Complete the following method in java that takes an array and a key(int value) that traverses the input parameter array with a nested for loop and finds the number of pairs of the numbers in the array that add up to the val(key) parameter. I'm not sure if this is correct: public static int nestedLoopsTwoSum(int[] X, int val) { int count = 0; for (int i = 0; i < X.length; i++) { int k; for (k = 0; k < i ; k++) { if ((X[i] + X[k]) == val) { count++; } } } return count; }
Complete the following method in java that takes an array and a key(int value) that traverses the input parameter array with a nested for loop and finds the number of pairs of the numbers in the array that add up to the val(key) parameter.
I'm not sure if this is correct:
public static int nestedLoopsTwoSum(int[] X, int val) {
int count = 0;
for (int i = 0; i < X.length; i++)
{
int k;
for (k = 0; k < i ; k++)
{
if ((X[i] + X[k]) == val)
{
count++;
}
}
}
return count;
}

//we have to find count of all pairs whose sum is passed value
//to find count of all such pairs, we have to generate all possible pairs
//first lets check whether your code, generates all possible pairs or not
//lets analyse your code:
public static int nestedLoopsTwoSum(int[] X, int val) {
int count = 0;
for (int i = 0; i < X.length; i++)//runs for each element of the X
{
int k;
for (k = 0; k < i ; k++)// for each element from index :0 to i-1
{
//each ith element is combined with all elements below i
//that means your code will generate all possible pairs
if ((X[i] + X[k]) == val)// then it checks whether, sum of pair equals val
{
count++;//if equal then increments count
}
}
}
return count;// finally returns count
}
//means it correctly returns the count of all pairs whose sum is val
Step by step
Solved in 2 steps

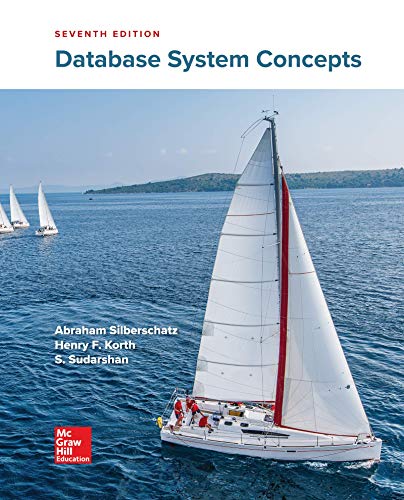
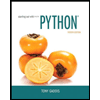
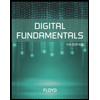
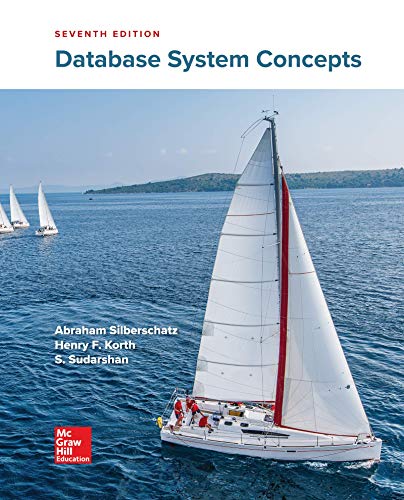
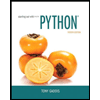
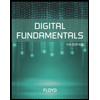
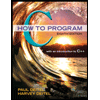
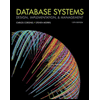
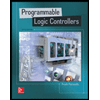