1 // 234567890E 10 11 12 23 13 14 2 import java.util.Scanner; 3 import java.util.*; 7 public class Demo { { public static void main (String [] args) //Creates an inventory object to store inventor y values Inventory item = new Inventory(); 15 16 56789 17 18 19 20 21 23 24 25 26 27 22222222 //Creates a scanner to get input from user @SuppressWarnings("resource") Scanner keyboard = new Scanner(System.in); //Initilizes array list for names for inventory List Names = new ArrayList<>(); Names.add("Cap"); Names.add("Shirt"); Names.add("Jeans"); //Initilizes array list for prices for inventor <>(); List invPrices = new ArrayList y 28 29 30 223 31 32 33 invPrices.add(29.45); invPrices.add(35.65); invPrices.add(59.99); //Initilizes name and price variables for the 1 oop below 34 35 36 37 38 39 40 mmmmmm27 String name; 41 //Loop that adds items to the inventory until t hey choose to quit while (name != "q" || name != "Q") 45 23444 46 { 47 48 49 50 51 System.out.println( "Enter the name of the item: or Q to quit"); name = keyboard.next(); item.setItemName(name); String setName = item.getItemName(); Names.add(setName); System.out.println( "Enter the price of the item: "); 235 52 53 54 (); 56 555 double price = keyboard.nextDouble(); item.setItemPrice(price); double setPrice = item.getItemPrice invPrices.add(setPrice); System.out.println( "Enter q to quit or any other key to continue: " ); 57 58 59 60 2554 String quit = keyboard.next(); } 61 51 //Method that gets the total price for all item s in the inventory public double totalPrice (List 62 Prices) { 63 64 ៩ ២៤៨ ++) double total = 0; for (int i = 0; i < Prices.size(); i { total += Prices.get(i)%;B } return total; } 69 5648022237167008 79 //Prints out the inventory System.out.println( "Here is your inventory: "); for (int i = 0; i < Names.size(); i++) { String formatPrice = String.format( "%.2f", invPrices.get(i)); 81 82 83 System.out.println("Item: Price: + formatPrice); 8800 84 85 86 get(i) + 888 87 } + Names. 89 System.out.println( "Total price of your purchase will be + totalPrice(invPrices)); } 103 } 2222222222222 100 101 102 104 105 1 public class Inventory 234568∞ 7 9 { //Inventory class fields private double price; private String name; //No argument constructor: Initializs itemand pr ice 10 public Inventory () 11 { 12 13 14 15 16 17 SENAYA name = null; price = 0; } 20 //Constructor that accepts parameters for both i tem and name public Inventory (String iName, double iPrice) { name = iName; 22222222222222 23 24 price = iPrice; } //Mutator method for item name public void setItemName (String item) name= item; 25 { } //Accessor method for item name public String getItemName () return name; { 33 34 } 35 36 39 40 41 42 ARABAS w w w w //Mutator method for price ammount public void setItemPrice (double newPrice) 38 { price = newPrice; } 43 44 { 45 return price; 46 } //Accessor method for price amount public double getItemPrice () 47 48 }
1 // 234567890E 10 11 12 23 13 14 2 import java.util.Scanner; 3 import java.util.*; 7 public class Demo { { public static void main (String [] args) //Creates an inventory object to store inventor y values Inventory item = new Inventory(); 15 16 56789 17 18 19 20 21 23 24 25 26 27 22222222 //Creates a scanner to get input from user @SuppressWarnings("resource") Scanner keyboard = new Scanner(System.in); //Initilizes array list for names for inventory List Names = new ArrayList<>(); Names.add("Cap"); Names.add("Shirt"); Names.add("Jeans"); //Initilizes array list for prices for inventor <>(); List invPrices = new ArrayList y 28 29 30 223 31 32 33 invPrices.add(29.45); invPrices.add(35.65); invPrices.add(59.99); //Initilizes name and price variables for the 1 oop below 34 35 36 37 38 39 40 mmmmmm27 String name; 41 //Loop that adds items to the inventory until t hey choose to quit while (name != "q" || name != "Q") 45 23444 46 { 47 48 49 50 51 System.out.println( "Enter the name of the item: or Q to quit"); name = keyboard.next(); item.setItemName(name); String