I am creating a digital clock and I am trying to make sure that it does not allow an hour less than zero. All of my others paramters are being met (the clock is being created at hour zero and is creating at hour 23) however, I can't figure out why it is allowing hours that are less than zero. This is my code: public class DigitalClock { private int currentHour; private int currentMinutes; public int getHour() { return currentHour; } public void setHour(int currentHour) { this.currentHour = currentHour; } public int getMinutes() { return currentMinutes; } public void setMinutes(int currentMinutes) { this.currentMinutes = currentMinutes; } public DigitalClock (int hour, int minutes) { if (hour <= 0 && hour <= 23) currentHour = hour; else currentHour = 0; if (0 <= minutes && minutes <=59) currentMinutes = minutes; else currentMinutes = 0; } }
I am creating a digital clock and I am trying to make sure that it does not allow an hour less than zero. All of my others paramters are being met (the clock is being created at hour zero and is creating at hour 23) however, I can't figure out why it is allowing hours that are less than zero. This is my code:
public class DigitalClock
{
private int currentHour;
private int currentMinutes;
public int getHour()
{
return currentHour;
}
public void setHour(int currentHour)
{
this.currentHour = currentHour;
}
public int getMinutes()
{
return currentMinutes;
}
public void setMinutes(int currentMinutes)
{
this.currentMinutes = currentMinutes;
}
public DigitalClock (int hour, int minutes)
{
if (hour <= 0 && hour <= 23)
currentHour = hour;
else
currentHour = 0;
if (0 <= minutes && minutes <=59)
currentMinutes = minutes;
else
currentMinutes = 0;
}
}

Solution:
You have done a minor mistake here-
if (hour <= 0 && hour <= 23)
currentHour = hour;
The right condition should be: (hour >= 0 && hour <= 23)
Now, everything is alright.
Step by step
Solved in 3 steps with 2 images

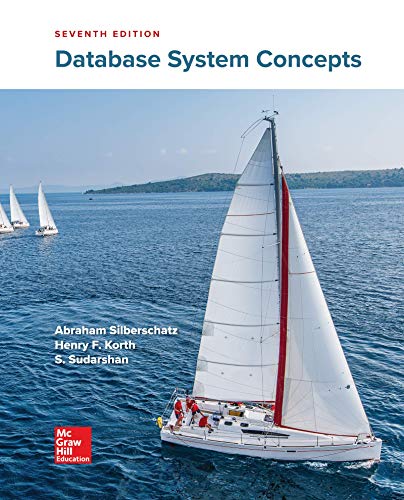
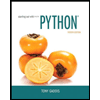
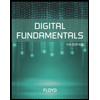
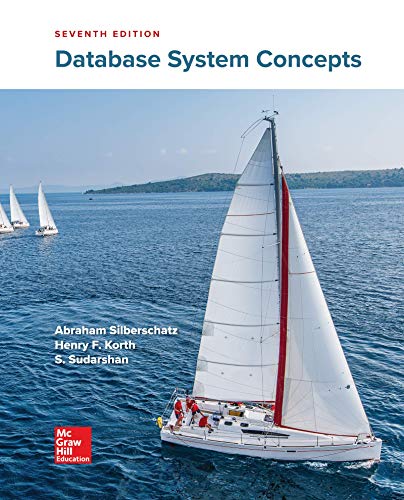
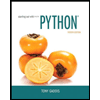
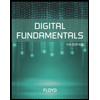
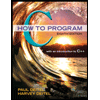
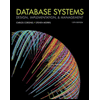
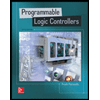