Hello! I am having difficulties with the code above. I am not sure how to implement pthread_join. I am unsure of what to put in the second parameter. I put NULL, but twhen I do that the pthread_create gets uncolored(I am using Clion) and says that the value is never used. This is written in C++ #include #include int count = 0; // This function implements the code that is // executed by the thread. void* myFunction(void* arg){ int actual_arg = *((int*) arg); for(unsigned int i = 0; i < 10; ++i) { count++; std::cout << "Thread #" << actual_arg << " count = " << count << std::endl; } pthread_exit(NULL); } // This program creates one thread, waits for it // to finish its job and exits. int main(){ int rc; pthread_t the_thread; int arg = 1; // Data being passed to the thread // Create a new thread rc = pthread_create(&the_thread, NULL, myFunction, (void*) &arg); // Wait for the thread to do its job, then finish // TODO: Implement a call to pthread_join to ensure // that main() waits for the thread to finish // before continuing. pthread_join(the_thread,NULL); std::cout << "Thread #" << arg << " done!" << std::endl; std::cout << "Final count = " << count << std::endl; pthread_exit(NULL); return 0; }
Hello! I am having difficulties with the code above. I am not sure how to implement pthread_join. I am unsure of what to put in the second parameter. I put NULL, but twhen I do that the pthread_create gets uncolored(I am using Clion) and says that the value is never used. This is written in C++ #include #include int count = 0; // This function implements the code that is // executed by the thread. void* myFunction(void* arg){ int actual_arg = *((int*) arg); for(unsigned int i = 0; i < 10; ++i) { count++; std::cout << "Thread #" << actual_arg << " count = " << count << std::endl; } pthread_exit(NULL); } // This program creates one thread, waits for it // to finish its job and exits. int main(){ int rc; pthread_t the_thread; int arg = 1; // Data being passed to the thread // Create a new thread rc = pthread_create(&the_thread, NULL, myFunction, (void*) &arg); // Wait for the thread to do its job, then finish // TODO: Implement a call to pthread_join to ensure // that main() waits for the thread to finish // before continuing. pthread_join(the_thread,NULL); std::cout << "Thread #" << arg << " done!" << std::endl; std::cout << "Final count = " << count << std::endl; pthread_exit(NULL); return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello! I am having difficulties with the code above. I am not sure how to implement pthread_join. I am unsure of what to put in the second parameter. I put NULL, but twhen I do that the pthread_create gets uncolored(I am using Clion) and says that the value is never used. This is written in C++
#include <iostream>
#include <pthread.h>
int count = 0;
// This function implements the code that is
// executed by the thread.
void* myFunction(void* arg){
int actual_arg = *((int*) arg);
for(unsigned int i = 0; i < 10; ++i) {
count++;
std::cout << "Thread #" << actual_arg << " count = " << count << std::endl;
}
pthread_exit(NULL);
}
// This program creates one thread, waits for it
// to finish its job and exits.
int main(){
int rc;
pthread_t the_thread;
int arg = 1; // Data being passed to the thread
// Create a new thread
rc = pthread_create(&the_thread, NULL, myFunction, (void*) &arg);
// Wait for the thread to do its job, then finish
// TODO: Implement a call to pthread_join to ensure
// that main() waits for the thread to finish
// before continuing.
pthread_join(the_thread,NULL);
std::cout << "Thread #" << arg << " done!" << std::endl;
std::cout << "Final count = " << count << std::endl;
pthread_exit(NULL);
return 0;
}
#include <pthread.h>
int count = 0;
// This function implements the code that is
// executed by the thread.
void* myFunction(void* arg){
int actual_arg = *((int*) arg);
for(unsigned int i = 0; i < 10; ++i) {
count++;
std::cout << "Thread #" << actual_arg << " count = " << count << std::endl;
}
pthread_exit(NULL);
}
// This program creates one thread, waits for it
// to finish its job and exits.
int main(){
int rc;
pthread_t the_thread;
int arg = 1; // Data being passed to the thread
// Create a new thread
rc = pthread_create(&the_thread, NULL, myFunction, (void*) &arg);
// Wait for the thread to do its job, then finish
// TODO: Implement a call to pthread_join to ensure
// that main() waits for the thread to finish
// before continuing.
pthread_join(the_thread,NULL);
std::cout << "Thread #" << arg << " done!" << std::endl;
std::cout << "Final count = " << count << std::endl;
pthread_exit(NULL);
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
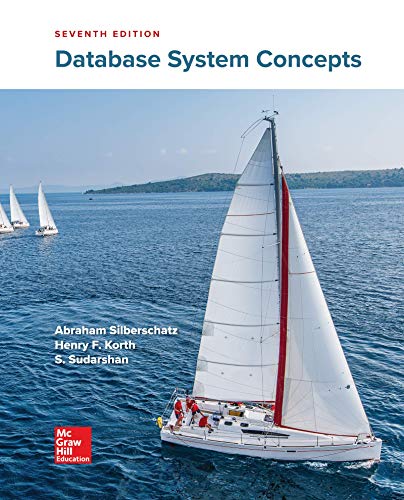
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
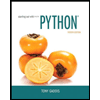
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
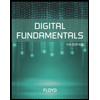
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
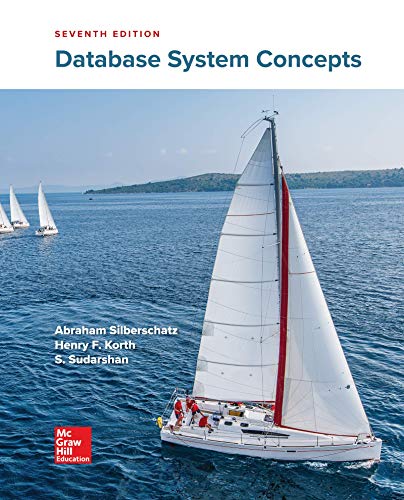
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
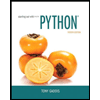
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
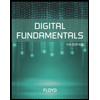
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
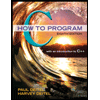
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
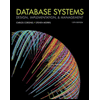
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
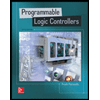
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education