Declare and initialize 2 Animal objects with the following properties: A animal named Zirly the Zebra with the zebraZirlyBehaviors created above and a weight of 812.3 pounds A animal named Henry the Hawk with the henryTheHawkBehaviors created above and a weight of 35.5 pounds. Then, store the 2 animal objects in an array named animalList Choose one of the following from the above choices (A, B, C or D within the code).
Declare and initialize 2 Animal objects with the following properties:
- A animal named Zirly the Zebra with the zebraZirlyBehaviors created above and a weight of 812.3 pounds
- A animal named Henry the Hawk with the henryTheHawkBehaviors created above and a weight of 35.5 pounds.
Then, store the 2 animal objects in an array named animalList
Choose one of the following from the above choices (A, B, C or D within the code).
// Assume the following constructor exists within the Animal class:
public Animal(String animalName,
double weight,
Behavior[] behaviorList) {
this.animalName = animalName;
this.weight = weight;
this.behaviorList = behaviorList;
}
//A
Animal zebraZirly = new Animal(812.3, "Zirly the Zebra", zebraZirlyBehaviors);
Animal henryTheHawk = new Animal(35.5, "Henry the Hawk", henryTheHawkBehaviors);
Animal[] animalList = new Animal[]{zebraZirly, henryTheHawk};
// B
Animal zebraZirly = new Animal("Zirly the Zebra", 812.3, zebraZirlyBehaviors);
Animal henryTheHawk = new Animal("Henry the Hawk", 35.5 , henryTheHawkBehaviors);
Animal[] animalList = new Animal[]{};
animalList.addAnimal(zebraZirly);
animalList.addAnimal(henryTheHawk);
// C
Animal zebraZirly = new Animal("Zirly the Zebra", 812.3, zebraZirlyBehaviors);
Animal henryTheHawk = new Animal("Henry the Hawk", 35.5 , henryTheHawkBehaviors);
double[] animalList = new double[]{zebraZirly, henryTheHawk};
// D
Animal zebraZirly = new Animal("Zirly the Zebra", 812.3, zebraZirlyBehaviors);
Animal henryTheHawk = new Animal("Henry the Hawk", 35.5 , henryTheHawkBehaviors);
Animal[] animalList = new Animal[]{zebraZirly, henryTheHawk};

Step by step
Solved in 2 steps

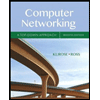
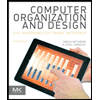
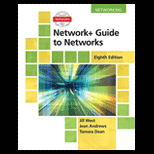
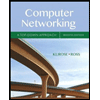
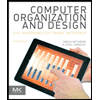
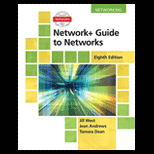
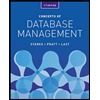
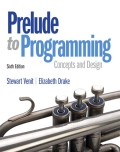
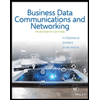