From two dictionary objects created in the codes below, find letters that only exist in one of the dictionaries. Use a SET object(s) and its mathematical operator(s). import string # import string library import random # import random library # a_base is a string containing concatenation of letters s_base = string.ascii_letters # random.seed() accepts any integer to initialize random numbers random.seed(582) # s is a string variable s = "" # this line creates a loop starting from 0 to 199 for i in range(200): # this line randomly selects letters from s_base variable and concatenates them to the value stored in s s += s_base[random.randint(0, 25)] # this line prints the value of s print(s) # count each char frequency count = {} for ch in s: if ch in count: count[ch] = count[ch]+1 else: count[ch] = 1 # print the frequency for key in count: print("{} -> {}".format(key, count[key])) def char_frequency(s): dict = {} for d in s: keys = dict.keys() if d in keys: dict[d] += 1 else: dict[d] = 1 return dict counts = char_frequency(s) print(char_frequency(s)) Subject: Python Programming
From two dictionary objects created in the codes below, find letters that only exist in one of the dictionaries. Use a SET object(s) and its mathematical operator(s).
import string # import string library
import random # import random library
# a_base is a string containing concatenation of letters
s_base = string.ascii_letters
# random.seed() accepts any integer to initialize random numbers
random.seed(582)
# s is a string variable
s = ""
# this line creates a loop starting from 0 to 199
for i in range(200):
# this line randomly selects letters from s_base variable and concatenates them to the value stored in s
s += s_base[random.randint(0, 25)]
# this line prints the value of s
print(s)
# count each char frequency
count = {}
for ch in s:
if ch in count:
count[ch] = count[ch]+1
else:
count[ch] = 1
# print the frequency
for key in count:
print("{} -> {}".format(key, count[key]))
def char_frequency(s):
dict = {}
for d in s:
keys = dict.keys()
if d in keys:
dict[d] += 1
else:
dict[d] = 1
return dict
counts = char_frequency(s)
print(char_frequency(s))
Subject: Python

Step by step
Solved in 2 steps with 1 images

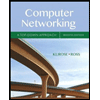
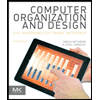
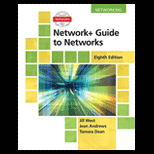
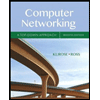
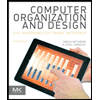
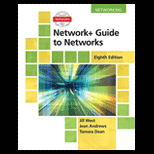
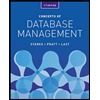
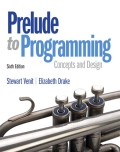
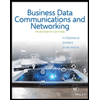