Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments. Ex. If the input is: Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6. 19 the output is: Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19
Given main() and the Instrument class, define a derived class, StringInstrument, for string instruments. Ex. If the input is: Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6. 19 the output is: Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section: Chapter Questions
Problem 1PP
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Hi I would like to know how to write this in c++ please.

Transcribed Image Text:**Programming Challenge: Implementing a String Instrument Class**
**Objective:**
Given the main() function and the base class `Instrument`, your task is to define a derived class, `StringInstrument`, specifically for string instruments.
### Example Input:
```
Drums
Zildjian
2015
2500
Guitar
Gibson
2002
1200
6
19
```
### Expected Output:
```
Instrument Information:
Name: Drums
Manufacturer: Zildjian
Year built: 2015
Cost: 2500
Instrument Information:
Name: Guitar
Manufacturer: Gibson
Year built: 2002
Cost: 1200
Number of strings: 6
Number of frets: 19
```
### Code Snippet:
Here is a snippet of the `StringInstrument.cpp` file that outlines the basic structure. You need to implement the necessary functions to handle string-specific attributes.
```cpp
#include "StringInstrument.h"
// TODO: Define mutator functions -
// SetNumOfStrings(), SetNumOfFrets()
// TODO: Define accessor functions -
// GetNumOfStrings(), GetNumOfFrets()
```
### Instructions:
1. **Create a Derived Class:**
- The class `StringInstrument` should inherit from the `Instrument` class.
2. **Implement Mutator Functions:**
- `SetNumOfStrings()`: Sets the number of strings for the instrument.
- `SetNumOfFrets()`: Sets the number of frets.
3. **Implement Accessor Functions:**
- `GetNumOfStrings()`: Returns the number of strings.
- `GetNumOfFrets()`: Returns the number of frets.
### Diagram Explanation:
There are no specific graphs or diagrams present in the image. The key focus is on implementing the class structure within the provided code framework.
Keep this structure in mind while integrating the functionalities, ensuring your class captures all relevant data for string instruments.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
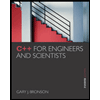
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
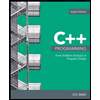
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
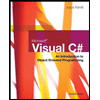
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
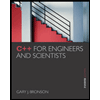
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
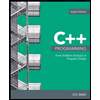
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
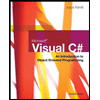
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
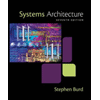
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
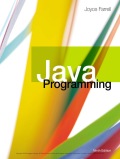
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage