I'm working on an assignment and i'm trying to create a to-do list in AndroidStudio. I've created the listview, edit text, switch and button, but I've hit a snag with the following: Create a new Java object to hold each todo item, it should contain a string with the text of the todo, and a boolean for if it is urgent or not. Create a List to hold all your items, and for the adapter to use for displaying them. When you click “Add”, you should create a new todo item and add it to the list, clear out the text in the EditText, and call “notifyDatasetChanged()” on your adapter so it refreshes. Implement a BaseAdapter to power the ListView. In AndroidStudio, type ctrl + O (the letter ‘O’, not number zero). From the list of inherited functions, implement these ones: int getCount() - This returns the number of rows that will be in your listView. In your case, it should be the number of strings in the array list object ( return list.size() ). Object getItem(int position) – This returns the item to show in the list at the specified position: ( return list.get(position) ) View getView(int position, View convertView, ViewGroup parent) – this returns the layout that will be positioned at the specified row in the list with the todo item text added. If the todo item is urgent, set the background of the row to red and change the color of the text to white. You can change the background color of the row after it’s been inflated with “view.setBackgroundColor(Color.RED)”, and by calling “setTextColor(Color.WHITE)” on the TextView. long getItemId(int position) – This is the database id of the item at position. For now, we aren’t using SQL, so just return the number: position. Set an onItemLongClick() listener to the ListView. Whenever you click on the list at a certain row, show an AlertDialog with the title: “Do you want to delete this?”, the message should display the “The selected row is: “, followed by the index that was clicked. The AlertDialog should also have a positive and negative button. The positive button should delete the item at the specified row and refresh the list.
I'm working on an assignment and i'm trying to create a to-do list in AndroidStudio. I've created the listview, edit text, switch and button, but I've hit a snag with the following: Create a new Java object to hold each todo item, it should contain a string with the text of the todo, and a boolean for if it is urgent or not. Create a List to hold all your items, and for the adapter to use for displaying them. When you click “Add”, you should create a new todo item and add it to the list, clear out the text in the EditText, and call “notifyDatasetChanged()” on your adapter so it refreshes. Implement a BaseAdapter to power the ListView. In AndroidStudio, type ctrl + O (the letter ‘O’, not number zero). From the list of inherited functions, implement these ones: int getCount() - This returns the number of rows that will be in your listView. In your case, it should be the number of strings in the array list object ( return list.size() ). Object getItem(int position) – This returns the item to show in the list at the specified position: ( return list.get(position) ) View getView(int position, View convertView, ViewGroup parent) – this returns the layout that will be positioned at the specified row in the list with the todo item text added. If the todo item is urgent, set the background of the row to red and change the color of the text to white. You can change the background color of the row after it’s been inflated with “view.setBackgroundColor(Color.RED)”, and by calling “setTextColor(Color.WHITE)” on the TextView. long getItemId(int position) – This is the database id of the item at position. For now, we aren’t using SQL, so just return the number: position. Set an onItemLongClick() listener to the ListView. Whenever you click on the list at a certain row, show an AlertDialog with the title: “Do you want to delete this?”, the message should display the “The selected row is: “, followed by the index that was clicked. The AlertDialog should also have a positive and negative button. The positive button should delete the item at the specified row and refresh the list.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I'm working on an assignment and i'm trying to create a to-do list in AndroidStudio. I've created the listview, edit text, switch and button, but I've hit a snag with the following:
- Create a new Java object to hold each todo item, it should contain a string with the text of the todo, and a boolean for if it is urgent or not. Create a List to hold all your items, and for the adapter to use for displaying them.
- When you click “Add”, you should create a new todo item and add it to the list, clear out the text in the EditText, and call “notifyDatasetChanged()” on your adapter so it refreshes.
- Implement a BaseAdapter to power the ListView. In AndroidStudio, type ctrl + O (the letter ‘O’, not number zero). From the list of inherited functions, implement these ones:
-
- int getCount() - This returns the number of rows that will be in your listView. In your case, it should be the number of strings in the array list object ( return list.size() ).
- Object getItem(int position) – This returns the item to show in the list at the specified position: ( return list.get(position) )
- View getView(int position, View convertView, ViewGroup parent) – this returns the layout that will be positioned at the specified row in the list with the todo item text added. If the todo item is urgent, set the background of the row to red and change the color of the text to white. You can change the background color of the row after it’s been inflated with “view.setBackgroundColor(Color.RED)”, and by calling “setTextColor(Color.WHITE)” on the TextView.
- long getItemId(int position) – This is the
database id of the item at position. For now, we aren’t using SQL, so just return the number: position.
- Set an onItemLongClick() listener to the ListView. Whenever you click on the list at a certain row, show an AlertDialog with the title: “Do you want to delete this?”, the message should display the “The selected row is: “, followed by the index that was clicked.
- The AlertDialog should also have a positive and negative button. The positive button should delete the item at the specified row and refresh the list.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step 1: Introduction
VIEWStep 2: To create a to-do list in AndroidStudio, you can follow these steps:
VIEWStep 3: Create a List to hold all the todo items. For example:
VIEWStep 4: Implement a BaseAdapter to power the ListView. Override the following methods in the adapter:
VIEWStep 5: Set an onItemLongClick() listener to the ListView. Inside the listener
VIEWStep 6: When the "Add" button is clicked, create a new todo item and add it to the list.
VIEWSolution
VIEWStep by step
Solved in 7 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
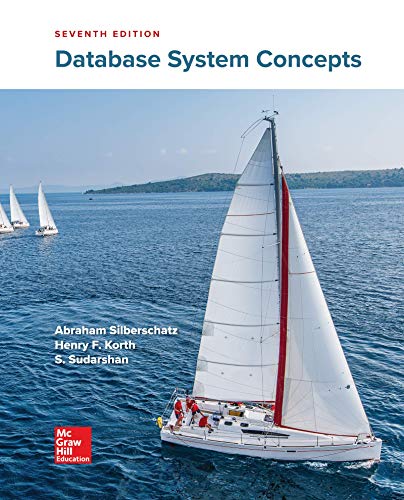
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
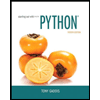
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
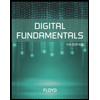
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
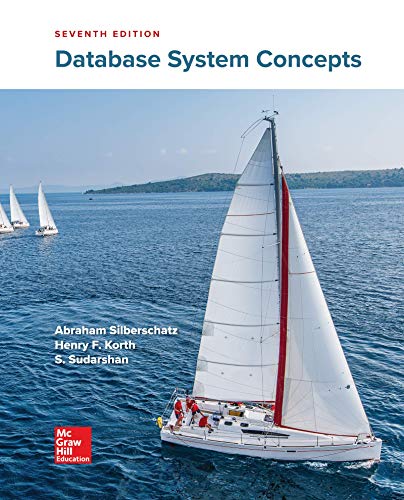
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
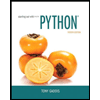
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
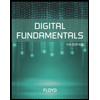
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
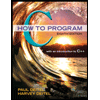
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
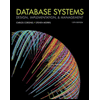
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
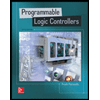
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education