I am creating a web app using NodeJS and MySQL, but one of my endpoints keeps producing a 400 error that says: "Unknown column 'undefined' in 'field list'" This endpoint is supposed to insert a new event into the event table. It seems to read all the inserted data as undefined, but I can't figure out why. I'm running my test through Arc and I am inputing the data as: { "event_ID": "699694", "LocID": "Virtual", "Date": "2023-03-03", "start": "00:00:00", "end": "23:45:00", "Title": "Application Deadline: Summer Undergraduate Research Fellowship", "description": "This is a research fellowship for undergrads", "contact_name": "OUR", "contact_phone": "2543342220", "contact_email": "our@test.edu" } I have inserted data for all the field list but they all come up as undefined. The code for the endpoint is available below: app.post("/events", async (req, res, next) => { const { event_ID, locID, date, start, end, title, description, contact_name, contact_phone, contact_email } = req.body; const query = `INSERT INTO Events (event_ID, LocID, Date, start, end, Title, description, contact_name, contact_phone, contact_email) VALUES (${event_ID}, "${locID}", "${date}", "${start}", "${end}", "${title}", "${description}", "${contact_name}", "${contact_phone}", "${contact_email}")`; console.log(query); db.execute(query) .then((result) => { res.status(200).json(result); }) .catch((err) => { // error occured, query did not execute res.status(400).json({ error: err }); }); });
I am creating a web app using NodeJS and MySQL, but one of my endpoints keeps producing a 400 error that says: "Unknown column 'undefined' in 'field list'" This endpoint is supposed to insert a new event into the event table. It seems to read all the inserted data as undefined, but I can't figure out why. I'm running my test through Arc and I am inputing the data as: { "event_ID": "699694", "LocID": "Virtual", "Date": "2023-03-03", "start": "00:00:00", "end": "23:45:00", "Title": "Application Deadline: Summer Undergraduate Research Fellowship", "description": "This is a research fellowship for undergrads", "contact_name": "OUR", "contact_phone": "2543342220", "contact_email": "our@test.edu" } I have inserted data for all the field list but they all come up as undefined. The code for the endpoint is available below: app.post("/events", async (req, res, next) => { const { event_ID, locID, date, start, end, title, description, contact_name, contact_phone, contact_email } = req.body; const query = `INSERT INTO Events (event_ID, LocID, Date, start, end, Title, description, contact_name, contact_phone, contact_email) VALUES (${event_ID}, "${locID}", "${date}", "${start}", "${end}", "${title}", "${description}", "${contact_name}", "${contact_phone}", "${contact_email}")`; console.log(query); db.execute(query) .then((result) => { res.status(200).json(result); }) .catch((err) => { // error occured, query did not execute res.status(400).json({ error: err }); }); });
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I am creating a web app using NodeJS and MySQL, but one of my endpoints keeps producing a 400 error that says:
"Unknown column 'undefined' in 'field list'"
This endpoint is supposed to insert a new event into the event table. It seems to read all the inserted data as undefined, but I can't figure out why. I'm running my test through Arc and I am inputing the data as:
{
"event_ID": "699694",
"LocID": "Virtual",
"Date": "2023-03-03",
"start": "00:00:00",
"end": "23:45:00",
"Title": "Application Deadline: Summer Undergraduate Research Fellowship",
"description": "This is a research fellowship for undergrads",
"contact_name": "OUR",
"contact_phone": "2543342220",
"contact_email": "our@test.edu"
}
I have inserted data for all the field list but they all come up as undefined. The code for the endpoint is available below:
app.post("/events", async (req, res, next) => {
const { event_ID, locID, date, start, end, title, description, contact_name, contact_phone, contact_email } = req.body;
const query = `INSERT INTO Events (event_ID, LocID, Date, start, end, Title, description, contact_name, contact_phone, contact_email)
VALUES (${event_ID}, "${locID}", "${date}", "${start}", "${end}", "${title}", "${description}", "${contact_name}", "${contact_phone}", "${contact_email}")`;
console.log(query);
db.execute(query)
.then((result) => {
res.status(200).json(result);
})
.catch((err) => {
// error occured, query did not execute
res.status(400).json({ error: err });
});
});
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
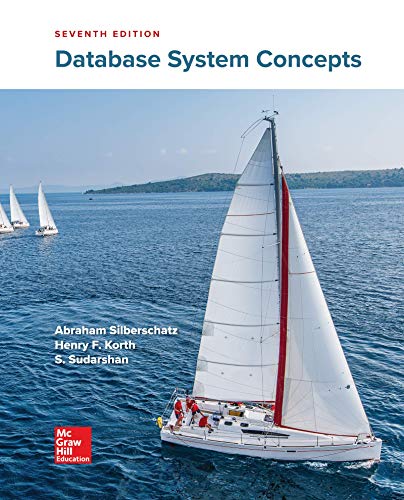
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
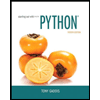
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
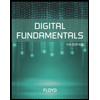
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
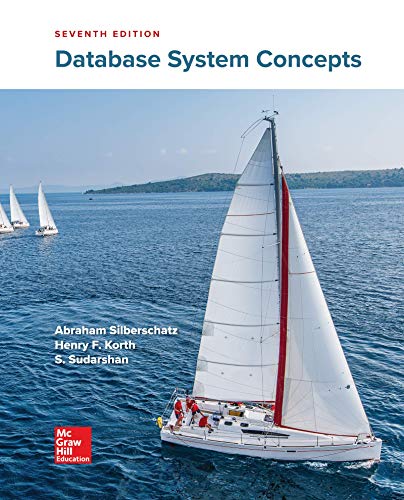
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
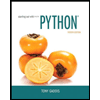
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
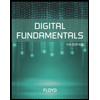
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
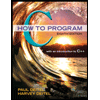
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
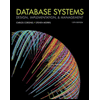
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
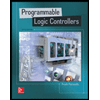
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education