#This is the class class ConvertCSVToJSON: def __init__(self, headings, ID, linesFromFile, inputFileName, outputFileName="Project7Output.txt"): self.headings=headings # Headings (row 1 of the file) self.ID=ID self.linesFromFile=linesFromFile # rows2-end of file (EOF) self.outputFileName=outputFileName self.inputFileName=inputFileName def createKeyValuePair(self, key, value): # Think about parameters and how the code will work result="\""+key+"\""+":"+value return result #create a line function/method in class to have access to headings and file. def createLine(self, ID, line): #splits the line into different components lineComponets=line.split(",") dictionary={}#creats a dictionary for the componets for i in range(len(self.heading)): dictionary[self.headings[i]]=lineComponents[i] #adds ID as a keyvalue pair dictionary["ID"]=str(ID) #should convert to json jsonLine="{" + ",".join([self.createKeyValuePair(key, "\"" + value + "\"") for key, value in dictionary. items()]) + "}" return jsonLine # Main function def main(): headings=["last Name", "First Name"] ID=1 inputFileName="Input1.txt" linesFromFile=["Sue", "Lou"] #objects used to call methods example1=ConvertCSVToJSON(headings, ID, linesFromFile, inputFileName) example2=ConvertCSVToJSON(headings, ID, linesFromFile, inputFileName, "Output.txt") returnedValue=example1.createKeyValuePair("Last Name", "Sue") print(returnedValue) #calls my createline method example1.headings=("First Name", "Last Name", "Age") example1.linesFromFile=("Sam,Jackson,24", "Tanya,Louis,52") for line in example1.linesFromFile: jsonLine = example1.createLine(line) print(jsonLine) if(__name__=="__main__"): main() I need to convert this from cvs to json without importing json
I'm not sure how to fix my error
import csv
#This is the class
class ConvertCSVToJSON:
def __init__(self, headings, ID, linesFromFile, inputFileName, outputFileName="Project7Output.txt"):
self.headings=headings # Headings (row 1 of the file)
self.ID=ID
self.linesFromFile=linesFromFile # rows2-end of file (EOF)
self.outputFileName=outputFileName
self.inputFileName=inputFileName
def createKeyValuePair(self, key, value): # Think about parameters and how the code will work
result="\""+key+"\""+":"+value
return result
#create a line function/method in class to have access to headings and file.
def createLine(self, ID, line): #splits the line into different components
lineComponets=line.split(",")
dictionary={}#creats a dictionary for the componets
for i in range(len(self.heading)):
dictionary[self.headings[i]]=lineComponents[i]
#adds ID as a keyvalue pair
dictionary["ID"]=str(ID)
#should convert to json
jsonLine="{" + ",".join([self.createKeyValuePair(key, "\"" + value + "\"") for key, value in dictionary. items()]) + "}"
return jsonLine
# Main function
def main():
headings=["last Name", "First Name"]
ID=1
inputFileName="Input1.txt"
linesFromFile=["Sue", "Lou"]
#objects used to call methods
example1=ConvertCSVToJSON(headings, ID, linesFromFile, inputFileName)
example2=ConvertCSVToJSON(headings, ID, linesFromFile, inputFileName, "Output.txt")
returnedValue=example1.createKeyValuePair("Last Name", "Sue")
print(returnedValue)
#calls my createline method
example1.headings=("First Name", "Last Name", "Age")
example1.linesFromFile=("Sam,Jackson,24", "Tanya,Louis,52")
for line in example1.linesFromFile:
jsonLine = example1.createLine(line)
print(jsonLine)
if(__name__=="__main__"):
main()
I need to convert this from cvs to json without importing json

Step by step
Solved in 3 steps

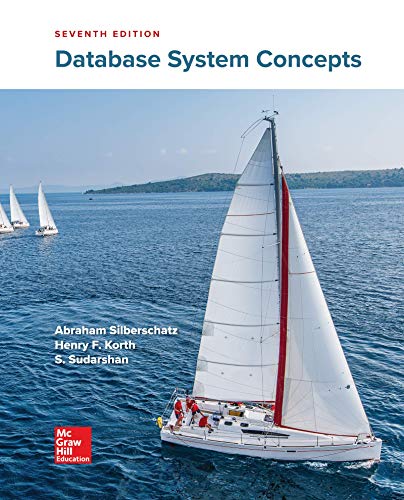
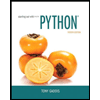
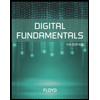
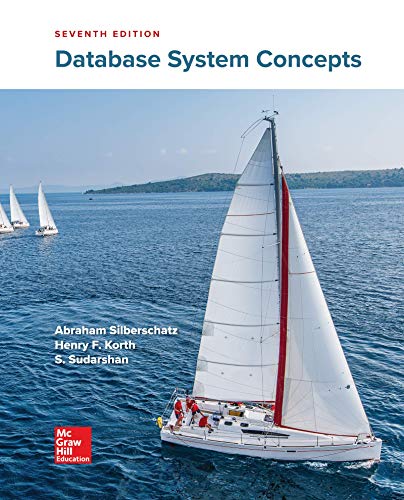
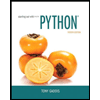
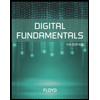
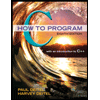
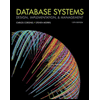
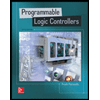