For the TimeSpan class described below, add a compareTo method that takes another TimeSpan object as a parameter & returns: -1 if this TimeSpan is SMALLER than to the TimeSpan object passed in as a parameter 0 if both TimeSpans are the same 1 if this TimeSpan is GREATER than the TimeSpan object passed in as a parameter Add code, as needed, to allow the Collections class to sort the ArrayList of TimeSpan objects import java.util.*; public class TimeSpan implements { private int hours; private int minutes;
. For the TimeSpan class described below, add a compareTo method that takes another TimeSpan object as a parameter & returns:
-1 if this TimeSpan is SMALLER than to the TimeSpan object passed in as a parameter
0 if both TimeSpans are the same
1 if this TimeSpan is GREATER than the TimeSpan object passed in as a parameter
Add code, as needed, to allow the Collections class to sort the ArrayList of TimeSpan objects
import java.util.*;
public class TimeSpan implements {
private int hours;
private int minutes;
public TimeSpan(int hours1, int minutes1) {
hours = 0;
minutes = 0;
add(hours1, minutes1);
}
public void add(int hours1, int minutes1) {
hours += hours1;
minutes += minutes1;
while (minutes > 60) {
minutes -= 60; // convert 60 min --> 1 hour
hours++;
}
}
public void add(TimeSpan time) {
add(time.hours, time.minutes);
}
public double getTotalHours() {
return hours + minutes / 60.0;
}
public String toString() {
return hours + "h-" + minutes + "m";
}
// *** Your method code here ***
public static void main(String[] args) {
TimeSpan t1 = new TimeSpan(4, 13);
TimeSpan t2 = new TimeSpan(5, 8);
TimeSpan t3 = new TimeSpan(3, 51);
TimeSpan t4 = new TimeSpan(6, 76);
TimeSpan t5 = new TimeSpan(1, 1);
ArrayList<TimeSpan> list = new ArrayList<TimeSpan>();
list.add(t1);
list.add(t2);
list.add(t3);
list.add(t4);
list.add(t5);
System.out.println(list);
Collections.sort(list);
System.out.println(list);
}
}
Expected Output: > run TimeSpan
[4h-13m, 5h-8m, 3h-51m, 7h-16m, 1h-1m]
[1h-1m, 3h-51m, 4h-13m, 5h-8m, 7h-16m]

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

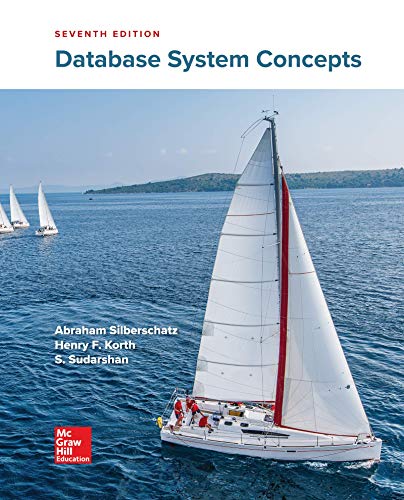
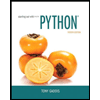
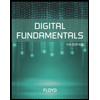
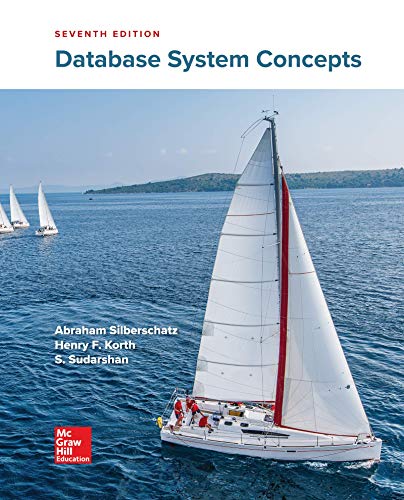
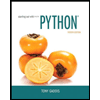
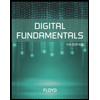
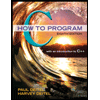
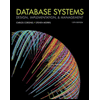
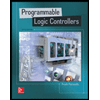