Please help me fix the errors, I've been try to fix it but it gives me new errors. I can only edit the code in studentMain.cpp, I cannot edit the code in bubble.cpp, bubble.h, and student.h. //bubble.cpp #include #include "student.h" void bubble(student *array[], int size) { int x; int y; student *temp = NULL; for(x = 0; x < size; x++) { for(y = 0; y < size-1; y++) { if(array[y]->mean > array[y+1]->mean) { temp = array[y+1]; array[y+1] = array[y]; array[y] = temp; } } } return; } //bubble.h void bubble(student *array[], int size); //student.h typedef struct student_info{ char *first; char *last; int exam1; int exam2; int exam3; float mean; } student; //studentMain.cpp #include #include #include "student.h" #include "bubble.h" int main() { // Create an array of 19 student pointers student *students[19]; // Allocate space for each student in the array for (int i = 0; i < 19; i++) { students[i] = (student *)malloc(sizeof(student)); } // Read in the data from the file FILE *fp = fopen("students.txt", "r"); if (fp == NULL) { printf("Error opening file\n"); return 1; } for (int i = 0; i < 19; i++) { fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3); // Calculate the student's mean students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0; } fclose(fp); // Sort the students using the bubble sort algorithm bubble(students, 19); // Find the mean, minimum, maximum, and median of the grades float mean = 0.0; float min = students[0]->mean; float max = students[18]->mean; float median = students[9]->mean; for (int i = 0; i < 19; i++) { mean += students[i]->mean; } mean /= 19.0; // Print the class statistics printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median); // Print the student averages for (int i = 0; i < 19; i++) { printf("%s %s %f\n", students[i]->first, students[i]->last); printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean); }
Please help me fix the errors, I've been try to fix it but it gives me new errors. I can only edit the code in studentMain.cpp, I cannot edit the code in bubble.cpp, bubble.h, and student.h.
//bubble.cpp
#include <stdlib.h>
#include "student.h"
void bubble(student *array[], int size)
{
int x;
int y;
student *temp = NULL;
for(x = 0; x < size; x++) {
for(y = 0; y < size-1; y++) {
if(array[y]->mean > array[y+1]->mean) {
temp = array[y+1];
array[y+1] = array[y];
array[y] = temp;
}
}
}
return;
}
//bubble.h
void bubble(student *array[], int size);
//student.h
typedef struct student_info{
char *first;
char *last;
int exam1;
int exam2;
int exam3;
float mean;
} student;
//studentMain.cpp
#include <stdio.h>
#include <stdlib.h>
#include "student.h"
#include "bubble.h"
int main()
{
// Create an array of 19 student pointers
student *students[19];
// Allocate space for each student in the array
for (int i = 0; i < 19; i++) {
students[i] = (student *)malloc(sizeof(student));
}
// Read in the data from the file
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
for (int i = 0; i < 19; i++) {
fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3);
// Calculate the student's mean
students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0;
}
fclose(fp);
// Sort the students using the bubble sort
bubble(students, 19);
// Find the mean, minimum, maximum, and median of the grades
float mean = 0.0;
float min = students[0]->mean;
float max = students[18]->mean;
float median = students[9]->mean;
for (int i = 0; i < 19; i++) {
mean += students[i]->mean;
}
mean /= 19.0;
// Print the class statistics
printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median);
// Print the student averages
for (int i = 0; i < 19; i++) {
printf("%s %s %f\n", students[i]->first, students[i]->last);
printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean);
}
// Free the allocated memory for each student
for (int i = 0; i < 19; i++) {
free(students[i]);
}
return 0;
}
![studentMain.cpp: In function 'int main()':
studentMain.cpp:40:20: warning: format 'f' expects a matching 'double' argument
printf("%s %s f\n", students[i]->first, students[i]->last);
[-Wformat=]
40 |
I
|
|
double
bubble.h:1:13: error: variable or field 'bubble' declared void
1 | void bubble (student *array[], int size);
برای ما برای ار برس دار
bubble.h:1:13: error: 'student' was not declared in this scope
bubble.h:1:22: error: 'array' was not declared in this scope
1 | void bubble (student *array[], int size);
bubble.h:1:28: error: expected primary-expression before ¹]' token
1 | void bubble (student *array[], int size);
bubble.h:1:31: error: expected primary-expression before 'int'
1 | void bubble (student *array[], int size);
I
الای ارسی مالی](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff76fb667-0622-451e-86fc-d3866765f8e5%2F9e8d027e-7a21-45bf-aa68-6b959e726bf6%2F5tgp4y_processed.png&w=3840&q=75)

Hello! I understand that diving into code can sometimes be overwhelming, especially when you're faced with error messages that might seem cryptic. However, no worries – we'll work through them step by step. Your code consists of the main student file, a utility for the bubble sort, and two headers that structure the functionalities. Let's systematically identify the issues and rectify them in studentMain.cpp
.
