Need help implenting the classes below in Python 3 Please take a close look at the UML diagram as well as the requirements of the classes.
Need help implenting the classes below in Python 3 Please take a close look at the UML diagram as well as the requirements of the classes.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help implenting the classes below in Python 3
Please take a close look at the UML diagram as well as the requirements of the classes.

Transcribed Image Text:The diagram depicts a class model for a university system with several key components: University, Student, Course, UnderGrad, and Graduate. Below is a detailed explanation of each class and its elements:
### Class: University
- **Attributes:**
- `name: string`
- **Methods:**
- `__str__`: String representation of the university.
- `add_undergrad(name, email, gpa)`: Adds an undergraduate student.
- `add_graduate(name, email, level)`: Adds a graduate student.
- `add_course(...)`: Adds a course.
- `remove_student(name) : list`: Removes a student by name.
- `get_students(name, id, email) : list`: Retrieves a list of students by name, id, or email.
- `get_courses(subject, number, title) : list`: Retrieves a list of courses by subject, number, or title.
- `enroll_student(id, subject, number)`: Enrolls a student in a course.
- `enrollment_report() : dict`: Generates an enrollment report.
- **Relationships:**
- Has a one-to-many relationship with `Course`.
- Has a one-to-many relationship with `Student`.
### Class: Student
- **Attributes:**
- `name: string`
- `id: string`
- `email: string`
- **Methods:**
- `reset_id_numbering()`: Resets the student ID numbering.
- `get_standing() : string`: Returns the academic standing of the student.
- `enroll_to(Course)`: Enrolls the student in a course.
- `__str__`: String representation of the student.
- **Relationships:**
- Inherits from both `UnderGrad` and `Graduate`.
### Class: Course
- **Attributes:**
- `subject: string`
- `number: int`
- `title: string`
- **Methods:**
- `__str__`: String representation of the course.
- **Relationships:**
- Course is associated with the University and can have multiple students enrolled.
### Class: UnderGrad
- **Attributes:**
- `gpa: float`
- **Methods:**
- `get_standing() : string`: Returns the academic standing based on GPA.
- `__str__`:

Transcribed Image Text:- **Instance Attributes and Properties**
- Protect the data to ensure encapsulation.
- Implement properties for all instance attributes unless noted.
- **Student**
- Student is an abstract class that represents general students. *Implement it as guided on exercise 10.26 on page 428 in the textbook*.
- Properties are read-only.
- email data should be validated on the form of "<any text>@ucdenver.edu", and should be all lowercase.
- Add the constructor to initialize all data.
- When a Student user is created, the id should be generated automatically in sequence. The first student id should be "000001" and increment by 1 once a new student object is created.
- The `reset_id_numbering` class method should reset the numbering of the ids, restarting to 1.
- Students can be enrolled to one or more courses, or not enrolled at all. `enroll_to` receives a course object and add it to the enrolled_to list.
- **UnderGrad:**
- Undergraduate students.
- gpa should be between 0 and 4
- Standing is "Undergraduate"
- Add constructor to initialize all data properly
- **Graduate:**
- Graduate (master/phd) students
- Standing is "Master" or "PhD" depending on the current level.
- current level represents either "master" or "phd"
- Add constructor to initialize all data properly
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
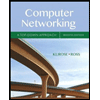
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
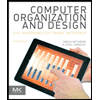
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
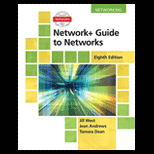
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
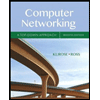
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
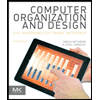
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
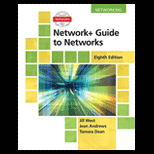
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
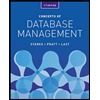
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
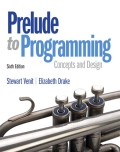
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
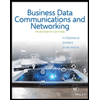
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY