Hello, C++ programming The source code starter file is included as an attachment The file starts with this string as the input to your encryption algorithm LLSNEEIRUIGNUL-CHXUADUPUGD-RTYI61IE-GDD5E0-607325-8576A598DFJ-EH8IKD7E037FJKE87--54625B6870-FJE941597---FHE7FHJ8KEN436-471369073C8767DFE-7KO8FLKE6895-8934 When properly decoded, the answer will describe how might you feel after you get this encyption scheme working Here is a list of steps needed to unencrypt your passcode, which is also found in the source code file: Create an empty stack The first 15 characters of the string get pushed into the stack in order (LLSNEEIRUIGNUL-) Create an empty queue The next 16 characters of the string get pushed into the queue in order (CHXUADUPUGD-RTYI) Create an empty deque For the remaining characters of the string in order (61IE-GDD5E0-607325-8576A598DFJ...) do the following conditionally: 1 = Pop the stack 2 = Pop the queue 3 = Pop the front of the deque 4 = Pop the back of the deque 5 = Move the top element of the stack to the back of queue 6 = Move the front element of the queue to the top of stack 7 = Move the top element of the stack to the front of deque 8 = Move the front element of the queue to the front of deque Anything else - Do nothing and move on NOTE: The term MOVE is not the same thing as COPY The deque will now have the secret phrase in it, listed from front to back, so print it out
Hello, C++ programming The source code starter file is included as an attachment The file starts with this string as the input to your encryption algorithm LLSNEEIRUIGNUL-CHXUADUPUGD-RTYI61IE-GDD5E0-607325-8576A598DFJ-EH8IKD7E037FJKE87--54625B6870-FJE941597---FHE7FHJ8KEN436-471369073C8767DFE-7KO8FLKE6895-8934 When properly decoded, the answer will describe how might you feel after you get this encyption scheme working Here is a list of steps needed to unencrypt your passcode, which is also found in the source code file: Create an empty stack The first 15 characters of the string get pushed into the stack in order (LLSNEEIRUIGNUL-) Create an empty queue The next 16 characters of the string get pushed into the queue in order (CHXUADUPUGD-RTYI) Create an empty deque For the remaining characters of the string in order (61IE-GDD5E0-607325-8576A598DFJ...) do the following conditionally: 1 = Pop the stack 2 = Pop the queue 3 = Pop the front of the deque 4 = Pop the back of the deque 5 = Move the top element of the stack to the back of queue 6 = Move the front element of the queue to the top of stack 7 = Move the top element of the stack to the front of deque 8 = Move the front element of the queue to the front of deque Anything else - Do nothing and move on NOTE: The term MOVE is not the same thing as COPY The deque will now have the secret phrase in it, listed from front to back, so print it out
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello, C++ programming
The source code starter file is included as an attachment
The file starts with this string as the input to your encryption
LLSNEEIRUIGNUL-CHXUADUPUGD-RTYI61IE-GDD5E0-607325-8576A598DFJ-EH8IKD7E037FJKE87--54625B6870-FJE941597---FHE7FHJ8KEN436-471369073C8767DFE-7KO8FLKE6895-8934
When properly decoded, the answer will describe how might you feel after you get this encyption scheme working
Here is a list of steps needed to unencrypt your passcode, which is also found in the source code file:
- Create an empty stack
- The first 15 characters of the string get pushed into the stack in order (LLSNEEIRUIGNUL-)
- Create an empty queue
- The next 16 characters of the string get pushed into the queue in order (CHXUADUPUGD-RTYI)
- Create an empty deque
- For the remaining characters of the string in order (61IE-GDD5E0-607325-8576A598DFJ...) do the following conditionally:
- 1 = Pop the stack
- 2 = Pop the queue
- 3 = Pop the front of the deque
- 4 = Pop the back of the deque
- 5 = Move the top element of the stack to the back of queue
- 6 = Move the front element of the queue to the top of stack
- 7 = Move the top element of the stack to the front of deque
- 8 = Move the front element of the queue to the front of deque
- Anything else - Do nothing and move on
- NOTE: The term MOVE is not the same thing as COPY
- The deque will now have the secret phrase in it, listed from front to back, so print it out

Transcribed Image Text:#include <iostream>
#include <deque>
#include <queue>
#include <stack>
#include <string>
int main()
{
}
// Here is your input
std::string passcode
// Create an empty stack
// The first 15 characters of the string get pushed into the stack in order
// Create an empty queue
// The next 16 characters of the string get pushed into the queue in order
// Create an empty deque
=
string
"LLSNEEIRUIGNUL-CHXUADUPUGD-RTY161IE-GDD5E0-607325-8576A598DFJ-EH8IKD7E037FJKE87--54625B6870-FJE941597---FHE7FHJ8KEN436-471369073C8767DFE-7KO8FLKE6895-8934";
// For the remaining characters of the string in order:
// 1
Pop the stack
// 2
Pop the queue
// 3
Pop the front of the deque
// 4 -
Pop the back of the deque
// 5 -
Move the top element of the stack to the back of queue
// 6 -
Move the front element of the queue to the top of stack
// 7 - Move the top element of the stack to the front of deque
// 8 - Move the front element of the queue to the front of deque
// Anything else - Do nothing and move on
std::cout << "How might you feel when you get this encryption scheme working properly?" << std::endl;
// The deque will have the secret phrase in it, listed from front to back, so print it out
// Cleanup
system("PAUSE");
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
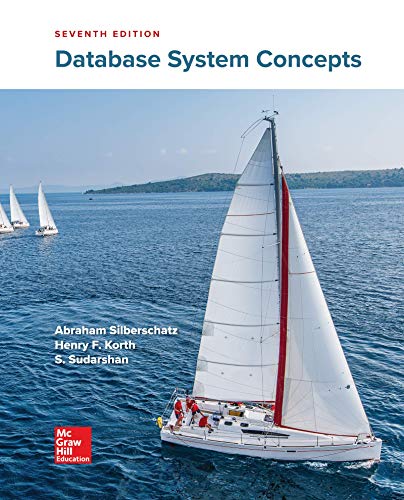
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
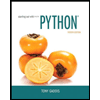
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
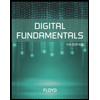
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
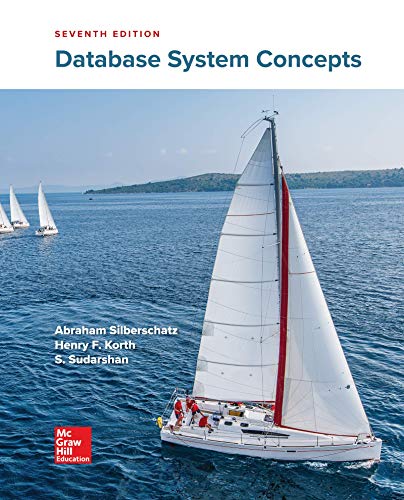
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
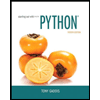
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
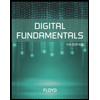
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
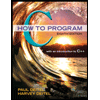
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
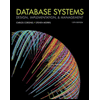
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
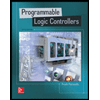
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education