GameState represents the state of the game "world." The GameState object tracks all of the moving entities like tanks * and shells, and provides the controller of the program (i.e. the GameDriver) access to whatever information it needs * to run the game. Essentially, GameState is the "data context" needed for the rest of the program. */
PART 1* I need help implementing some functions for a tank game coded in java. They are labeled under //TODO. Below are the first 3 files. I will post another question for the remaining 5 files labeled PART 2. Thank you!
/**
* GameState represents the state of the game "world." The GameState object tracks all of the moving entities like tanks
* and shells, and provides the controller of the program (i.e. the GameDriver) access to whatever information it needs
* to run the game. Essentially, GameState is the "data context" needed for the rest of the program.
*/
public class GameState {
public static final double TANK_X_LOWER_BOUND = 30.0;
public static final double TANK_X_UPPER_BOUND = RunGameView.SCREEN_DIMENSIONS.width - 100.0;
public static final double TANK_Y_LOWER_BOUND = 30.0;
public static final double TANK_Y_UPPER_BOUND = RunGameView.SCREEN_DIMENSIONS.height - 120.0;
public static final double SHELL_X_LOWER_BOUND = -10.0;
public static final double SHELL_X_UPPER_BOUND = RunGameView.SCREEN_DIMENSIONS.width;
public static final double SHELL_Y_LOWER_BOUND = -10.0;
public static final double SHELL_Y_UPPER_BOUND = RunGameView.SCREEN_DIMENSIONS.height;
public static final String PLAYER_TANK_ID = "player-tank";
public static final String AI_TANK_ID = "
// TODO: Feel free to add more tank IDs if you want to support multiple AI tanks! Just make sure they're unique.
// TODO: Implement.
// There's a lot of information the GameState will need to store to provide contextual information. Add whatever
// instance variables, constructors, and methods are needed.
}
/**
* Model class representing a shell that has been fired by a tank. A shell has a position and an angle, as well as a
* speed. Shells by default should be unable to turn and only move forward.
*/
// TODO: Notice that Shell has a lot in common with Tank. For full credit, you will need to find a way to share code
// between the two classes so that the logic for e.g. moveForward, etc. are not duplicated.
public class Shell {
private static final String SHELL_ID_PREFIX = "shell-";
private static final double MOVEMENT_SPEED = 4.0;
private static long uniqueId = 0L;
private final String id;
private double x;
private double y;
private double angle;
public Shell(double x, double y, double angle) {
this.id = getUniqueId();
this.x = x;
this.y = y;
this.angle = angle;
}
private String getUniqueId() {
return SHELL_ID_PREFIX + uniqueId++;
}
}
/**
* Model class representing a tank in the game. A tank has a position and an angle. It has a movement speed and a turn
* speed, both represented below as constants.
*/
// TODO: Notice that Tank has a lot in common with Shell. For full credit, you will need to find a way to share code
// between the two classes so that the logic for e.g. moveForward, etc. are not duplicated.
public class Tank {
private static final double MOVEMENT_SPEED = 2.0;
private static final double TURN_SPEED = Math.toRadians(3.0);
private final String id;
private double x;
private double y;
private double angle;
public Tank(String id, double x, double y, double angle) {
this.id = id;
this.x = x;
this.y = y;
this.angle = angle;
}
public String getId() {
return id;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double getAngle() {
return angle;
}
// TODO: The methods below are provided so you don't have to do the math for movement. However, note that they are
// protected. You should not be calling these methods directly from outside the Tank class hierarchy. Instead,
// consider how to design your Tank class(es) so that a Tank can represent both a player-controlled tank and an AI
// controlled tank.
protected void moveForward() {
x += MOVEMENT_SPEED * Math.cos(angle);
y += MOVEMENT_SPEED * Math.sin(angle);
}
protected void moveBackward() {
x -= MOVEMENT_SPEED * Math.cos(angle);
y -= MOVEMENT_SPEED * Math.sin(angle);
}
protected void turnLeft() {
angle -= TURN_SPEED;
}
protected void turnRight() {
angle += TURN_SPEED;
}
// The following methods will be useful for determining where a shell should be spawned when it
// is created by this tank. It needs a slight offset so it appears from the front of the tank,
// even if the tank is rotated. The shell should have the same angle as the tank.
private double getShellX() {
return getX() + 30.0 * (Math.cos(getAngle()) + 0.5);
}
private double getShellY() {
return getY() + 30.0 * (Math.sin(getAngle()) + 0.5);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

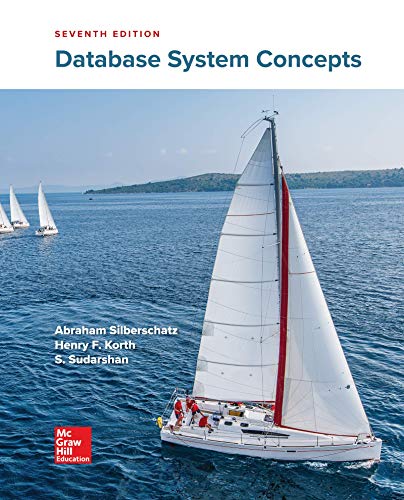
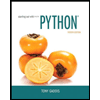
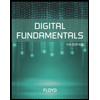
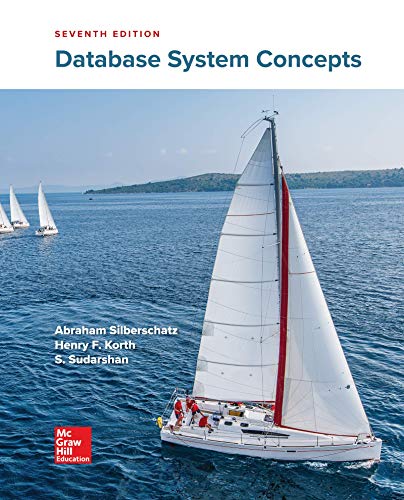
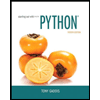
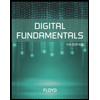
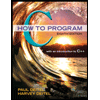
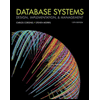
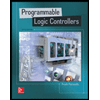