CPS506 Lab 6 Haskell: Type classes and custom types Preamble In this lab you'll create a custom type in Haskell and make it an instance of several built-in Haskell type-classes. Getting Started The provided cabal project defines a module called Lab6. This module will define a custom type called DigitalTime. The DigitalTime type will be used to represent time as a 3-tuple of integers. One for hour, minute, and second, respectively. Thus, the type definition should look like this: data DigitalTime = DigitalTime (Int, Int, Int) Next, you will implement several type classes over this type. These type classes and their operations can be found below. Assume that the hour range is 1-12. Do not use 24h time. Type Classes to Implement Show: show DigitalTime (h, m, s) Print the time in the format "" Eq: (==) (DigitalTime (h1, m1, s1)) (DigitalTime (h2, m2, s2)) Two times are considered equal if they have the same hour, minute, and second values. Ord: (<=) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, s2)) Time are ordered first by hour, then by minute, then by second. Only compare minutes if the hours are the same, and only compare seconds if the minutes are the same. Num: (+) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, s2)) When adding times, first add the seconds. If the seconds overflow (>59), carry the minute. Then add the minutes. If the minutes would overflow (>59), carry the hour. If the hour overflows (>12), it simply wraps around. (-) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, 2)) Just like addition, but this time check for underflow. If subtracting seconds results in a negative number, wrap back around and carry to the minutes, and so on. fromInteger sec Assume that the integer sec is a value in seconds. Convert those seconds into a DigitalTime. For example, 10000 seconds would be two hours, 46 minutes, and 40 seconds, or DigitalTime (2, 46, 40). For the Num type class, you are only required to implement these three operations. Notice that this is not a complete definition, and the compiler will complain with a warning when you load your module into GHCI. This is generally not a good thing, but it's fine for the purposes of this lab. Submission Labs are to be completed and submitted individually. Submit your Lab6.hs source file on D2L under Lab #6.
CPS506 Lab 6
Haskell: Type classes and custom types
Preamble
In this lab you'll create a custom type in Haskell and make it an instance of several built-in Haskell type-classes.
Getting Started
The provided cabal project defines a module called Lab6. This module will define a custom type called DigitalTime. The DigitalTime type will be used to represent time as a 3-tuple of integers. One for hour, minute, and second, respectively. Thus, the type definition should look like this:
data DigitalTime = DigitalTime (Int, Int, Int) Next, you will implement several type classes over this type. These type classes and their operations can be found below. Assume that the hour range is 1-12. Do not use 24h time.
Type Classes to Implement
Show:
show DigitalTime (h, m, s) Print the time in the format "<hh:mm:ss>"
Eq:
(==) (DigitalTime (h1, m1, s1)) (DigitalTime (h2, m2, s2)) Two times are considered equal if they have the same hour, minute, and second values.
Ord:
(<=) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, s2))
Time are ordered first by hour, then by minute, then by second. Only compare minutes if the hours are the same, and only compare seconds if the minutes are the same.
Num:
(+) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, s2))
When adding times, first add the seconds. If the seconds overflow (>59), carry the minute. Then add the minutes. If the minutes would overflow (>59), carry the hour. If the hour overflows (>12), it simply wraps around.
(-) (DigitalTime (h1, ml, s1)) (DigitalTime (h2, m2, 2)) Just like addition, but this time check for underflow. If subtracting seconds results in a negative number, wrap back around and carry to the minutes, and so on.
fromInteger sec
Assume that the integer sec is a value in seconds. Convert those seconds into a DigitalTime. For example, 10000 seconds would be two hours, 46 minutes, and 40 seconds, or DigitalTime (2, 46, 40).
For the Num type class, you are only required to implement these three operations. Notice that this is not a complete definition, and the compiler will complain with a warning when you load your module into GHCI. This is generally not a good thing, but it's fine for the purposes of this lab.
Submission
Labs are to be completed and submitted individually. Submit your Lab6.hs source file on D2L under Lab #6.

Step by step
Solved in 3 steps

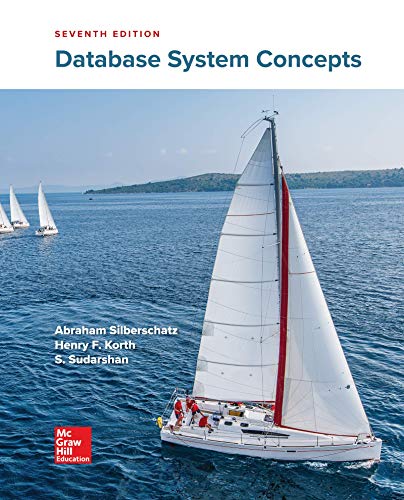
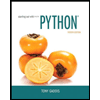
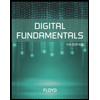
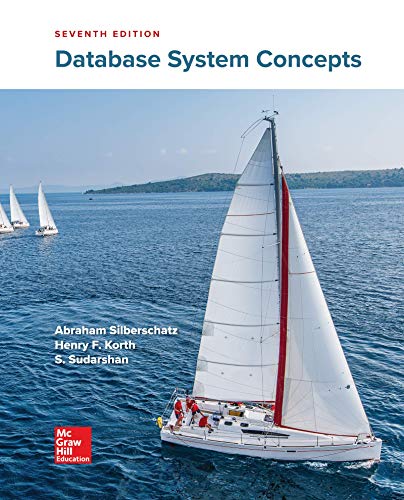
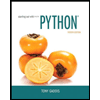
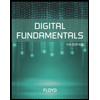
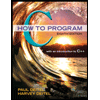
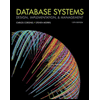
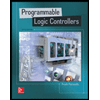