VendingMachine
Given two integers as user inputs that represent the number of drinks to buy and the number of bottles to restock, create a VendingMachine object that performs the following operations:
- Purchases input number of drinks
- Restocks input number of bottles
- Reports inventory
Review the definition of "VendingMachine.cpp" by clicking on the orange arrow. A VendingMachine's initial inventory is 20 drinks.
Ex: If the input is:
5 2
the output is:
Inventory: 17 bottles
VendingMachine.h
#ifndef VENDINGMACHINE_H_
#define VENDINGMACHINE_H_
#include <iostream>
using namespace std;
class VendingMachine {
public:
VendingMachine();
void Purchase(int amount);
int GetInventory();
void Restock(int amount);
void Report();
private:
int bottles;
};
#endif /* VENDINGMACHINE_H_ */
VendingMachine.cpp
#include <iostream>
#include <string>
#include "VendingMachine.h"
using namespace std;
VendingMachine::VendingMachine() {
bottles = 20;
}
void VendingMachine::Purchase(int amount) {
bottles = bottles - amount;
}
int VendingMachine::GetInventory() {
return bottles;
}
void VendingMachine::Restock(int amount) {
bottles = bottles + amount;
}
void VendingMachine::Report() {
cout << "Inventory: " << bottles << " bottles" << endl;
}
main.cpp
#include <iostream>
#include "VendingMachine.h"
using namespace std;
int main() {
/* Type your code here */
}
HELP ME TYPE CODE HERE C++ THANK YOU

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

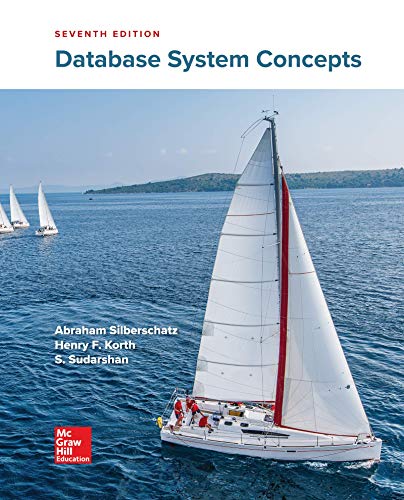
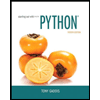
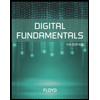
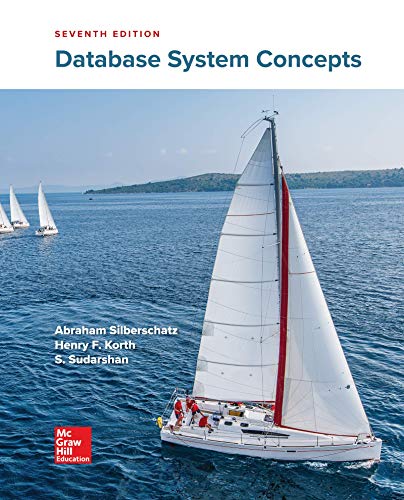
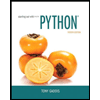
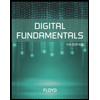
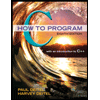
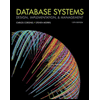
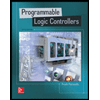