How to add more comments in these code
How to add more comments in these code
# matrics addition
def sum3(a, b):
sum_matrics = np.zeros((3, 3), dtype=float)
for i in range(3):
for j in range(3):
a_ele = a[i][j]
b_ele = b[i][j]
# The elements in the corresponding positions are summed
sum_matrics[i][j] = a_ele + b_ele
return sum_matrics
# scalar multiplication
def scalar3(a, scalar):
scalar_matrics = np.zeros((3, 3), dtype=float)
for i in range(3):
for j in range(3):
a_ele = a[i][j]
# Each element is multiplied by a factor
scalar_matrics[i][j] = scalar * a_ele
return scalar_matrics
# matrics production
def product3(a, b):
product_matrics = np.zeros((3, 3), dtype=float)
for i in range(3):
for j in range(3):
tmp0 = a[i][0] * b[0][j]
tmp1 = a[i][1] * b[1][j]
tmp2 = a[i][2] * b[2][j]
product_matrics[i][j] = tmp0 + tmp1 + tmp2
return product_matrics
if __name__ == "__main__":
while 1:
# get the user input
opera_num = input(
'Please input the operation number: \n1 Matrics Addition \n2 Scalar Multiplication \n3 Product of Two Matrics \n4 Exis \n')
# matrics addition
if opera_num == '1':
input_str = input('Please input two 3*3 matircs:\nfor example:\n1 2 3 4 5 6 7 8 9,1 2 3 4 5 6 7 8 9\n')
a = np.zeros((3, 3), dtype=float)
b = np.zeros((3, 3), dtype=float)
inputs = input_str.split(',')
input0 = inputs[0].split()
input1 = inputs[1].split()
try:
for i in range(3):
for j in range(3):
a[i][j] = float(input0[i * 3 + j])
b[i][j] = float(input1[i * 3 + j])
except:
print('wrong input, please start again')
continue
print(sum3(a, b))
# scalar multiplication
elif opera_num == '2':
input_str = input('Please input the scalar and a 3*3 matrics:\nfor example:\n1 2 3 4 5 6 7 8 9,2\n')
a = np.zeros((3, 3), dtype=float)
b = 0
inputs = input_str.split(',')
input0 = inputs[0].split()
input1 = inputs[1]
try:
for i in range(3):
for j in range(3):
a[i][j] = float(input0[i * 3 + j])
b = float(input1)
except:
print('Wrong input, please start again')
continue
print(scalar3(a, b))
# matrics production
elif opera_num == '3':
input_str = input('Please input two 3*3 matrics:\nfor example:\n1 2 3 4 5 6 7 8 9,1 2 3 4 5 6 7 8 9\n')
a = np.zeros((3, 3), dtype=float)
b = np.zeros((3, 3), dtype=float)
inputs = input_str.split(',')
input0 = inputs[0].split()
input1 = inputs[1].split()
try:
for i in range(3):
for j in range(3):
a[i][j] = float(input0[i * 3 + j])
b[i][j] = float(input1[i * 3 + j])
except:
print('Wrong input, please start again')
continue
print(product3(a, b))
# Exis
elif opera_num == '4':
print('Bye Bye ~')
break
else:
print('Wrong input, please start again')

Step by step
Solved in 2 steps

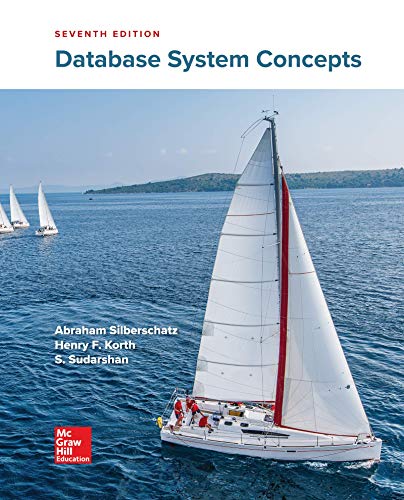
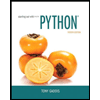
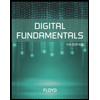
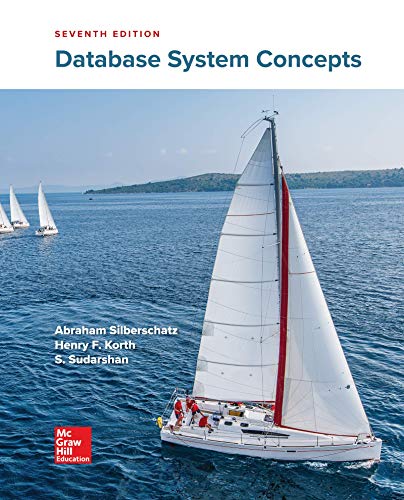
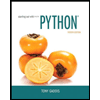
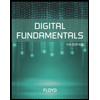
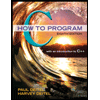
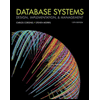
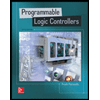