use python Implement the design of the Student and Lawyer class derived from Person class so that the following code generates the output below: class Person: def __init__(self, name, contact): self.name = name self.contact = contact def __str__(self): s = f"Name: {self.name}\nContact: {self.contact}" return s #Write your code here john_cena = Student("John Cena", "9752325", 100005, "CSE") john_cena.add_courses("CSE111 Programming Language II", "CSE230 Discrete Mathematics", "EEE101 Electrical Circuits 1") print("1.=========================================================") print(john_cena) print("2.=========================================================") john_cena.show_course_list("CSE") print("3.=========================================================") john_cena.show_course_list("ECE") print("4.=========================================================") john_cena.add_courses("ARC101 Design I: Basic Design") print("5.=========================================================") print(john_cena) print("6.=========================================================") saul_goodman = Lawyer("Saul Goodman", "5885858", "Dhaka") saul_goodman.add_client("Jesse Pinkman Criminal", "Walter White Criminal", "John Cena Civil", "Naruto Uzumaki Civil") print("7.=========================================================") print(saul_goodman) OUTPUT: 1.========================================================= Student Details: Name: John Cena Contact: 9752325 ID: 100005 Department: CSE Courses List: {'CSE': ['CSE111 Programming Language II', 'CSE230 Discrete Mathematics'], 'EEE': ['EEE101 Electrical Circuits 1']} 2.========================================================= Courses from CSE department: CSE111 Programming Language II CSE230 Discrete Mathematics 3.========================================================= John Cena did not take any course from ECE department 4.========================================================= 5.========================================================= Student Details: Name: John Cena Contact: 9752325 ID: 100005 Department: CSE Courses List: {'CSE': ['CSE111 Programming Language II', 'CSE230 Discrete Mathematics'], 'EEE': ['EEE101 Electrical Circuits 1'], 'ARC': ['ARC101 Design I: Basic Design']} 6.========================================================= 7.========================================================= Lawyer Details: Name: Saul Goodman Contact: 5885858 Area: Dhaka Client List: {'Criminal': ['Jesse Pinkman Criminal', 'Walter White Criminal'], 'Civil': ['John Cena Civil', 'Naruto Uzumaki Civil']}
use python
Implement the design of the Student and Lawyer class derived from Person
class so that the following code generates the output below:
class Person:
def __init__(self, name, contact):
self.name = name
self.contact = contact
def __str__(self):
s = f"Name: {self.name}\nContact: {self.contact}"
return s
#Write your code here
john_cena = Student("John Cena", "9752325", 100005, "CSE")
john_cena.add_courses("CSE111
Mathematics", "EEE101 Electrical Circuits 1")
print("1.=========================================================")
print(john_cena)
print("2.=========================================================")
john_cena.show_course_list("CSE")
print("3.=========================================================")
john_cena.show_course_list("ECE")
print("4.=========================================================")
john_cena.add_courses("ARC101 Design I: Basic Design")
print("5.=========================================================")
print(john_cena)
print("6.=========================================================")
saul_goodman = Lawyer("Saul Goodman", "5885858", "Dhaka")
saul_goodman.add_client("Jesse Pinkman Criminal", "Walter White
Criminal", "John Cena Civil", "Naruto Uzumaki Civil")
print("7.=========================================================")
print(saul_goodman)
OUTPUT:
1.=========================================================
Student Details:
Name: John Cena
Contact: 9752325
ID: 100005
Department: CSE
Courses List:
{'CSE': ['CSE111 Programming Language II', 'CSE230 Discrete
Mathematics'], 'EEE': ['EEE101 Electrical Circuits 1']}
2.=========================================================
Courses from CSE department:
CSE111 Programming Language II
CSE230 Discrete Mathematics
3.=========================================================
John Cena did not take any course from ECE department
4.=========================================================
5.=========================================================
Student Details:
Name: John Cena
Contact: 9752325
ID: 100005
Department: CSE
Courses List:
{'CSE': ['CSE111 Programming Language II', 'CSE230 Discrete
Mathematics'], 'EEE': ['EEE101 Electrical Circuits 1'], 'ARC': ['ARC101
Design I: Basic Design']}
6.=========================================================
7.=========================================================
Lawyer Details:
Name: Saul Goodman
Contact: 5885858
Area: Dhaka
Client List: {'Criminal': ['Jesse Pinkman Criminal', 'Walter White
Criminal'], 'Civil': ['John Cena Civil', 'Naruto Uzumaki Civil']}

Step by step
Solved in 4 steps with 1 images

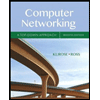
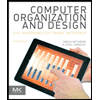
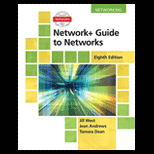
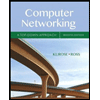
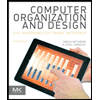
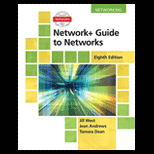
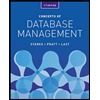
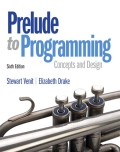
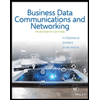