setName = item.getItemName(); Names.add(setName); System.out.println( "Enter the price of the item: "); 235 52 53 54 (); 56 555 double price = keyboard.nextDouble(); item.setItemPrice(price); double setPrice = item.getItemPrice invPrices.add(setPrice); System.out.println( "Enter q to quit or any other key to continue: " ); 57 58 59 60 2554 String quit = keyboard.next(); } 61 51 //Method that gets the total price for all item s in the inventory public double totalPrice (List 62 Prices) { 63 64 ៩ ២៤៨ ++) double total = 0; for (int i = 0; i < Prices.size(); i { total += Prices.get(i)%;B } return total; } 69 5648022237167008 79 //Prints out the inventory System.out.println( "Here is your inventory: "); for (int i = 0; i < Names.size(); i++) { String formatPrice = String.format( "%.2f", invPrices.get(i)); 81 82 83 System.out.println("Item: Price: + formatPrice); 8800 84 85 86 get(i) + 888 87 } + Names. 89 System.out.println( "Total price of your purchase will be + totalPrice(invPrices)); } 103 } 2222222222222 100 101 102 104 105 1 public class Inventory 234568∞ 7 9 { //Inventory class fields private double price; private String name; //No argument constructor: Initializs itemand pr ice 10 public Inventory () 11 { 12 13 14 15 16 17 SENAYA name = null; price = 0; } 20 //Constructor that accepts parameters for both i tem and name public Inventory (String iName, double iPrice) { name = iName; 22222222222222 23 24 price = iPrice; } //Mutator method for item name public void setItemName (String item) name= item; 25 { } //Accessor method for item name public String getItemName () return name; { 33 34 } 35 36 39 40 41 42 ARABAS w w w w //Mutator method for price ammount public void setItemPrice (double newPrice) 38 { price = newPrice; } 43 44 { 45 return price; 46 } //Accessor method for price amount public double getItemPrice () 47 48 }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Hello, I am having a bit of trouble in the demo class of my Java
![1
//
234567890E
10
11
12
23
13
14
2 import java.util.Scanner;
3 import java.util.*;
7 public class Demo
{
{
public static void main (String [] args)
//Creates an inventory object to store inventor
y values
Inventory item = new Inventory();
15
16
56789
17
18
19
20
21
23
24
25
26
27
22222222
//Creates a scanner to get input from user
@SuppressWarnings("resource")
Scanner keyboard = new Scanner(System.in);
//Initilizes array list for names for inventory
List<String> Names = new ArrayList<>();
Names.add("Cap");
Names.add("Shirt");
Names.add("Jeans");
//Initilizes array list for prices for inventor
<>();
List<Double> invPrices = new ArrayList
y
28
29
30
223
31
32
33
invPrices.add(29.45);
invPrices.add(35.65);
invPrices.add(59.99);
//Initilizes name and price variables for the 1
oop below
34
35
36
37
38
39
40
mmmmmm27
String name;
41
//Loop that adds items to the inventory until t
hey choose to quit
while (name != "q" || name != "Q")
45
23444
46
{
47
48
49
50
51
System.out.println(
"Enter the name of the item: or Q to quit");
name = keyboard.next();
item.setItemName(name);
String setName = item.getItemName();
Names.add(setName);
System.out.println(
"Enter the price of the item: ");
235
52
53
54
();
56
555
double price
=
keyboard.nextDouble();
item.setItemPrice(price);
double setPrice = item.getItemPrice
invPrices.add(setPrice);
System.out.println(
"Enter q to quit or any other key to continue:
"
);
57
58
59
60
2554
String quit
=
keyboard.next();
}
61
51
//Method that gets the total price for all item
s in the inventory
public double totalPrice (List<Double>
62
Prices)
{
63
64
៩ ២៤៨
++)
double total = 0;
for (int i = 0; i < Prices.size(); i
{
total += Prices.get(i)%;B
}
return total;
}
69
5648022237167008
79
//Prints out the inventory
System.out.println(
"Here is your inventory: ");
for (int i = 0; i < Names.size(); i++)
{
String formatPrice = String.format(
"%.2f", invPrices.get(i));
81
82
83
System.out.println("Item:
Price: + formatPrice);
8800
84
85
86
get(i) +
888
87
}
+ Names.
