have this c++ code and it runs but receive a style check error: Stylecheck error on robot.cc file warning: member initializer for 'x_' is redundant [modernize-use-default-member-init] : x_(0), ^~~~~ warning: member initializer for 'y_' is redundant [modernize-use-default-member-init] y_(0), ^~~~~ warning: member initializer for 'mod_' is redundant [modernize-use-default-member-init] mod_(0), warning: initializer for member 'icon1_' is redundant [readability-redundant-member-init] icon1_(), warning: initializer for member 'icon2_' is redundant [readability-redundant-member-init] icon2_() {} main.cc file #include "robotutils/robotclicklistener.h" // // You do not need to edit this file. // // Helper function to create robot*.bmp. Feel free to make your own // icons and use this for inspiration. /* void CreateRobotIcon() { graphics::Image image(31, 31); // Arms image.DrawLine(0, 10, 10, 15, 109, 131, 161, 6); image.DrawLine(30, 10, 10, 15, 109, 131, 161, 6); // Legs image.DrawLine(10, 15, 10, 30, 109, 131, 161, 6); image.DrawLine(20, 15, 20, 30, 109, 131, 161, 6); // Body image.DrawRectangle(5, 0, 20, 22, 130, 151, 179); // Eyes image.DrawCircle(10, 8, 2, 255, 255, 255); image.DrawCircle(20, 8, 2, 255, 255, 255); image.DrawCircle(9, 8, 2, 62, 66, 71); image.DrawCircle(19, 8, 2, 62, 66, 71); image.SaveImageBmp("robot.bmp"); } */ int main() { RobotClickListener listener{}; listener.Start(); return 0; } robot.cc file #include "robot.h" #include #include "cpputils/graphics/image.h" // ========================= YOUR CODE HERE ========================= // This implementation file (robot.cc) should hold the // implementation of member functions declared in the header (robot.h). // // Implement the following member functions, declared in robot.h: // 1. Robot constructor // 2. SetPosition // 3. GetX // 4. GetY // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Robot class. // =================================================================== Robot::Robot(const std::string& filename1, const std::string& filename2) :x_(0), y_(0), mod_(0), filename1_(filename1), filename2_(filename2), icon1_(), icon2_() {} // SetPosition implementation void Robot::SetPosition(int x, int y) { x_ = x; y_ = y; } // GetX implementation int Robot::GetX() const { return x_; } // GetY implementation int Robot::GetY() const { return y_; } // You don't need to modify these. These are helper functions // used to load the robot icons and draw them on the screen. void Robot::Draw(graphics::Image& image) { // Load the image into the icon if needed. if (icon1_.GetWidth() <= 0) { icon1_.Load(filename1_); } if (icon2_.GetWidth() <= 0) { icon2_.Load(filename2_); } mod_ = (mod_ + 1) % 2; if (mod_ == 1) { DrawIconOnImage(icon1_, image); } else { DrawIconOnImage(icon2_, image); } } void Robot::DrawIconOnImage(graphics::Image& icon, graphics::Image& image) const { int width = icon.GetWidth(); int height = icon.GetHeight(); for (int i = 0; i < width; i++) { for (int j = 0; j < height; j++) { int x = x_ + i - width / 2; int y = y_ + j - height / 2; if (y >= 0 && x >= 0 && x < image.GetWidth() && y < image.GetHeight()) { image.SetColor(x, y, icon.GetColor(i, j)); } } } } robot.h file #include #include "cpputils/graphics/image.h" class Robot { public: // You don't need to change this file, but you will be // implementing some of these member functions in robot.cc. Robot(const std::string& filename1, const std::string& filename2); void SetPosition(int x, int y); int GetX() const; int GetY() const; void Draw(graphics::Image& image); private: void DrawIconOnImage(graphics::Image& icon, graphics::Image& image) const; std::string filename1_; std::string filename2_; graphics::Image icon1_; graphics::Image icon2_; graphics::Color color_; int x_ = 0; int y_ = 0; int mod_ = 0; };
have this c++ code and it runs but receive a style check error: Stylecheck error on robot.cc file
warning: member initializer for 'x_' is redundant [modernize-use-default-member-init]
: x_(0),
^~~~~
warning: member initializer for 'y_' is redundant [modernize-use-default-member-init]
y_(0),
^~~~~
warning: member initializer for 'mod_' is redundant [modernize-use-default-member-init]
mod_(0),
warning: initializer for member 'icon1_' is redundant [readability-redundant-member-init]
icon1_(),
warning: initializer for member 'icon2_' is redundant [readability-redundant-member-init]
icon2_() {}
main.cc file
#include "robotutils/robotclicklistener.h"
//
// You do not need to edit this file.
//
// Helper function to create robot*.bmp. Feel free to make your own
// icons and use this for inspiration.
