Currently working with class structure type. I want to add an abstract data type, a const, and a class constructor to a code I wrote earlier to help my own undertsanging of the subject material outside of the book. to summarize I am trying to update a C++ code I wrote earlier (perhaps around 30-60 lines of code) and make it compile and run showing how to use: (please label the code added // with abstract data type, const, and class constructor to help me identify it) a. abstract data type b. const c. class constructor #include #include using namespace std; class address // address structure type { public: string streetAddress; string city; string state; int zipcode; }; int main() { address guest; // class address named guest address home; // class adress named home and inputs home.streetAddress = "1234 Right Way Rd."; home.city = "Huntsville"; home.state = "AL"; home.zipcode = 35803; cout << "What is your address? " << endl; // getting inputs for class address named guest cout << "Enter your street address: "; getline(cin, guest.streetAddress); cout << "What city is it located in: "; getline(cin, guest.city); cout << "What state is it located in: "; cin >> guest.state; cout << "What is the zipcode: "; cin >> guest.zipcode; cout << " " << endl; cout << "Your home address is: " << endl; // outputs for guest cout << " " << endl; cout << guest.streetAddress << endl; cout << guest.city << ", " << guest.state << " " << guest.zipcode << endl; cout << " " << endl; cout << "My home address is: " << endl; // outputs for home cout << " " << endl; cout << home.streetAddress << endl; cout << home.city << ", " << home.state << " " << home.zipcode << endl; cout << " " << endl; return 0; }
Currently working with class structure type. I want to add an abstract data type, a const, and a class constructor to a code I wrote earlier to help my own undertsanging of the subject material outside of the book. to summarize I am trying to update a C++ code I wrote earlier (perhaps around 30-60 lines of code) and make it compile and run showing how to use: (please label the code added // with abstract data type, const, and class constructor to help me identify it)
a. abstract data type
b. const
c. class constructor
#include <iostream>
#include <string>
using namespace std;
class address // address structure type
{
public:
string streetAddress;
string city;
string state;
int zipcode;
};
int main()
{
address guest; // class address named guest
address home; // class adress named home and inputs
home.streetAddress = "1234 Right Way Rd.";
home.city = "Huntsville";
home.state = "AL";
home.zipcode = 35803;
cout << "What is your address? " << endl; // getting inputs for class address named guest
cout << "Enter your street address: ";
getline(cin, guest.streetAddress);
cout << "What city is it located in: ";
getline(cin, guest.city);
cout << "What state is it located in: ";
cin >> guest.state;
cout << "What is the zipcode: ";
cin >> guest.zipcode;
cout << " " << endl;
cout << "Your home address is: " << endl; // outputs for guest
cout << " " << endl;
cout << guest.streetAddress << endl;
cout << guest.city << ", " << guest.state << " " << guest.zipcode << endl;
cout << " " << endl;
cout << "My home address is: " << endl; // outputs for home
cout << " " << endl;
cout << home.streetAddress << endl;
cout << home.city << ", " << home.state << " " << home.zipcode << endl;
cout << " " << endl;
return 0;
}

Step by step
Solved in 3 steps with 1 images

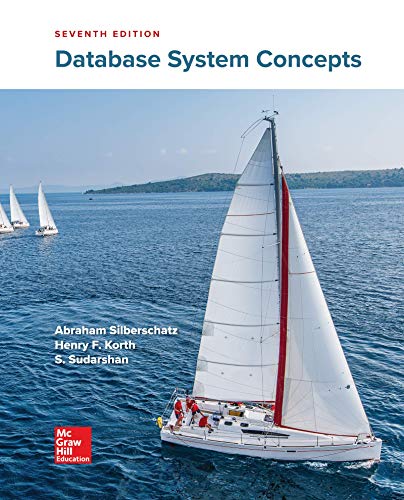
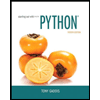
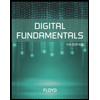
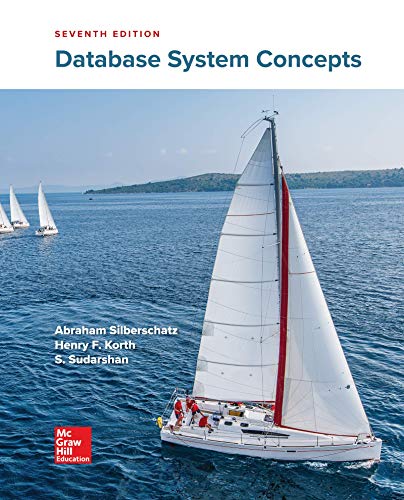
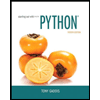
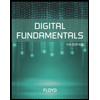
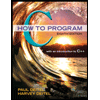
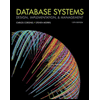
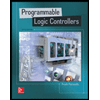