Given this imperative Python snippet, please re-write it to be more functional: people = [{'name': 'Sabah', 'height': 170}, {'name': 'Tim', 'height': 180}, {'name': 'Saly'}] height_total = 0 height_count = 0 for person in people: if 'height' in person: height_total += person['height'] height_count += 1 if height_count > 0: average_height = height_total / height_count print average_height
Given this imperative Python snippet, please re-write it to be more functional:
people = [{'name': 'Sabah', 'height': 170},
{'name': 'Tim', 'height': 180},
{'name': 'Saly'}] height_total = 0
height_count = 0
for person in people:
if 'height' in person:
height_total += person['height'] height_count += 1
if height_count > 0:
average_height = height_total / height_count print average_height

Here's the algorithm for the code that calculates the average height of people with recorded heights using a functional approach:
1. Input:
- Create a list named
people
containing dictionaries, each representing a person with a 'name' and an optional 'height' value.
2. Extract Heights:
- Use a list comprehension to iterate through the
people
list and extract the 'height' values of individuals who have it. - Create a new list named
heights
to store these extracted heights.
3. Calculate Average Height:
- Check if the
heights
list is not empty (i.e., there are recorded heights). - If there are recorded heights:
- Calculate the total height by using the
sum
function to sum all the values in theheights
list. - Calculate the number of recorded heights by using the
len
function on theheights
list. - Compute the average height by dividing the total height by the number of recorded heights.
- Calculate the total height by using the
4. Output:
- If there are recorded heights, print the calculated average height.
5. End of Algorithm.
This algorithm outlines the step-by-step process of extracting heights, calculating the average, and providing the result. It follows a functional programming style to make the code more concise and readable.
Step by step
Solved in 4 steps with 1 images

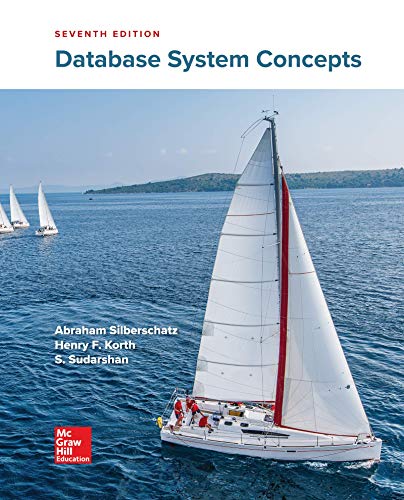
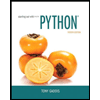
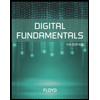
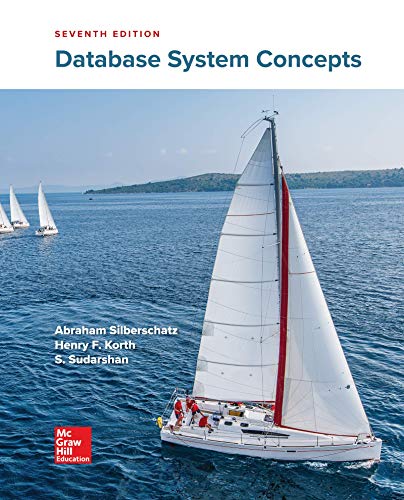
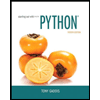
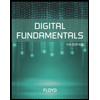
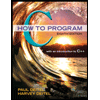
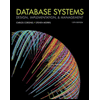
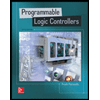