Hi, here is my code that represent a MazeSolver. The maze contains 10 rows and 10 columns. Iam unable to make it presented in graphic version. Kindly assist. 10. . . . . . . . . G.#########.#########.#. . .#####.#.#######. . .#######. #########.#########. . S#######.######### import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;class MazeSolver {private int n; // Size of the maze (n x n)private char[][] maze; // Maze gridprivate boolean[][] visited; // To track visited cellsprivate int startX, startY, goalX, goalY; // Start and Goal positions// Constructor to initialize the mazepublic MazeSolver(String filename) throws IOException {readMaze(filename);visited = new boolean[n][n];}// Method to read the maze from a filepublic void readMaze(String filename) throws IOException {BufferedReader br = new BufferedReader(new FileReader(filename));n = Integer.parseInt(br.readLine()); // First line contains the size of the mazemaze = new char[n][n];for (int i = 0; i < n; i++) {String line = br.readLine();for (int j = 0; j < n; j++) {maze[i][j] = line.charAt(j);if (maze[i][j] == 'S') { // Identify start positionstartX = i;startY = j;} else if (maze[i][j] == 'G') { // Identify goal positiongoalX = i;goalY = j;}}}br.close();}// Display the current state of the mazepublic void displayMaze() {for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {System.out.print(maze[i][j]);}System.out.println();}}// Public method to initiate solving the mazepublic void solveMaze() {if (solveMaze(startX, startY)) {System.out.println("Path found!");} else {System.out.println("No path found.");}}// Private recursive method implementing DFS to find the pathprivate boolean solveMaze(int x, int y) {// Check if out of bounds or hitting a wall or already visitedif (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {return false;}// Check if reached the goalif (x == goalX && y == goalY) {return true;}// Mark current cell as visitedvisited[x][y] = true;// Explore in four directions: down, up, right, leftif (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {return true;}// Backtrack if no path is found in any directionvisited[x][y] = false;return false;}}
Hi, here is my code that represent a MazeSolver. The maze contains 10 rows and 10 columns. Iam unable to make it presented in graphic version. Kindly assist. 10. . . . . . . . . G.#########.#########.#. . .#####.#.#######. . .#######. #########.#########. . S#######.######### import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;class MazeSolver {private int n; // Size of the maze (n x n)private char[][] maze; // Maze gridprivate boolean[][] visited; // To track visited cellsprivate int startX, startY, goalX, goalY; // Start and Goal positions// Constructor to initialize the mazepublic MazeSolver(String filename) throws IOException {readMaze(filename);visited = new boolean[n][n];}// Method to read the maze from a filepublic void readMaze(String filename) throws IOException {BufferedReader br = new BufferedReader(new FileReader(filename));n = Integer.parseInt(br.readLine()); // First line contains the size of the mazemaze = new char[n][n];for (int i = 0; i < n; i++) {String line = br.readLine();for (int j = 0; j < n; j++) {maze[i][j] = line.charAt(j);if (maze[i][j] == 'S') { // Identify start positionstartX = i;startY = j;} else if (maze[i][j] == 'G') { // Identify goal positiongoalX = i;goalY = j;}}}br.close();}// Display the current state of the mazepublic void displayMaze() {for (int i = 0; i < n; i++) {for (int j = 0; j < n; j++) {System.out.print(maze[i][j]);}System.out.println();}}// Public method to initiate solving the mazepublic void solveMaze() {if (solveMaze(startX, startY)) {System.out.println("Path found!");} else {System.out.println("No path found.");}}// Private recursive method implementing DFS to find the pathprivate boolean solveMaze(int x, int y) {// Check if out of bounds or hitting a wall or already visitedif (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {return false;}// Check if reached the goalif (x == goalX && y == goalY) {return true;}// Mark current cell as visitedvisited[x][y] = true;// Explore in four directions: down, up, right, leftif (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {return true;}// Backtrack if no path is found in any directionvisited[x][y] = false;return false;}}
Chapter7: Characters, Strings, And The Stringbuilder
Section: Chapter Questions
Problem 3GZ
Related questions
Question
Hi, here is my code that represent a MazeSolver. The maze contains 10 rows and 10 columns. Iam unable to make it presented in graphic version. Kindly assist.