Step by step
Solved in 3 steps

Hi, it is still giving me the same 5 errors as last time even after modifying the code. I tried reordering #include "student.h" and #include "bubble.h" in studentMain.cpp but it gave me more errors. Is this happening because I am compiling with the wrong command, I am compiling it all together like this: g++ studentMain.cpp bubble.cpp bubble.h student.h grades.txt. I have the modified code below and a reminder that I can only edit the code in the studentMain.cpp file:
//studentMain.cpp
#include <stdio.h>
#include <stdlib.h>
#include "student.h"
#include "bubble.h"
int main()
{
// Create an array of 19 student pointers
student *students[19];
// Allocate space for each student in the array
for (int i = 0; i < 19; i++) {
students[i] = (student *)malloc(sizeof(student));
}
// Read in the data from the file
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
for (int i = 0; i < 19; i++) {
fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3);
// Calculate the student's mean
students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0;
}
fclose(fp);
// Sort the students using the bubble sort
bubble(students, 19);
// Find the mean, minimum, maximum, and median of the grades
float mean = 0.0;
float min = students[0]->mean;
float max = students[18]->mean;
float median = students[9]->mean;
for (int i = 0; i < 19; i++) {
mean += students[i]->mean;
}
mean /= 19.0;
// Print the class statistics
printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median);
// Print the student averages
for (int i = 0; i < 19; i++) {
printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean);
}
// Free the allocated memory for each student
for (int i = 0; i < 19; i++) {
free(students[i]);
}
return 0;
}
//bubble.cpp
#include <stdlib.h>
#include "student.h"
void bubble(student *array[], int size)
{
int x;
int y;
student *temp = NULL;
for(x = 0; x < size; x++) {
for(y = 0; y < size-1; y++) {
if(array[y]->mean > array[y+1]->mean) {
temp = array[y+1];
array[y+1] = array[y];
array[y] = temp;
}
}
}
return;
}
//bubble.h
void bubble(student *array[], int size);
//student.h
typedef struct student_info{
char *first;
char *last;
int exam1;
int exam2;
int exam3;
float mean;
} student;
//grades.txt
CSCE1040
Erica Sanders 75 89 67
Kelley Cummings 74 70 79
Jamie Reynolds 64 52 66
Shawna Huff 80 88 61
Muriel Holmes 81 74 79
Marion Harmon 77 64 69
Catherine Moss 51 80 73
Kristin Fernandez 86 69 81
Elsa Alvarado 63 77 67
Cora Spencer 76 79 71
Valerie Olson 85 78 79
Anne Singleton 85 87 65
Rene Boone 85 85 77
James Morgan 69 86 51
Cedric Haynes 72 73 88
Elijah Snyder 65 92 91
Roger Howard 79 95 71
Archie Black 70 81 63
Melvin Watkins 66 67 72
![bubble.h:1:13: error: variable or field 'bubble' declared void
1 | void bubble (student *array[], int size);
م م م م کی برسی مالی
bubble.h:1:13: error: 'student' was not declared in this scope
bubble.h:1:22: error: 'array' was not declared in this scope
1 | void bubble (student *array[], int size);
عا م کی برسی مالی
bubble.h:1:28: error: expected primary-expression before ¹]' token
1 | void bubble (student *array[], int size);
I
bubble.h:1:31: error: expected primary-expression before 'int'
1 | void bubble (student *array[], int size);
I](https://content.bartleby.com/qna-images/question/f76fb667-0622-451e-86fc-d3866765f8e5/e935ebba-2484-472a-ab97-32141dcbefd5/5312jt_thumbnail.png)
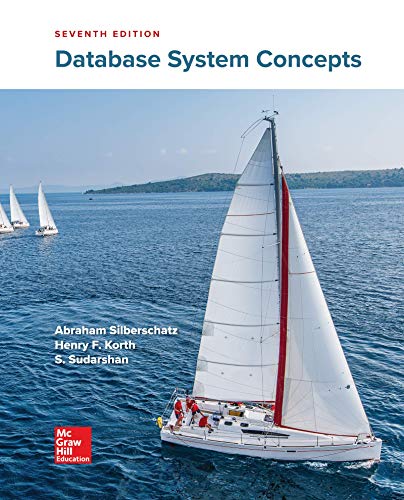
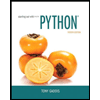
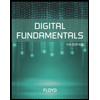
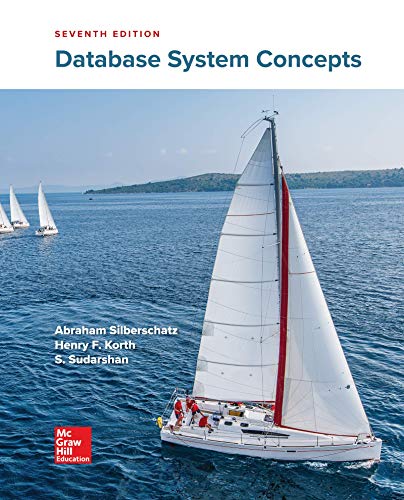
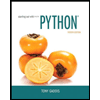
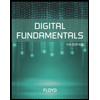
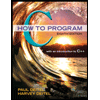
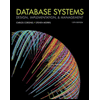
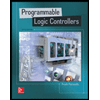