Given the following code, explain why the counter resulted in 0 when the program was asked to arrange the array in ascending order. import java.util.*; public class BubbleSort { static int bubbleSort(int[] arr) { int n = arr.length; int temp = 0, count = 0; for (int i = 0; i < n; i++) { for (int j = 1; j < (n - i); j++) { if (arr[j - 1] > arr[j]) { count++; temp = arr[j - 1]; arr[j - 1] = arr[j]; arr[j] = temp; } } } return count; } public static void main(String[] args) { int n = 10000; int max = 10000, min = 1; int[] data = new int[n]; int[] data1 = new int[n]; // Generating 10000 numbers in the range 1-10000 for (int i = 0; i < n; i++) { data[i] = (int)Math.floor(Math.random() * (max - min + 1) + min); data1[i] = data[i]; } // To get data in ascending order Arrays.sort(data1); int count = bubbleSort(data1); System.out.println("The number of statements executed in the process of sorting the data arranged in ascending order: " + count); count = bubbleSort(data); System.out.println("The number of statements executed in the process of sorting the randomly arranged data: " + count); // To get data in descending order I am adding - in front of every number in array data1 // which is in ascending order for (int i = 0; i < n; i++) { data1[i] = -data1[i]; } count = bubbleSort(data1); System.out.println("The number of statements executed in the process of sorting the data arranged in descending order: " + count
Given the following code, explain why the counter resulted in 0 when the program was asked to arrange the array in ascending order.
import java.util.*;
public class BubbleSort
{
static int bubbleSort(int[] arr)
{
int n = arr.length;
int temp = 0, count = 0;
for (int i = 0; i < n; i++)
{
for (int j = 1; j < (n - i); j++)
{
if (arr[j - 1] > arr[j])
{
count++;
temp = arr[j - 1];
arr[j - 1] = arr[j];
arr[j] = temp;
}
}
}
return count;
}
public static void main(String[] args)
{
int n = 10000;
int max = 10000, min = 1;
int[] data = new int[n];
int[] data1 = new int[n];
// Generating 10000 numbers in the range 1-10000
for (int i = 0; i < n; i++)
{
data[i] = (int)Math.floor(Math.random() * (max - min + 1) + min);
data1[i] = data[i];
}
// To get data in ascending order
Arrays.sort(data1);
int count = bubbleSort(data1);
System.out.println("The number of statements executed in the process of sorting the data arranged in ascending order: " + count);
count = bubbleSort(data);
System.out.println("The number of statements executed in the process of sorting the randomly arranged data: " + count);
// To get data in descending order I am adding - in front of every number in array data1
// which is in ascending order
for (int i = 0; i < n; i++)
{
data1[i] = -data1[i];
}
count = bubbleSort(data1);
System.out.println("The number of statements executed in the process of sorting the data arranged in descending order: " + count);
}
}


Step by step
Solved in 2 steps with 1 images

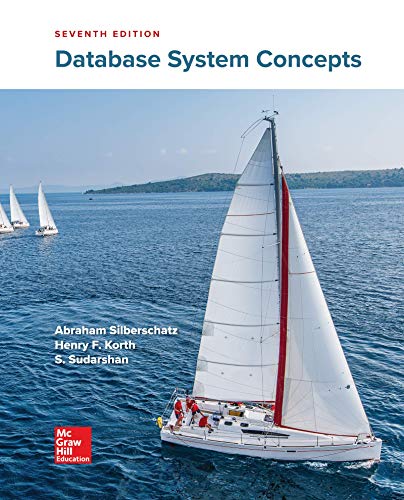
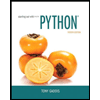
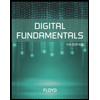
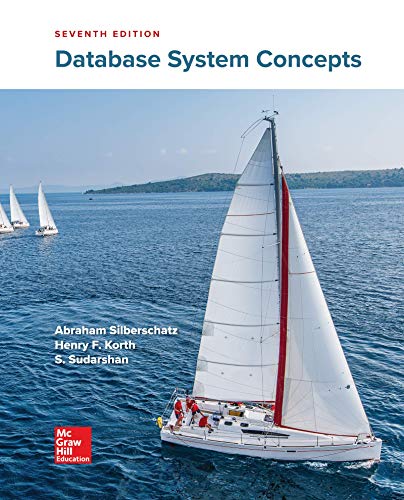
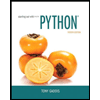
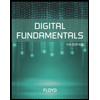
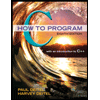
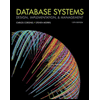
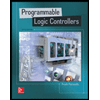