# ===================== Provided Helper Functions ===================== def transform_string(s: str) -> str: """Return a new string based on s in which all letters have been converted to uppercase and punctuation characters have been stripped from both ends. Inner punctuation is left untouched. >>> transform_string('Birthday!!!') 'BIRTHDAY' >>> transform_string('"Quoted?"') 'QUOTED' >>> transform_string('To be? Or not to be?') 'TO BE? OR NOT TO BE' """ punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r""" result = s.upper().strip(punctuation) return result def is_vowel_phoneme(phoneme: str) -> bool: """Return True if and only if phoneme is a vowel phoneme. That is, whether phoneme ends in a 0, 1, or 2. Precondition: len(phoneme) > 0 and phoneme.isupper() >>> is_vowel_phoneme('AE0') True >>> is_vowel_phoneme('DH') False >>> is_vowel_phoneme('IH2') True """ return phoneme[-1] in '012' The functions required def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int], word_to_phonemes: PRONUNCIATION_DICT) -> bool: """Return True if and only if the lines from poem_lines with index in lines_to_check all rhyme, according to word_to_phonemes. Precondition: lines_to_check != [] >>> poem_lines = ['The mouse', 'in my house', 'electric.'] >>> lines_to_check = [0, 1] >>> word_to_phonemes = {'THE': ('DH', 'AH0'), ... 'MOUSE': ('M', 'AW1', 'S'), ... 'IN': ('IH0', 'N'), ... 'MY': ('M', 'AY1'), ... 'HOUSE': ('HH', 'AW1', 'S'), ... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K', ... 'T', 'R', 'IH0', 'K')} >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True >>> lines_to_check = [0, 1, 2] >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) False >>> lines_to_check = [2] >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True """ def get_symbol_to_lines(rhyme_scheme: Tuple[str]) -> Dict[str, List[int]]: """Return a dictionary where each key is an item in rhyme_scheme and its corresponding value is a list of the indexes in rhyme_scheme where the item appears. >>> result = get_symbol_to_lines(('A', 'A', 'B', 'B', 'A')) >>> expected = {'A': [0, 1, 4], 'B': [2, 3]} >>> expected == result True >>> result = get_symbol_to_lines(('*', '*', '*', '*', '*')) >>> expected = {'*': [0, 1, 2, 3, 4]} >>> expected == result True """ def check_rhyme_scheme(poem_lines: POEM, description: POETRY_FORM_DESCRIPTION, word_to_phonemes: PRONUNCIATION_DICT) \ -> List[List[POEM_LINE]]: """Return a list of lists of lines from poem_lines that do NOT rhyme with each other as specified by the poetry form description, according to the pronunciation dictionary word_to_phonemes. If all lines rhyme as they should, return the empty list. The lines is each inner list should be in the same order as they appear in poem_lines. Precondition: len(poem_lines) == len(description[1]) >>> poem_lines = ['The first line leads off,', ... 'With a gap before the next.', 'Then the poem ends.'] >>> description = ((5, 7, 5), ('A', 'B', 'A')) >>> word_to_phonemes = {'NEXT': ('N', 'EH1', 'K', 'S', 'T'), ... 'GAP': ('G', 'AE1', 'P'), ... 'BEFORE': ('B', 'IH0', 'F', 'AO1', 'R'), ... 'LEADS': ('L', 'IY1', 'D', 'Z'), ... 'WITH': ('W', 'IH1', 'DH'), ... 'LINE': ('L', 'AY1', 'N'), ... 'THEN': ('DH', 'EH1', 'N'), ... 'THE': ('DH', 'AH0'), ... 'A': ('AH0'), ... 'FIRST': ('F', 'ER1', 'S', 'T'), ... 'ENDS': ('EH1', 'N', 'D', 'Z'), ... 'POEM': ('P', 'OW1', 'AH0', 'M'), ... 'OFF': ('AO1', 'F')} >>> bad_lines = check_rhyme_scheme(poem_lines, description, ... word_to_phonemes) >>> bad_lines.sort() >>> bad_lines [['The first line leads off,', 'Then the poem ends.']] """
# ===================== Provided Helper Functions =====================
def transform_string(s: str) -> str:
"""Return a new string based on s in which all letters have been
converted to uppercase and punctuation characters have been stripped
from both ends. Inner punctuation is left untouched.
>>> transform_string('Birthday!!!')
'BIRTHDAY'
>>> transform_string('"Quoted?"')
'QUOTED'
>>> transform_string('To be? Or not to be?')
