Hello. I'm working on decompressing text in run length encoding in C++. I'm almost finished with the code but I'm confused about how to fix the whitespace. Program requirements: Do not forget to close the file after you are done with it. You must use a while loop to handle the loop reading information from the file. You must use a for loop to handle the count/char output. Use the sample runs to ensure that all output is spelled/spaced correctly. Hint: You will need to make use of .get(ch) to "throw out" the irrelavent spaces. With stdin you would have used std::cin.get(ch), however with file objects remember you will need to use file_obj.get(ch) .
Hello. I'm working on decompressing text in run length encoding in C++. I'm almost finished with the code but I'm confused about how to fix the whitespace. Program requirements: Do not forget to close the file after you are done with it. You must use a while loop to handle the loop reading information from the file. You must use a for loop to handle the count/char output. Use the sample runs to ensure that all output is spelled/spaced correctly. Hint: You will need to make use of .get(ch) to "throw out" the irrelavent spaces. With stdin you would have used std::cin.get(ch), however with file objects remember you will need to use file_obj.get(ch) .
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello. I'm working on decompressing text in run length encoding in C++. I'm almost finished with the code but I'm confused about how to fix the whitespace.
Program requirements:
- Do not forget to close the file after you are done with it.
- You must use a while loop to handle the loop reading information from the file.
- You must use a for loop to handle the count/char output.
- Use the sample runs to ensure that all output is spelled/spaced correctly.
Hint:
You will need to make use of .get(ch) to "throw out" the irrelavent spaces. With stdin you would have used std::cin.get(ch), however with file objects remember you will need to use file_obj.get(ch) .

Transcribed Image Text:Sample Run 3 (simplestring.txtas input)
Enter filename to decompress: simplestring.dat
RRRRRRRRRRRRRGGGGGGGGGRRRRRRRRRRBBRRRRRRRRRRRGGGRGRGGGGBBBBBBRRRRRRRR
Sample Run 4 (cat.txt as input)
Enter filename to decompress: cat.txt
/// //_.-' .-/";
///_.-'
|/ (_..-' // (<
/ // // // `-. ‚_)' // /
Sample Run 5 (horse.txt as input)
Enter filename to decompress:
)
-/
horse.txt
|\ /\
__--/
---/ \/
\,,/_
(_
LA
/\
D
||
/___\
/ I
(D) 1
\_ / -1
/ \_ 0 0)
/
\__/
.
/ // /
'( ) ) // //
; `` / ///
/// / //
.:
I
||
|_/

Transcribed Image Text:567
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30 ✓
31
32 ✓
33
34
35
36
37
38
39
40
= 42 45 4 45 46
41
43
44
#include <fstream>
#include <iostream>
int main() {
}
char ch;
int count;
std::string filename;
// let's create an fstream variable
std::ifstream input_file;
// now read file name from user
std::cout << "Enter filename to decompress:
std::cin>> filename;
// open file to read data
input_file.open(filename);
// if file doesn't open
if (!input_file) {
std::cout << "File " << filename << "does not exist!\n";
}
// if file opens
else {
}
// read data until it reaches end of file
while (input_file) {
// input characters and numbers from file
input_file >> count;
input_file.get(ch);
}
// print character, count # of times
for (int i = 0; i < count; i++) {
std::cout << ch;
}
// close the file
input_file.close();
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
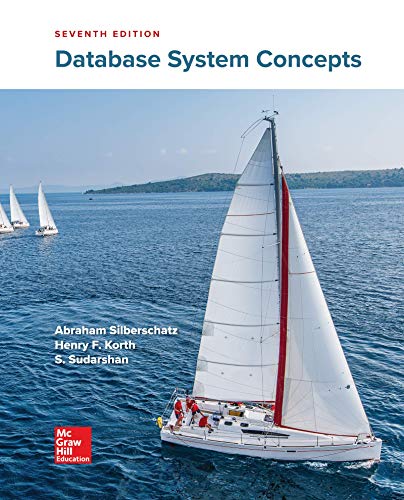
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
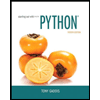
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
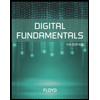
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
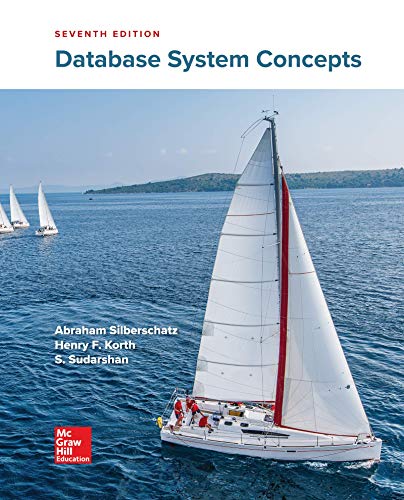
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
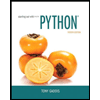
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
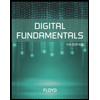
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
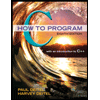
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
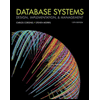
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
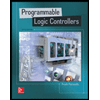
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education