89
System.out.println(
"Total price of your purchase will be +
totalPrice(invPrices));
}
103 }
2222222222222
100
101
102
104
105](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca6ebb3e-d829-4b6b-baac-593cdb4771c8%2F94edb436-a9fb-4ec0-a322-d64bfe949048%2Fozrtbp7_processed.png&w=3840&q=75)
Transcribed Image Text:1
//
234567890E
10
11
12
23
13
14
2 import java.util.Scanner;
3 import java.util.*;
7 public class Demo
{
{
public static void main (String [] args)
//Creates an inventory object to store inventor
y values
Inventory item = new Inventory();
15
16
56789
17
18
19
20
21
23
24
25
26
27
22222222
//Creates a scanner to get input from user
@SuppressWarnings("resource")
Scanner keyboard = new Scanner(System.in);
//Initilizes array list for names for inventory
List<String> Names = new ArrayList<>();
Names.add("Cap");
Names.add("Shirt");
Names.add("Jeans");
//Initilizes array list for prices for inventor
<>();
List<Double> invPrices = new ArrayList
y
28
29
30
223
31
32
33
invPrices.add(29.45);
invPrices.add(35.65);
invPrices.add(59.99);
//Initilizes name and price variables for the 1
oop below
34
35
36
37
38
39
40
mmmmmm27
String name;
41
//Loop that adds items to the inventory until t
hey choose to quit
while (name != "q" || name != "Q")
45
23444
46
{
47
48
49
50
51
System.out.println(
"Enter the name of the item: or Q to quit");
name = keyboard.next();
item.setItemName(name);
String setName = item.getItemName();
Names.add(setName);
System.out.println(
"Enter the price of the item: ");
235
52
53
54
();
56
555
double price
=
keyboard.nextDouble();
item.setItemPrice(price);
double setPrice = item.getItemPrice
invPrices.add(setPrice);
System.out.println(
"Enter q to quit or any other key to continue:
"
);
57
58
59
60
2554
String quit
=
keyboard.next();
}
61
51
//Method that gets the total price for all item
s in the inventory
public double totalPrice (List<Double>
62
Prices)
{
63
64
៩ ២៤៨
++)
double total = 0;
for (int i = 0; i < Prices.size(); i
{
total += Prices.get(i)%;B
}
return total;
}
69
5648022237167008
79
//Prints out the inventory
System.out.println(
"Here is your inventory: ");
for (int i = 0; i < Names.size(); i++)
{
String formatPrice = String.format(
"%.2f", invPrices.get(i));
81
82
83
System.out.println("Item:
Price: + formatPrice);
8800
84
85
86
get(i) +
888
87
}
+ Names.
89
System.out.println(
"Total price of your purchase will be +
totalPrice(invPrices));
}
103 }
2222222222222
100
101
102
104
105

Transcribed Image Text:1 public class Inventory
234568∞
7
9
{
//Inventory class fields
private double price;
private String name;
//No argument constructor: Initializs itemand pr
ice
10 public Inventory ()
11
{
12
13
14
15
16
17
SENAYA
name = null;
price
= 0;
}
20
//Constructor that accepts parameters for both i
tem and name
public Inventory (String iName, double iPrice)
{
name = iName;
22222222222222
23
24
price
=
iPrice;
}
//Mutator method for item name
public void setItemName (String item)
name= item;
25
{
}
//Accessor method for item name
public String getItemName ()
return name;
{
33
34
}
35
36
39
40
41
42
ARABAS w w w w
//Mutator method for price ammount
public void setItemPrice (double newPrice)
38
{
price
= newPrice;
}
43
44
{
45
return price;
46
}
//Accessor method for price amount
public double getItemPrice ()
47
48 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
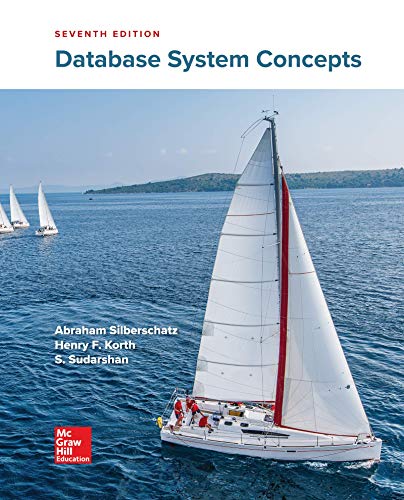
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
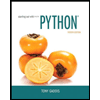
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
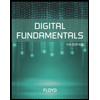
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
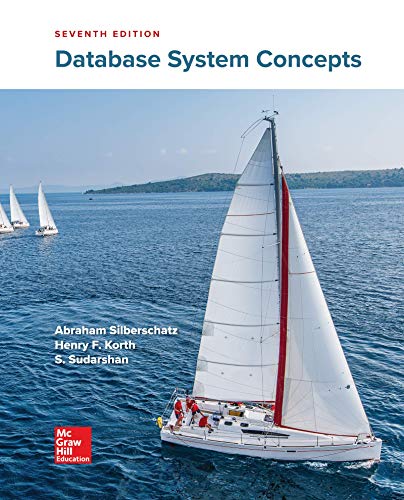
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
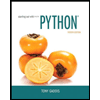
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
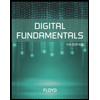
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
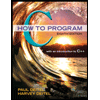
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
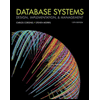
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
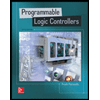
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education