Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status Review the definition of "SimpleCar.cpp" by clicking on the orange arrow. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles --- simplecar.cpp -- #include "SimpleCar.h" #include #include using namespace std; SimpleCar::SimpleCar() { miles = 0; } void SimpleCar::Drive(int dist) { miles = miles + dist; } void SimpleCar::Reverse(int dist) { miles = miles - dist; } int SimpleCar::GetOdometer() { return miles; } void SimpleCar::HonkHorn() { cout << "beep beep" << endl; } void SimpleCar::Report() { cout << "Car has driven: " << miles << " miles" << endl; } -- main.cpp -- #include "SimpleCar.h" #include #include using namespace std; int main() { /* Type your code here */ return 0; } -- simplecar.h -- #ifndef SIMPLECAR_H_ #define SIMPLECAR_H_ #include using namespace std; class SimpleCar { public: SimpleCar(); void Drive(int dist); void Reverse(int dist); int GetOdometer(); void HonkHorn(); void Report(); private: int miles; }; #endif /* SIMPLECAR_H_ */
23.8 LAB: Simple car
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations:
- Drives input number of miles forward
- Drives input number of miles in reverse
- Honks the horn
- Reports car status
Review the definition of "SimpleCar.cpp" by clicking on the orange arrow.
Ex: If the input is:
100 4the output is:
beep beep Car has driven: 96 miles#include "SimpleCar.h"
#include <iostream>
#include <string>
using namespace std;
SimpleCar::SimpleCar() {
miles = 0;
}
void SimpleCar::Drive(int dist) {
miles = miles + dist;
}
void SimpleCar::Reverse(int dist) {
miles = miles - dist;
}
int SimpleCar::GetOdometer() {
return miles;
}
void SimpleCar::HonkHorn() {
cout << "beep beep" << endl;
}
void SimpleCar::Report() {
cout << "Car has driven: " << miles << " miles" << endl;
}
--
main.cpp
--
#include "SimpleCar.h"
#include <iostream>
#include <vector>
using namespace std;
int main() {
/* Type your code here */
return 0;
}
--
simplecar.h
--
#ifndef SIMPLECAR_H_
#define SIMPLECAR_H_
#include <iostream>
using namespace std;
class SimpleCar {
public:
SimpleCar();
void Drive(int dist);
void Reverse(int dist);
int GetOdometer();
void HonkHorn();
void Report();
private:
int miles;
};
#endif /* SIMPLECAR_H_ */

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

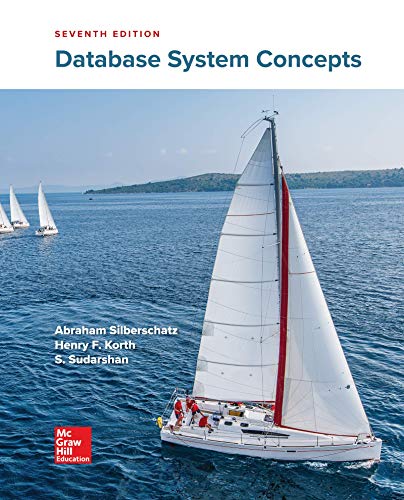
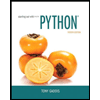
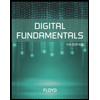
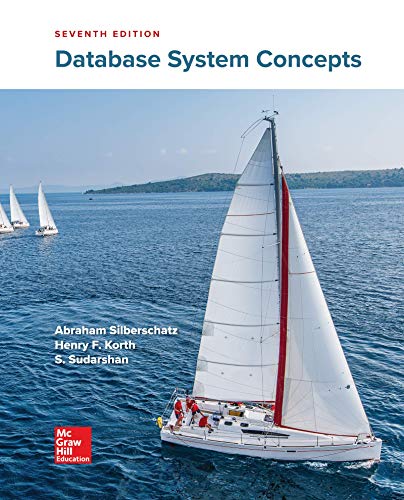
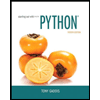
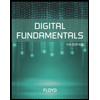
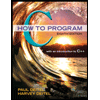
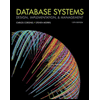
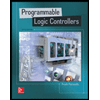