Part 2: Coding 1. Write a complete Java program named FindAverage that contains the following: a. A main method that asks the user to provide the number of rows and columns for a 2- dimensional array of integers. b. A main method calls the getArray() method that creates the 2D array of specified size and populates it with random values from 0 to 100. c. A main method prints the elements of the 2D array created by getArray0. d. A main method calls the printAverage method that will:
Part 2: Coding 1. Write a complete Java program named FindAverage that contains the following: a. A main method that asks the user to provide the number of rows and columns for a 2- dimensional array of integers. b. A main method calls the getArray() method that creates the 2D array of specified size and populates it with random values from 0 to 100. c. A main method prints the elements of the 2D array created by getArray0. d. A main method calls the printAverage method that will:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:## Part 2: Coding
### Task
Write a complete Java program named `FindAverage` that contains the following:
1. **Main Method Requirements:**
- **User Input:**
- Ask the user to provide the number of rows and columns for a 2-dimensional array of integers.
- **Array Creation:**
- A main method must call the `getArray()` method to create the 2D array of the specified size.
- Populate the array with random values from 0 to 100.
- **Print Array:**
- Print the elements of the 2D array created by `getArray()`.
- **Compute and Print Average:**
- Call the `printAverage` method that will:
- Receive the 2-dimensional array as input.
- Calculate the average of elements in this array.
- Display the average on the console.
2. **Output Requirements:**
- The average of all elements should be formatted as `xxx.xx`.
3. **Additional Guidance:**
- **Hint:** You can print the 2D array at any point in the program using either a traditional for loop, foreach loop, or available methods in the `java.util` package.
- **Program Comments:**
- Add comments to your program. Start your program with the following header:
```java
/******************************************
```
Ensure that the program is well-commented and follows standard coding practices for clarity and maintainability.
Expert Solution

Step 1
logic to generate random number in range:-
Min + (int)(Math.random() * ((Max - Min) + 1));
where set Min =1 and Max=100 (requirement)
Logic to get length of 2D array.
randArray.length to get number of rows
and randArray[i].length to get number of columns.
end.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
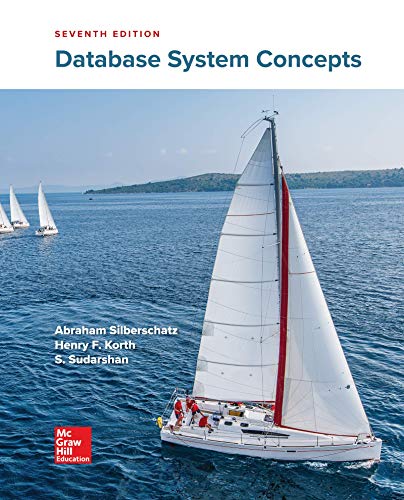
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
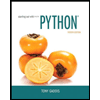
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
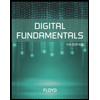
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
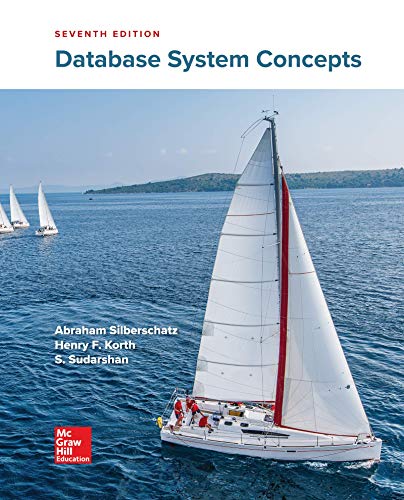
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
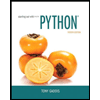
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
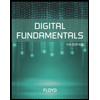
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
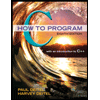
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
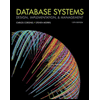
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
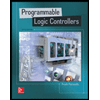
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education