JAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into its words while (words.hasMoreTokens()) { // For each word in s result.append(encode(words.nextToken()) + " "); // Encode it } return result.toString(); // Return the result } // encrypt() public String decrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into words while (words.hasMoreTokens()) { // For each word in s result.append(decode(words.nextToken()) + " "); // Decode it } return result.toString(); // Return the decryption } // decrypt() public abstract String encode(String word); // Abstract methods public abstract String decode(String word); } // Cipher class Transpose extends Cipher { // encode() reverses and returns a word // Modify this to use rotation instead of reverse public String encode(String word) { StringBuffer result = new StringBuffer(word); return result.reverse().toString(); } // encode() public String decode(String word) { return encode(word); // Just call encode } // decode } // Transpose
JAVA Language:
Transpose Rotate.
Question:
import java.util.*;
public class TestTranspose {
public static void main(String[] args) {
String plain = "this is the secret message"; // Here's the message
Transpose transpose = new Transpose();
String secret = transpose.encrypt(plain);
System.out.println("\n ********* Transpose Cipher Encryption *********");
System.out.println("PlainText: " + plain); // Display the results
System.out.println("Encrypted: " + secret);
System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt
}
}
abstract class Cipher {
public String encrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into its words
while (words.hasMoreTokens()) { // For each word in s
result.append(encode(words.nextToken()) + " "); // Encode it
}
return result.toString(); // Return the result
} // encrypt()
public String decrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into words
while (words.hasMoreTokens()) { // For each word in s
result.append(decode(words.nextToken()) + " "); // Decode it
}
return result.toString(); // Return the decryption
} // decrypt()
public abstract String encode(String word); // Abstract methods
public abstract String decode(String word);
} // Cipher
class Transpose extends Cipher {
// encode() reverses and returns a word
// Modify this to use rotation instead of reverse
public String encode(String word) {
StringBuffer result = new StringBuffer(word);
return result.reverse().toString();
} // encode()
public String decode(String word) {
return encode(word); // Just call encode
} // decode
} // Transpose

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

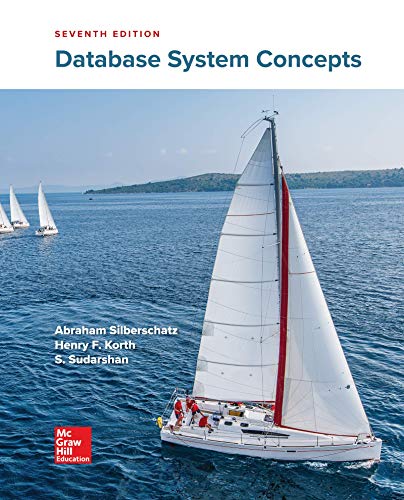
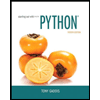
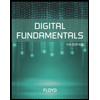
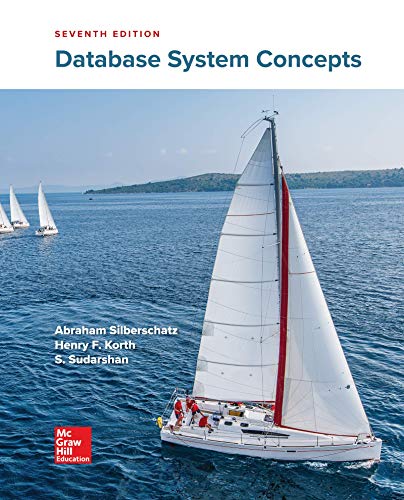
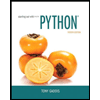
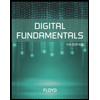
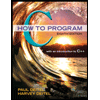
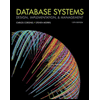
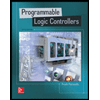