I get an error saying a nonstatic member reference must be relative to a specific object for the float functions. I
I just need help with the 3 functions in the Deck class. I get an error saying a nonstatic member reference must be relative to a specific object for the float functions. I also need help writing the sort function.
#include <iostream>
#include <string>
#include <fstream>
#include <random>
#include <
using namespace std;
/*******************************
* CARD CLASS
*******************************/
class Card {
private:
char Suit, Value;
// Weight Proves that A > K > Q > J > T > 9-2
short Weight;
public:
Card();
Card(char suit, char value);
string GetCard() const;
int GetWeight() const;
char GetSuit() const;
static bool LessThan(Card LHS, Card RHS);
static bool Equal(Card LHS, Card RHS);
Card::Card()
Card::Card(char suit, char value) : Suit(suit), Value(value){
switch(Value);
}
string Card::GetCard() const
int Card::GetWeight() const
char Card::GetSuit() const
bool Card::LessThan(Card LHS, Card RHS) {
if (LHS.Weight == RHS.Weight) {
return LHS.Suit < RHS.Suit;
}
return LHS.Weight < RHS.Weight;
}
bool Card::Equal(Card LHS, Card RHS)
/************************************************
| DECK MEMBER FUNCTION DEFINITONS |
| v v v v v v v v v v v |
*************************************************/
Deck::Deck()
{
cout << "Creating Empty Deck";
}
Deck::Deck(vector<Card> cards) : cards(cards)
{
this->cards = cards;
}
void Deck::AddToDeck(Card card) {
cards.push_back(card);
}
void Deck::ShuffleDeck() {
for (int i = 0; i < 156; i++) {
int a = GetRandomCardIndex();
int b = GetRandomCardIndex();
while(a == b) {
a = GetRandomCardIndex();
b = GetRandomCardIndex();
}
swap(cards[a], cards[b]);
}
}
//Will sort all the cards in the cards vector. You must use the Card::LessThan() function in order to sort.
void Deck::SortDeck() {
// YOUR CODE HERE
}
//This function will return a numerical rating describing the quality of the deck shuffle.
float Deck::RateShuffleValues(Deck deck) {
int a, b, c, d;
for (int i = 0; i < cards.size(); i++) {
if (cards[i].GetCard() == "DA")
a = i;
else if (cards[i].GetCard() == "SA")
b = i;
else if (cards[i].GetCard() == "CA")
c = i;
else if (cards[i].GetCard() == "HA")
d = i;
}
return (abs(a - b) + abs(a - c) + abs(a - d)) / 3;
}
//This function will return a numerical rating describing the quality of the deck shuffle.
float Deck::RateShuffleSuits(Deck deck) {
int a, b, c, d;
for (int i = 0; i < cards.size(); i++) {
if (cards[i].GetCard() == "DA")
a = i;
else if (cards[i].GetCard() == "DK")
b = i;
else if (cards[i].GetCard() == "DQ")
c = i;
else if (cards[i].GetCard() == "DJ")
d = i;
}
return (abs(a - b) + abs(a - c) + abs(a - d)) / 3;
}
int Deck::GetRandomCardIndex() const {
return (rand() % cards.size());
}
void Deck::PrintDeck() const {
cout << endl;
for (int i = 0; i < cards.size(); i++) {
cout << cards[i].GetCard() << " ";
if ((i + 1) % 4 == 0) {
cout << endl;
}
}
cout << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

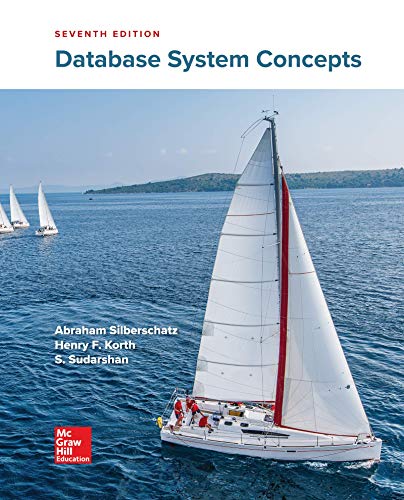
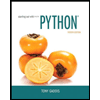
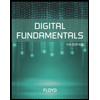
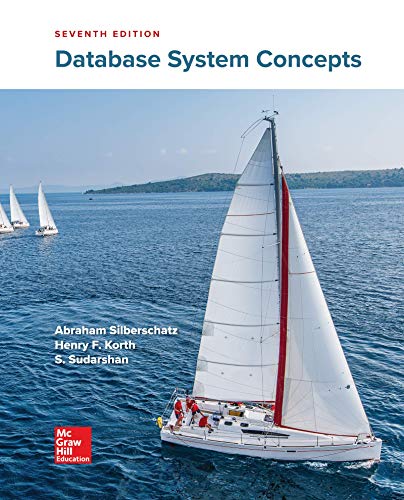
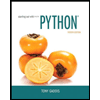
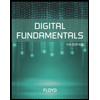
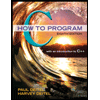
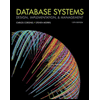
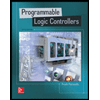