how to make a scanner to get speed from the user and add the speed with the last value of the object for example Honda (speed+0.2)
question for Java a class demo has three objects
Car car = new Car("make:Honda","color:Black",0.2);
Car car2 = new Car("make:Ford","color:blue", 0.5);
Car car3 = new Car("make:Tesla","color:silver",1.2);
how to make a scanner to get speed from the user and add the speed with the last value of the object for example Honda (speed+0.2)

To add the speed with last value of the object, we need to use the statement this.Speed += maxSpeed; in the setter method of speed and to check the updated value, we need to use the getter method for speed.
import java.util.Scanner;
public class Car
{
private String model;
private String color;
private double Speed;
public Car(String model, String color, double Speed)
{
this.model = model;
this.color = color;
this.Speed = Speed;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double getSpeed() {
return Speed;
}
public void setSpeed(double maxSpeed) {
this.Speed += maxSpeed;
}
public static void main(String[] args) {
Scanner Keyboard=new Scanner(System.in);
Car car1 = new Car("make:Honda","color:Black",0.2);
System.out.print("Enter the speed to upgrade:");
double newspeed=Keyboard.nextDouble();
car1.setSpeed(newspeed);
System.out.println(" New speed "+car1.getSpeed());
}
}
Step by step
Solved in 2 steps with 2 images

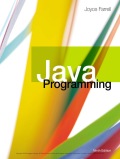
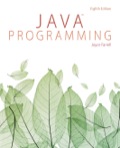
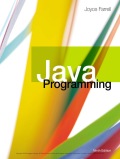
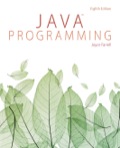
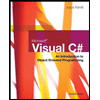
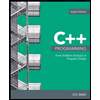