Hello. Please help me complete the code. A picture of the problem statement has been provided, as well as the code to be completed and the driver code. Please make sure the test cases are passed. /** This class models a ball that bounces off walls. */ public class Ball {
Hello. Please help me complete the code. A picture of the problem statement has been provided, as well as
the code to be completed and the driver code.
Please make sure the test cases are passed.
/**
This class models a ball that bounces off walls.
*/
public class Ball
{
// Instance variables
/* Your code goes here */
/**
Constructs a ball at (0, 0) traveling northeast.
@param rightWall the position of the wall to the right
@param topWall the position of the wall at the top
*/
public Ball(int rightWall, int topWall)
{
/* Your code goes here */
}
/**
Moves the ball.
*/
public void move()
{
/* Your code goes here */
}
/**
Turns the ball direction 90 degrees clockwise.
*/
private void turn()
{
/* Your code goes here */
}
/**
Gets the current x-position.
@return the x-position
*/
public int getX()
{
/* Your code goes here */
}
/**
Gets the current y-position.
@return the y-position
*/
public int getY()
{
/* Your code goes here */
}
}
public class BallTester
{
public static void main(String[] args)
{
Ball ball1 = new Ball(6, 4);
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 0 0");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 1 1");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 2 2");
ball1.move();
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 4 4");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 5 3");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 6 2");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 5 1");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 4 0");
ball1.move();
System.out.println(ball1.getX() + " " + ball1.getY());
System.out.println("Expected: 3 1");
Ball ball2 = new Ball(10, 10);
for (int i = 1; i <= 12; i++) { ball2.move(); }
System.out.println(ball2.getX() + " " + ball2.getY());
System.out.println("Expected: 8 8");
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

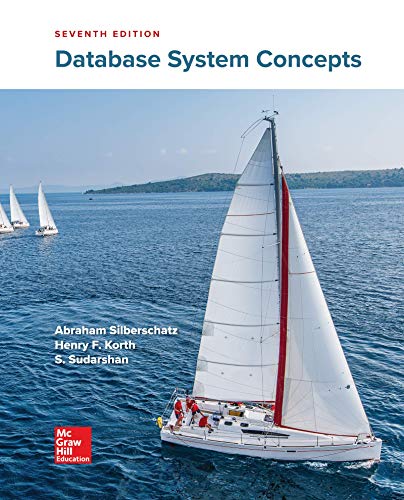
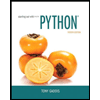
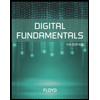
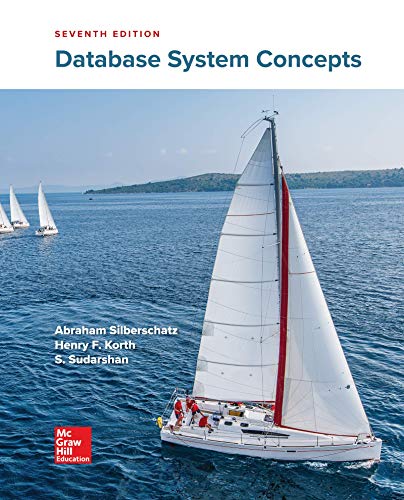
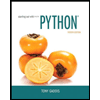
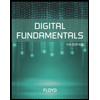
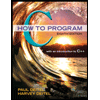
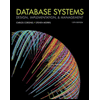
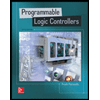