'TO BE? OR NOT TO BE'
"""
punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r"""
result = s.upper().strip(punctuation)
return result
def is_vowel_phoneme(phoneme: str) -> bool:
"""Return True if and only if phoneme is a vowel phoneme. That is, whether
phoneme ends in a 0, 1, or 2.
Precondition: len(phoneme) > 0 and phoneme.isupper()
>>> is_vowel_phoneme('AE0')
True
>>> is_vowel_phoneme('DH')
False
>>> is_vowel_phoneme('IH2')
True
"""
return phoneme[-1] in '012'
The functions required
def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int],
word_to_phonemes: PRONUNCIATION_DICT) -> bool:
"""Return True if and only if the lines from poem_lines with index in
lines_to_check all rhyme, according to word_to_phonemes.
Precondition: lines_to_check != []
>>> poem_lines = ['The mouse', 'in my house', 'electric.']
>>> lines_to_check = [0, 1]
>>> word_to_phonemes = {'THE': ('DH', 'AH0'),
... 'MOUSE': ('M', 'AW1', 'S'),
... 'IN': ('IH0', 'N'),
... 'MY': ('M', 'AY1'),
... 'HOUSE': ('HH', 'AW1', 'S'),
... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K',
... 'T', 'R', 'IH0', 'K')}
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
True
>>> lines_to_check = [0, 1, 2]
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
False
>>> lines_to_check = [2]
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
True
"""
def get_symbol_to_lines(rhyme_scheme: Tuple[str]) -> Dict[str, List[int]]:
"""Return a dictionary where each key is an item in rhyme_scheme and
its corresponding value is a list of the indexes in rhyme_scheme where
the item appears.
>>> result = get_symbol_to_lines(('A', 'A', 'B', 'B', 'A'))
>>> expected = {'A': [0, 1, 4], 'B': [2, 3]}
>>> expected == result
True
>>> result = get_symbol_to_lines(('*', '*', '*', '*', '*'))
>>> expected = {'*': [0, 1, 2, 3, 4]}
>>> expected == result
True
"""
def check_rhyme_scheme(poem_lines: POEM,
description: POETRY_FORM_DESCRIPTION,
word_to_phonemes: PRONUNCIATION_DICT) \
-> List[List[POEM_LINE]]:
"""Return a list of lists of lines from poem_lines that do NOT rhyme with
each other as specified by the poetry form description, according to the
pronunciation dictionary word_to_phonemes. If all lines rhyme as they
should, return the empty list. The lines is each inner list should be
in the same order as they appear in poem_lines.
Precondition: len(poem_lines) == len(description[1])
>>> poem_lines = ['The first line leads off,',
... 'With a gap before the next.', 'Then the poem ends.']
>>> description = ((5, 7, 5), ('A', 'B', 'A'))
>>> word_to_phonemes = {'NEXT': ('N', 'EH1', 'K', 'S', 'T'),
... 'GAP': ('G', 'AE1', 'P'),
... 'BEFORE': ('B', 'IH0', 'F', 'AO1', 'R'),
... 'LEADS': ('L', 'IY1', 'D', 'Z'),
... 'WITH': ('W', 'IH1', 'DH'),
... 'LINE': ('L', 'AY1', 'N'),
... 'THEN': ('DH', 'EH1', 'N'),
... 'THE': ('DH', 'AH0'),
... 'A': ('AH0'),
... 'FIRST': ('F', 'ER1', 'S', 'T'),
... 'ENDS': ('EH1', 'N', 'D', 'Z'),
... 'POEM': ('P', 'OW1', 'AH0', 'M'),
... 'OFF': ('AO1', 'F')}
>>> bad_lines = check_rhyme_scheme(poem_lines, description,
... word_to_phonemes)
>>> bad_lines.sort()
>>> bad_lines
[['The first line leads off,', 'Then the poem ends.']]
"""

Step by step
Solved in 3 steps with 4 images

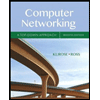
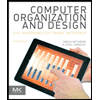
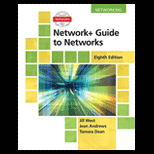
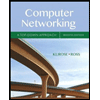
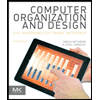
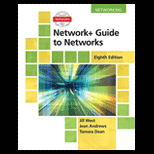
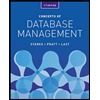
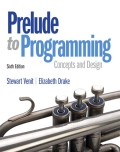
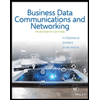