: assert calc_hypotenuse(3,4) == 5
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![Write a function to calculate the hypotenuse using Pythagorean theorem:
= Va? + b?)
To do this you will need to use a function to calculate the square root of a number. We need to import this function from a package called numpy
(numerical python), and this packages is imported and given the shortname np by calling import numpy as np. You only need to do this once and
the standard convention is to import all the packages we need at the top of file.
To calculate the square root of a number, x , you would type, np.sqrt(x) , e.g. np. sqrt(4) would give you 2. Use the cell below to try out numpy's
square root function.
In [ ]: import numpy as np
In [ ]: # use this cell to play around with np. sqrt()
Finish the function below so that it calculates the hypotenuse
In [ ]:
# This function calculates the hypotenuse
def calc_hypotenuse(a,b):
'uses pythagorus
a: first argument
b: second argument
# YOUR CODE HERE
raise NotImplementedError()
return c
In [ ]: calc_hypotenuse(3,4)
In [ ]: assert calc_hypotenuse(3,4)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F42d27e46-79c1-48af-88ec-d78905728737%2F97d50bfc-9b8a-4455-a6cf-12bf64687293%2F2m2zdej_processed.png&w=3840&q=75)
Transcribed Image Text:Write a function to calculate the hypotenuse using Pythagorean theorem:
= Va? + b?)
To do this you will need to use a function to calculate the square root of a number. We need to import this function from a package called numpy
(numerical python), and this packages is imported and given the shortname np by calling import numpy as np. You only need to do this once and
the standard convention is to import all the packages we need at the top of file.
To calculate the square root of a number, x , you would type, np.sqrt(x) , e.g. np. sqrt(4) would give you 2. Use the cell below to try out numpy's
square root function.
In [ ]: import numpy as np
In [ ]: # use this cell to play around with np. sqrt()
Finish the function below so that it calculates the hypotenuse
In [ ]:
# This function calculates the hypotenuse
def calc_hypotenuse(a,b):
'uses pythagorus
a: first argument
b: second argument
# YOUR CODE HERE
raise NotImplementedError()
return c
In [ ]: calc_hypotenuse(3,4)
In [ ]: assert calc_hypotenuse(3,4)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
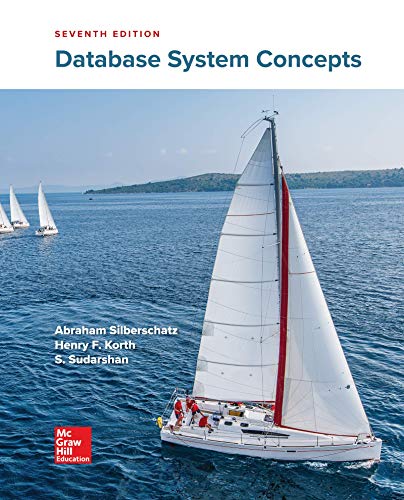
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
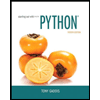
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
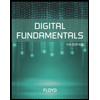
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
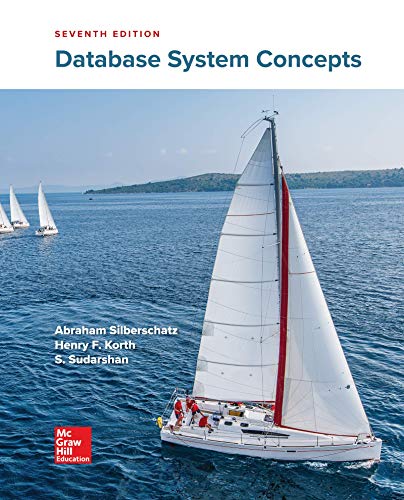
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
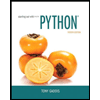
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
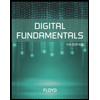
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
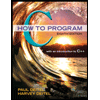
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
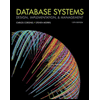
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
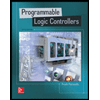
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education