Hey I need to change this program below in a way that it saves and reads back the addresses utilizing Object Serialization. import java.io.*; import java.util.Scanner; public class AddressBook { //declaring the main function public static void main(String []args){ //creating the scanner object to get the input from the user Scanner sc = new Scanner(System.in); //printing the welcome message System.out.println("Welcome to Address Book!"); //asking the user to enter the details System.out.println("Enter last name, first name, phone number and email address: "); System.out.println("Enter EOF to end input"); //initialising the filewriter to write into the file FileWriter fileWriter; //initialising the bufferedwrite to write line into the file BufferedWriter writer; //initialising the filereader to read data from the file FileReader fileReader; //initialising the bufferedreader to read lines from the file BufferedReader reader; //implementing with the try catch block to handle exceptions try { //creating the file name addresses.txt fileWriter = new FileWriter("addresses.txt"); //creating the buffered writer to write into file writer = new BufferedWriter(fileWriter); while(true) { //getting the input from the user String line = sc.nextLine(); //if user enter EOF then break from getting the input if (line.equals("EOF")) break; //writing into the file writer.write(line+"\n"); } //closing the file after writing into the file writer.close(); }catch(IOException e){ //handling the exceptions System.out.println("Error in Opening the file"); } //prompting the user to input the last name of the person System.out.println("Enter the last name of the person: "); String name = sc.next(); //creating the try catch block to reading and handling exceptions while reading the file try { // declaring the filereader to read the data from the file fileReader = new FileReader("addresses.txt"); //declaring the buffered reader to read line from the file reader = new BufferedReader(fileReader); String line; boolean found = false; while((line = reader.readLine()) != null){ //getting the data from the file and splitting the data into the array String []details = line.split(" "); //if details are found than print the details if(details[0].equals(name)){ System.out.println("Details Found: " + details[1] + " " + details[0] +" "+ details[2]); found = true; break; } } //if details are not found than promt that no details present if(found == false){ System.out.println("Details are not present in the file"); } reader.close(); }catch(IOException e){ //handling the exceptions System.out.println("Error in Opening the file"); } } }
Hey I need to change this program below in a way that it saves and reads back the addresses utilizing Object Serialization.
import java.io.*;
import java.util.Scanner;
public class AddressBook {
//declaring the main function
public static void main(String []args){
//creating the scanner object to get the input from the user
Scanner sc = new Scanner(System.in);
//printing the welcome message
System.out.println("Welcome to Address Book!");
//asking the user to enter the details
System.out.println("Enter last name, first name, phone number and email address: ");
System.out.println("Enter EOF to end input");
//initialising the filewriter to write into the file
FileWriter fileWriter;
//initialising the bufferedwrite to write line into the file
BufferedWriter writer;
//initialising the filereader to read data from the file
FileReader fileReader;
//initialising the bufferedreader to read lines from the file
BufferedReader reader;
//implementing with the try catch block to handle exceptions
try {
//creating the file name addresses.txt
fileWriter = new FileWriter("addresses.txt");
//creating the buffered writer to write into file
writer = new BufferedWriter(fileWriter);
while(true) {
//getting the input from the user
String line = sc.nextLine();
//if user enter EOF then break from getting the input
if (line.equals("EOF"))
break;
//writing into the file
writer.write(line+"\n");
}
//closing the file after writing into the file
writer.close();
}catch(IOException e){
//handling the exceptions
System.out.println("Error in Opening the file");
}
//prompting the user to input the last name of the person
System.out.println("Enter the last name of the person: ");
String name = sc.next();
//creating the try catch block to reading and handling exceptions while reading the file
try {
// declaring the filereader to read the data from the file
fileReader = new FileReader("addresses.txt");
//declaring the buffered reader to read line from the file
reader = new BufferedReader(fileReader);
String line;
boolean found = false;
while((line = reader.readLine()) != null){
//getting the data from the file and splitting the data into the array
String []details = line.split(" ");
//if details are found than print the details
if(details[0].equals(name)){
System.out.println("Details Found: " + details[1] + " " + details[0] +" "+ details[2]);
found = true;
break;
}
}
//if details are not found than promt that no details present
if(found == false){
System.out.println("Details are not present in the file");
}
reader.close();
}catch(IOException e){
//handling the exceptions
System.out.println("Error in Opening the file");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

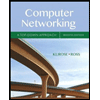
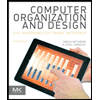
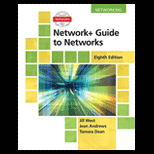
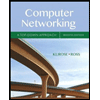
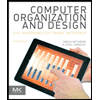
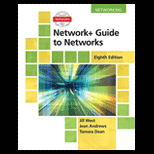
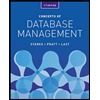
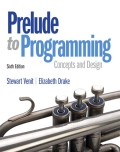
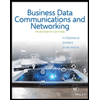