/*
void CreateRobotIcon() {
graphics::Image image(31, 31);
// Arms
image.DrawLine(0, 10, 10, 15, 109, 131, 161, 6);
image.DrawLine(30, 10, 10, 15, 109, 131, 161, 6);
// Legs
image.DrawLine(10, 15, 10, 30, 109, 131, 161, 6);
image.DrawLine(20, 15, 20, 30, 109, 131, 161, 6);
// Body
image.DrawRectangle(5, 0, 20, 22, 130, 151, 179);
// Eyes
image.DrawCircle(10, 8, 2, 255, 255, 255);
image.DrawCircle(20, 8, 2, 255, 255, 255);
image.DrawCircle(9, 8, 2, 62, 66, 71);
image.DrawCircle(19, 8, 2, 62, 66, 71);
image.SaveImageBmp("robot.bmp");
}
*/
int main() {
RobotClickListener listener{};
listener.Start();
return 0;
}
robot.cc file
#include "robot.h"
#include <string>
#include "cpputils/graphics/image.h"
// ========================= YOUR CODE HERE =========================
// This implementation file (robot.cc) should hold the
// implementation of member functions declared in the header (robot.h).
//
// Implement the following member functions, declared in robot.h:
// 1. Robot constructor
// 2. SetPosition
// 3. GetX
// 4. GetY
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Robot class.
// ===================================================================
Robot::Robot(const std::string& filename1, const std::string& filename2)
:x_(0),
y_(0),
mod_(0),
filename1_(filename1),
filename2_(filename2),
icon1_(),
icon2_() {}
// SetPosition implementation
void Robot::SetPosition(int x, int y) {
x_ = x;
y_ = y;
}
// GetX implementation
int Robot::GetX() const {
return x_;
}
// GetY implementation
int Robot::GetY() const {
return y_;
}
// You don't need to modify these. These are helper functions
// used to load the robot icons and draw them on the screen.
void Robot::Draw(graphics::Image& image) {
// Load the image into the icon if needed.
if (icon1_.GetWidth() <= 0) {
icon1_.Load(filename1_);
}
if (icon2_.GetWidth() <= 0) {
icon2_.Load(filename2_);
}
mod_ = (mod_ + 1) % 2;
if (mod_ == 1) {
DrawIconOnImage(icon1_, image);
} else {
DrawIconOnImage(icon2_, image);
}
}
void Robot::DrawIconOnImage(graphics::Image& icon,
graphics::Image& image) const {
int width = icon.GetWidth();
int height = icon.GetHeight();
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
int x = x_ + i - width / 2;
int y = y_ + j - height / 2;
if (y >= 0 && x >= 0 && x < image.GetWidth() && y < image.GetHeight()) {
image.SetColor(x, y, icon.GetColor(i, j));
}
}
}
}
robot.h file
#include <string>
#include "cpputils/graphics/image.h"
class Robot {
public:
// You don't need to change this file, but you will be
// implementing some of these member functions in robot.cc.
Robot(const std::string& filename1, const std::string& filename2);
void SetPosition(int x, int y);
int GetX() const;
int GetY() const;
void Draw(graphics::Image& image);
private:
void DrawIconOnImage(graphics::Image& icon, graphics::Image& image) const;
std::string filename1_;
std::string filename2_;
graphics::Image icon1_;
graphics::Image icon2_;
graphics::Color color_;
int x_ = 0;
int y_ = 0;
int mod_ = 0;
};

Step by step
Solved in 2 steps

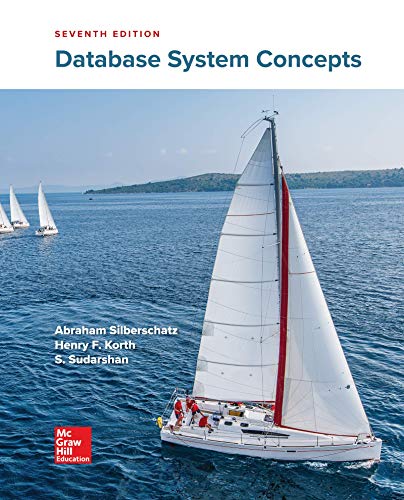
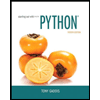
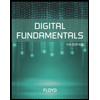
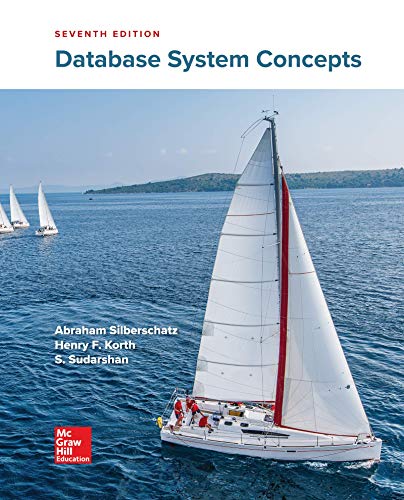
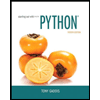
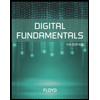
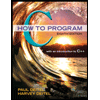
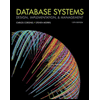
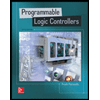