Give DETAILED EXPLANATION about how this code works. Also, show output of the program while pointing out what it does. (IF CAN MAKE THE PROGRAM BETTER, I WILL RATE IT ASAP.) Thanks in advance.
Give DETAILED EXPLANATION about how this code works. Also, show output of the program while pointing out what it does. (IF CAN MAKE THE PROGRAM BETTER, I WILL RATE IT ASAP.) Thanks in advance.
#include "tchar.h"
#include <stdio.h>
#include <conio.h>
#include <string.h>
#include <stdlib.h>
#include <iostream>
using namespace std;
struct allocList
{
char* startAlloc;
char* endAlloc;
int fk_pid;
allocList* nxt;
};
struct procList
{
int pid;
int jobStatus;
char* startProc;
char* endProc;
procList* nxt;
};
bool initProc(procList*&, int*, int);
bool initAlloc(allocList*&, int*, int);
void doFirstFit(int arrMemory[], int arrJobs[], int m, int n);
void cmptFragmentation(procList*, allocList*);
int search(procList*, int);
int _tmain(int argc, _TCHAR* argv[])
{
int arrMemory[] = { 100, 500, 200, 300, 600 };
int arrJobs[] = { 212, 17, 112, 426, 500 };
int m = (sizeof(arrMemory) / sizeof(arrMemory[0]));
int n = (sizeof(arrJobs) / sizeof(arrJobs[0]));
allocList* ptrAllocStart = NULL;
procList* ptrProcStart = NULL;
initProc(ptrProcStart, arrJobs, (sizeof(arrJobs) / sizeof(int)));
initAlloc(ptrAllocStart, arrMemory, (sizeof(arrMemory) / sizeof(int)));
cout << "Memory Block:" << endl << "Block\tSpace" << endl;
int i;
for (i = 0; i < sizeof(arrMemory) / sizeof(int); i++)
cout << i + 1 << "\t" << arrMemory[i] << endl;
cout << "\nJobs:" << endl << "Job\tSize" << endl;
for (i = 0; i < sizeof(arrJobs) / sizeof(int); i++)
cout << i + 1 << "\t" << arrJobs[i] << endl;
doFirstFit(arrMemory, arrJobs, m, n);
cmptFragmentation(ptrProcStart, ptrAllocStart);
system("pause");
return 0;
}
void doFirstFit(int arrMemory[], int arrJobs[], int m, int n)
{
int allocation[5];
int internalFrag[5] = { 0 };
memset(allocation, -1, sizeof(allocation));
for (int i = 0; i < n; i++)
{
for (int j = 0; j < m; j++)
{
if (arrMemory[j] >= arrJobs[i])
{
allocation[i] = j + 1;
internalFrag[i] = arrMemory[j] - arrJobs[i];
arrMemory[j] -= arrJobs[i];
break;
}
}
}
cout << "\n\nFirst Fit:\nMemory Block\tSize\tJob\tInternal " << "Fragmentation\n";
for (int i = 0; i < n; i++)
{
if (allocation[i] != -1)
cout << "\t" << allocation[i];
else
cout << "\t" << "NA";
cout << "\t" << arrJobs[i] << "\t" << i + 1 << "\t";
if (allocation[i] != -1)
cout << internalFrag[i] << endl;
else
cout << "NA";
}
}
bool initProc(procList*& ptrProcStart, int* ptrArrProc, int length)
{
int i;
procList* ptrProc = ptrProcStart;
for (i = 0; i < length; i++)
{
if (ptrProc != NULL)
{
ptrProc->nxt = new procList;
ptrProc = ptrProc->nxt;
ptrProc->startProc = (char*)malloc(*(ptrArrProc + i));
ptrProc->endProc = ptrProc->startProc + *(ptrArrProc + i);
memset(ptrProc->startProc, 'a' + i, *(ptrArrProc + i));
ptrProc->jobStatus = 0;
ptrProc->pid = i;
ptrProc->nxt = NULL;
}
else
{
ptrProc = new procList;
ptrProc->startProc = (char*)malloc(*(ptrArrProc + i));
ptrProc->endProc = ptrProc->startProc + *(ptrArrProc + i);
memset(ptrProc->startProc, 'a' + i, *(ptrArrProc + i));
ptrProc->jobStatus = 0;
ptrProc->pid = i;
ptrProc->nxt = NULL;
ptrProcStart = ptrProc;
}
}
return true;
}
bool initAlloc(allocList*& ptrAllocStart, int* ptrArrAlloc, int length)
{
int i;
allocList* ptrAlloc = ptrAllocStart;
for (i = 0; i < length; i++)
{
if (ptrAlloc != NULL)
{
ptrAlloc->nxt = new allocList;
ptrAlloc = ptrAlloc->nxt;
ptrAlloc->startAlloc = (char*)malloc(*(ptrArrAlloc + i));
ptrAlloc->endAlloc = ptrAlloc->startAlloc + *(ptrArrAlloc + i);
memset(ptrAlloc->startAlloc, 'a' + i, *(ptrArrAlloc + i));
ptrAlloc->fk_pid = i;
ptrAlloc->nxt = NULL;
}
else
{
ptrAlloc = new allocList;
ptrAlloc->startAlloc = (char*)malloc(*(ptrArrAlloc + i));
ptrAlloc->endAlloc = ptrAlloc->startAlloc + *(ptrArrAlloc + i);
memset(ptrAlloc->startAlloc, 'a' + i, *(ptrArrAlloc + i));
ptrAlloc->fk_pid = i;
ptrAlloc->nxt = NULL;
ptrAllocStart = ptrAlloc;
}
}
return true;
}
void cmptFragmentation(procList* proc, allocList* alloc)
{
allocList* memory;
memory = alloc;
cout << endl << endl << "Defragmentation\nMemory " << "Block\tSize\tJob\tFreeSpace\n";
int i = 0;
while (memory != NULL)
{
i++;
cout << "\t" << i << "\t" << memory->endAlloc - memory->startAlloc << "\t" << memory->fk_pid + 1 << "\t"
<< memory->endAlloc - memory->startAlloc - search(proc, memory->fk_pid - 1) << endl;
memory = memory->nxt;
}
}
int search(procList* job, int id)
{
int size = 0;
while (job != NULL)
{
if (job->pid == id)
{
size = (int)job->endProc - (int)job->startProc;
break;
}
job = job->nxt;
}
return size;
}

Step by step
Solved in 4 steps with 3 images

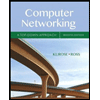
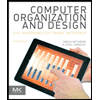
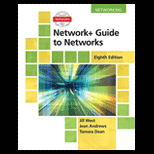
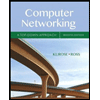
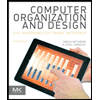
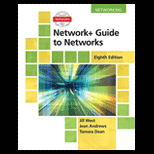
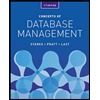
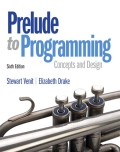
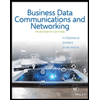