Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status The SimpleCar class is found in the file SimpleCar.java. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles // Simulates a simple car with operations to drive and check the odometer. public class SimpleCar { // Number of miles driven private int miles; public SimpleCar(){ miles = 0; } public void drive(int dist){ miles = miles + dist; } public void reverse(int dist){ miles = miles - dist; } public int getOdometer(){ return miles; } public void honkHorn(){ System.out.println("beep beep"); } public void report(){ System.out.println("Car has driven: " + miles + " miles"); } }
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations:
- Drives input number of miles forward
- Drives input number of miles in reverse
- Honks the horn
- Reports car status
The SimpleCar class is found in the file SimpleCar.java.
Ex: If the input is:
100 4the output is:
beep beep Car has driven: 96 miles// Simulates a simple car with operations to drive and check the odometer.
public class SimpleCar {
// Number of miles driven
private int miles;
public SimpleCar(){
miles = 0;
}
public void drive(int dist){
miles = miles + dist;
}
public void reverse(int dist){
miles = miles - dist;
}
public int getOdometer(){
return miles;
}
public void honkHorn(){
System.out.println("beep beep");
}
public void report(){
System.out.println("Car has driven: " + miles + " miles");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

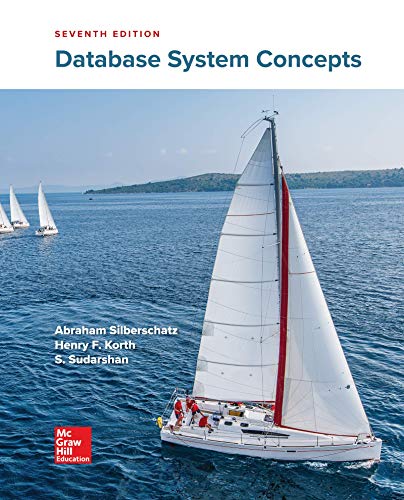
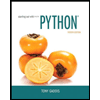
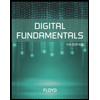
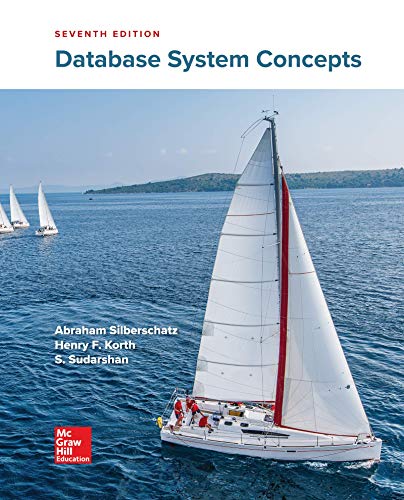
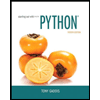
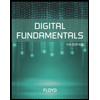
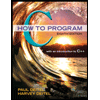
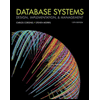
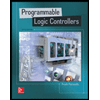