10
. . . . . . . . . G
.#########
.#########
.#. . .#####
.#.#######
. . .#######
. #########
.#########
. . S#######
.#########
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
class MazeSolver {
private int n; // Size of the maze (n x n)
private char[][] maze; // Maze grid
private boolean[][] visited; // To track visited cells
private int startX, startY, goalX, goalY; // Start and Goal positions
// Constructor to initialize the maze
public MazeSolver(String filename) throws IOException {
readMaze(filename);
visited = new boolean[n][n];
}
// Method to read the maze from a file
public void readMaze(String filename) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(filename));
n = Integer.parseInt(br.readLine()); // First line contains the size of the maze
maze = new char[n][n];
for (int i = 0; i < n; i++) {
String line = br.readLine();
for (int j = 0; j < n; j++) {
maze[i][j] = line.charAt(j);
if (maze[i][j] == 'S') { // Identify start position
startX = i;
startY = j;
} else if (maze[i][j] == 'G') { // Identify goal position
goalX = i;
goalY = j;
}
}
}
br.close();
}
// Display the current state of the maze
public void displayMaze() {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(maze[i][j]);
}
System.out.println();
}
}
// Public method to initiate solving the maze
public void solveMaze() {
if (solveMaze(startX, startY)) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
// Private recursive method implementing DFS to find the path
private boolean solveMaze(int x, int y) {
// Check if out of bounds or hitting a wall or already visited
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
return false;
}
// Check if reached the goal
if (x == goalX && y == goalY) {
return true;
}
// Mark current cell as visited
visited[x][y] = true;
// Explore in four directions: down, up, right, left
if (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {
return true;
}
// Backtrack if no path is found in any direction
visited[x][y] = false;
return false;
}
}
import java.io.FileReader;
import java.io.IOException;
class MazeSolver {
private int n; // Size of the maze (n x n)
private char[][] maze; // Maze grid
private boolean[][] visited; // To track visited cells
private int startX, startY, goalX, goalY; // Start and Goal positions
// Constructor to initialize the maze
public MazeSolver(String filename) throws IOException {
readMaze(filename);
visited = new boolean[n][n];
}
// Method to read the maze from a file
public void readMaze(String filename) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(filename));
n = Integer.parseInt(br.readLine()); // First line contains the size of the maze
maze = new char[n][n];
for (int i = 0; i < n; i++) {
String line = br.readLine();
for (int j = 0; j < n; j++) {
maze[i][j] = line.charAt(j);
if (maze[i][j] == 'S') { // Identify start position
startX = i;
startY = j;
} else if (maze[i][j] == 'G') { // Identify goal position
goalX = i;
goalY = j;
}
}
}
br.close();
}
// Display the current state of the maze
public void displayMaze() {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(maze[i][j]);
}
System.out.println();
}
}
// Public method to initiate solving the maze
public void solveMaze() {
if (solveMaze(startX, startY)) {
System.out.println("Path found!");
} else {
System.out.println("No path found.");
}
}
// Private recursive method implementing DFS to find the path
private boolean solveMaze(int x, int y) {
// Check if out of bounds or hitting a wall or already visited
if (x < 0 || x >= n || y < 0 || y >= n || maze[x][y] == '#' || visited[x][y]) {
return false;
}
// Check if reached the goal
if (x == goalX && y == goalY) {
return true;
}
// Mark current cell as visited
visited[x][y] = true;
// Explore in four directions: down, up, right, left
if (solveMaze(x + 1, y) || solveMaze(x - 1, y) || solveMaze(x, y + 1) || solveMaze(x, y - 1)) {
return true;
}
// Backtrack if no path is found in any direction
visited[x][y] = false;
return false;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
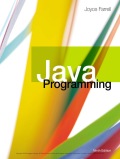
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
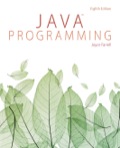
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
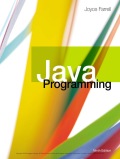
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
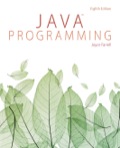
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
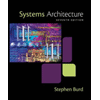
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning