Given following code and write comments for those code #include #include #include #include #define MAX_PASSWORD_LENGTH 128 #define MIN_PASSWORD_LENGTH 10 #define ALLOW_PASSPHRASE true #define MIN_PHRASE_LENGTH 20 #define OPTIONAL_TESTS_REQUIRED true #define MIN_OPTIONAL_TESTS_TO_PASS 4 bool isStrongPassword(char *password); void printTestResults(char *password, bool isPassphrase, int optionalTestsPassed); int main() { char passwords[][128] = { "password", "mypassword", "thisismypassword", "passssword", "This is my password phrase1", "Tinypw1", "Ireallydontlikehavingtomakeupnewpasswordsallthetime1", "Iloveyouxxxooo1", "Boom**********!", "IHATEPWORDS1!", "ihatepwords1!", "IHatePwords!", "IHatePwords", "my pass phrase does not need to pass tests", "short pass phrase", "x", "x1", "Zxcvbnmnas7", "Zxcvbnmnas~", "Zxcvbnmnas7~" }; for (int i = 0; i < sizeof(passwords) / sizeof(passwords[0]); ++i) { bool isPassphrase = strchr(passwords[i], ' ') && strlen(passwords[i]) >= MIN_PHRASE_LENGTH; int optionalTestsPassed = 0; if (OPTIONAL_TESTS_REQUIRED) { if (strpbrk(passwords[i], "abcdefghijklmnopqrstuvwxyz")) { optionalTestsPassed++; } } bool isPasswordStrong = isStrongPassword(passwords[i]) && (isPassphrase || optionalTestsPassed >= MIN_OPTIONAL_TESTS_TO_PASS); printTestResults(passwords[i], isPassphrase, optionalTestsPassed); printf("Strong? : %s\n", isPasswordStrong ? "true" : "false"); printf("Total optional tests passed: %d\n", optionalTestsPassed); printf("\n"); } return 0; } bool isStrongPassword(char *password) { if (strlen(password) < MIN_PASSWORD_LENGTH) { return false; } if (strlen(password) > MAX_PASSWORD_LENGTH) { return false; } for (int i = 0; i < strlen(password) - 2; ++i) { if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) { return false; } } return true; } void printTestResults(char *password, bool isPassphrase, int optionalTestsPassed) { printf("Proposed password: %s\n", password); printf("Failed Tests : "); if (strlen(password) < MIN_PASSWORD_LENGTH) { printf("[1] "); } if (strlen(password) > MAX_PASSWORD_LENGTH) { printf("[2] "); } for (int i = 0; i < strlen(password) - 2; ++i) { if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) { printf("[3] "); break; } } printf("\n"); printf("Passed Tests : "); if (strlen(password) >= MIN_PASSWORD_LENGTH && strlen(password) <= MAX_PASSWORD_LENGTH && !(strlen(password) > MIN_PHRASE_LENGTH && isPassphrase)) { printf("[1-3] "); } if (optionalTestsPassed >= MIN_OPTIONAL_TESTS_TO_PASS || !OPTIONAL_TESTS_REQUIRED) { printf("[4-7] "); } printf("\n"); printf("Required Test Errors : [\n"); if (strlen(password) < MIN_PASSWORD_LENGTH) { printf("'The password must be at least %d characters long.'\n", MIN_PASSWORD_LENGTH); } if (strlen(password) > MAX_PASSWORD_LENGTH) { printf("'The password must be fewer than %d characters.'\n", MAX_PASSWORD_LENGTH); } for (int i = 0; i < strlen(password) - 2; ++i) { if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) { printf("'The password may not contain a sequence of three or more repeated characters.'\n"); break; } } printf("]\n"); printf("Optional Test Errors : [\n"); if (OPTIONAL_TESTS_REQUIRED) { if (!(strpbrk(password, "abcdefghijklmnopqrstuvwxyz"))) { printf("'The password must contain at least one lowercase letter.'\n"); } } printf("]\n"); printf("Is a Pass phrase : %s\n", isPassphrase ? "true" : "false"); }
Given following code and write comments for those code
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
#include <ctype.h>
#define MAX_PASSWORD_LENGTH 128
#define MIN_PASSWORD_LENGTH 10
#define ALLOW_PASSPHRASE true
#define MIN_PHRASE_LENGTH 20
#define OPTIONAL_TESTS_REQUIRED true
#define MIN_OPTIONAL_TESTS_TO_PASS 4
bool isStrongPassword(char *password);
void printTestResults(char *password, bool isPassphrase, int optionalTestsPassed);
int main() {
char passwords[][128] = {
"password",
"mypassword",
"thisismypassword",
"passssword",
"This is my password phrase1",
"Tinypw1",
"Ireallydontlikehavingtomakeupnewpasswordsallthetime1",
"Iloveyouxxxooo1",
"Boom**********!",
"IHATEPWORDS1!",
"ihatepwords1!",
"IHatePwords!",
"IHatePwords",
"my pass phrase does not need to pass tests",
"short pass phrase",
"x",
"x1",
"Zxcvbnmnas7",
"Zxcvbnmnas~",
"Zxcvbnmnas7~"
};
for (int i = 0; i < sizeof(passwords) / sizeof(passwords[0]); ++i) {
bool isPassphrase = strchr(passwords[i], ' ') && strlen(passwords[i]) >= MIN_PHRASE_LENGTH;
int optionalTestsPassed = 0;
if (OPTIONAL_TESTS_REQUIRED) {
if (strpbrk(passwords[i], "abcdefghijklmnopqrstuvwxyz")) {
optionalTestsPassed++;
}
}
bool isPasswordStrong = isStrongPassword(passwords[i]) &&
(isPassphrase || optionalTestsPassed >= MIN_OPTIONAL_TESTS_TO_PASS);
printTestResults(passwords[i], isPassphrase, optionalTestsPassed);
printf("Strong? : %s\n", isPasswordStrong ? "true" : "false");
printf("Total optional tests passed: %d\n", optionalTestsPassed);
printf("\n");
}
return 0;
}
bool isStrongPassword(char *password) {
if (strlen(password) < MIN_PASSWORD_LENGTH) {
return false;
}
if (strlen(password) > MAX_PASSWORD_LENGTH) {
return false;
}
for (int i = 0; i < strlen(password) - 2; ++i) {
if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) {
return false;
}
}
return true;
}
void printTestResults(char *password, bool isPassphrase, int optionalTestsPassed) {
printf("Proposed password: %s\n", password);
printf("Failed Tests : ");
if (strlen(password) < MIN_PASSWORD_LENGTH) {
printf("[1] ");
}
if (strlen(password) > MAX_PASSWORD_LENGTH) {
printf("[2] ");
}
for (int i = 0; i < strlen(password) - 2; ++i) {
if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) {
printf("[3] ");
break;
}
}
printf("\n");
printf("Passed Tests : ");
if (strlen(password) >= MIN_PASSWORD_LENGTH && strlen(password) <= MAX_PASSWORD_LENGTH &&
!(strlen(password) > MIN_PHRASE_LENGTH && isPassphrase)) {
printf("[1-3] ");
}
if (optionalTestsPassed >= MIN_OPTIONAL_TESTS_TO_PASS || !OPTIONAL_TESTS_REQUIRED) {
printf("[4-7] ");
}
printf("\n");
printf("Required Test Errors : [\n");
if (strlen(password) < MIN_PASSWORD_LENGTH) {
printf("'The password must be at least %d characters long.'\n", MIN_PASSWORD_LENGTH);
}
if (strlen(password) > MAX_PASSWORD_LENGTH) {
printf("'The password must be fewer than %d characters.'\n", MAX_PASSWORD_LENGTH);
}
for (int i = 0; i < strlen(password) - 2; ++i) {
if (password[i] == password[i + 1] && password[i + 1] == password[i + 2]) {
printf("'The password may not contain a sequence of three or more repeated characters.'\n");
break;
}
}
printf("]\n");
printf("Optional Test Errors : [\n");
if (OPTIONAL_TESTS_REQUIRED) {
if (!(strpbrk(password, "abcdefghijklmnopqrstuvwxyz"))) {
printf("'The password must contain at least one lowercase letter.'\n");
}
}
printf("]\n");
printf("Is a Pass phrase : %s\n", isPassphrase ? "true" : "false");
}

Step by step
Solved in 4 steps with 5 images

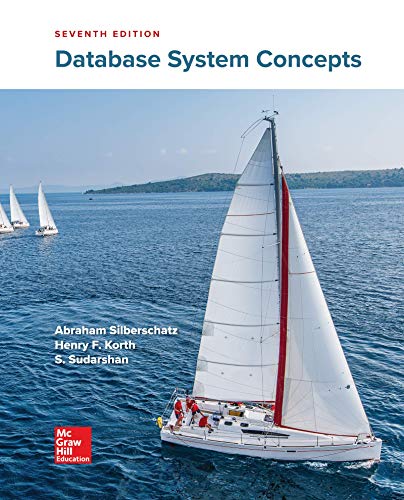
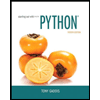
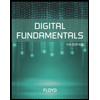
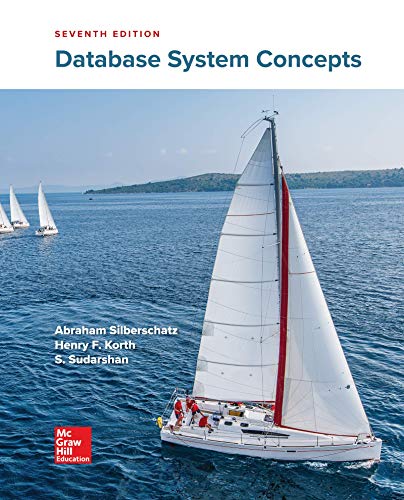
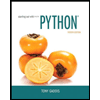
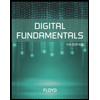
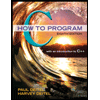
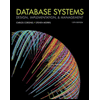
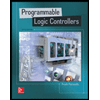